


Convert an array to a list using the asList() method of the Arrays class in Java
Use the asList() method of the Arrays class in Java to convert an array into a list
In Java programming, you often encounter the need to convert an array into a list. Java provides an asList() method of the Arrays class, which can easily convert arrays into lists. This article explains how to use the asList() method and provides code examples to demonstrate it.
First, let us understand the definition and function of the asList() method. The asList() method is a static method of the Arrays class, which is defined as follows:
public static <T> List<T> asList(T... a)
It accepts a variable-length parameter and converts it into a list object. We can pass an array of any type to the asList() method and it will return a list of the corresponding type. This way, we can operate on arrays just like lists.
The following is a simple example that demonstrates how to convert an integer array into a list and operate on it:
import java.util.Arrays; import java.util.List; public class ArrayToListExample { public static void main(String[] args) { // 定义一个整型数组 Integer[] array = {1, 2, 3, 4, 5}; // 将数组转换为列表 List<Integer> list = Arrays.asList(array); // 输出列表中的元素 for (Integer num : list) { System.out.println(num); } // 修改列表中的元素 list.set(0, 10); // 输出修改后的数组 System.out.println(Arrays.toString(array)); } }
The above code first defines an integer array and assigns it to {1, 2, 3, 4, 5}. Then, convert the array to a list through the Arrays.asList() method and assign the returned list to the list variable. Next, use an enhanced for loop to iterate through the list and output each element in it. Finally, modify the first element in the list to 10 by calling the list.set() method, and output the modified array through the Arrays.toString() method.
Run the above example code, the following results will be output:
1 2 3 4 5 [10, 2, 3, 4, 5]
As you can see from the output results, after converting the array into a list, we can use the list to operate the array. When we modify an element in the list, the corresponding array is also modified.
It should be noted that the asList() method returns a fixed-size list, which means that we cannot add or delete elements to the list. If we try to do this, an UnsupportedOperationException will be thrown.
If we want to get a list that can be added or deleted, we can achieve this by constructing an ArrayList object. The following is a sample code:
import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class ArrayToListExample { public static void main(String[] args) { // 定义一个整型数组 Integer[] array = {1, 2, 3, 4, 5}; // 将数组转换为列表 List<Integer> list = new ArrayList<>(Arrays.asList(array)); // 输出列表中的元素 for (Integer num : list) { System.out.println(num); } // 添加元素到列表 list.add(6); // 输出修改后的列表 System.out.println(list); } }
The above code uses the ArrayList constructor and takes the list returned by the Arrays.asList() method as a parameter when converting the array to a list. In this way, we get a list that can be added or deleted.
In this article, we introduced how to convert an array into a list using the asList() method of the Arrays class. In this way, we can easily operate arrays in Java programming just like operating lists. Whether it is simply traversing the elements of the list or modifying an element in the list, we can easily achieve it through the asList() method. However, it should be noted that the asList() method returns a fixed-size list and does not support adding or deleting elements. If you need to modify the list, you can do so by constructing an ArrayList object. I hope this article helped you understand how arrays are converted to lists in Java.
The above is the detailed content of Convert an array to a list using the asList() method of the Arrays class in Java. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
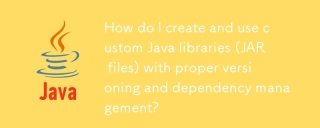
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
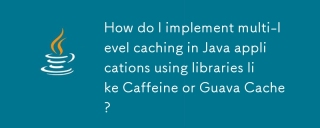
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
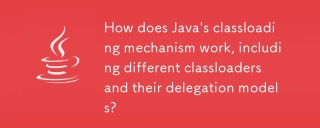
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool