php editor Xinyi introduces to you a method to improve code maintainability: adopt PHP design pattern. Design patterns are a set of summarized design experiences that are used repeatedly and are known to most people. Design patterns can be used to effectively solve code maintainability problems. By rationally applying design patterns, the code can be made clearer, easier to understand and maintain, and the quality and maintainability of the code can be improved. This is a skill that every PHP developer should learn and master.
The singleton pattern ensures that a class has only one instance. This is useful for classes that require global access, such as a database connection or a configuration manager. The following is the PHP implementation of the singleton pattern:
class Database
{
private static $instance = null;
private function __construct() {}
public static function getInstance()
{
if (self::$instance === null) {
self::$instance = new Database();
}
return self::$instance;
}
}
The Observer pattern allows objects (called observers) to subscribe to events or state changes (called topics). When the topic state changes, it notifies all observers. This is a great way to communicate and decouple components.
interface Observer { public function update($message); } class ConcreteObserver implements Observer { public function update($message) { echo "Received message: $message" . php_EOL; } } class Subject { private $observers = []; public function addObserver(Observer $observer) { $this->observers[] = $observer; } public function notifyObservers($message) { foreach ($this->observers as $observer) { $observer->update($message); } } }Strategy Mode
The Strategy pattern allows you to define a set of
algorithms or behaviors in a class and select and change them at runtime. This provides a high degree of flexibility while keeping the code easy to maintain.
interface SortStrategy
{
public function sort($data);
}
class BubbleSortStrategy implements SortStrategy
{
public function sort($data)
{
// Implement bubble sort alGorithm
}
}
class QuickSortStrategy implements SortStrategy
{
public function sort($data)
{
// Implement quick sort algorithm
}
}
class SortManager
{
private $strategy;
public function setStrategy(SortStrategy $strategy)
{
$this->strategy = $strategy;
}
public function sortData($data)
{
$this->strategy->sort($data);
}
}
The factory method pattern defines an interface for creating objects, letting subclasses decide which type of object is actually created. This allows you to create different types of objects without changing client code.
interface Creator { public function createProduct(); } class ConcreteCreatorA implements Creator { public function createProduct() { return new ProductA(); } } class ConcreteCreatorB implements Creator { public function createProduct() { return new ProductB(); } } class Client { private $creator; public function setCreator(Creator $creator) { $this->creator = $creator; } public function createProduct() { return $this->creator->createProduct(); } }Decorator Pattern
The decorator pattern dynamically extends the functionality of a class without modifying its source code. It creates a class that wraps the original class and adds new behavior to it.
interface Shape { public function draw(); } class Circle implements Shape { public function draw() { echo "Drawing a circle." . PHP_EOL; } } class Decorator implements Shape { private $component; public function __construct(Shape $component) { $this->component = $component; } public function draw() { $this->component->draw(); } } class RedDecorator extends Decorator { public function __construct(Shape $component) { parent::__construct($component); } public function draw() { parent::draw(); echo "Adding red color to the shape." . PHP_EOL; } }in conclusion
PHP
Design patternprovides powerful tools to improve code maintainability, reusability and scalability. By adopting these design patterns, you can write code that is more flexible, easier to understand, and maintain, saving time and effort in the long run.
The above is the detailed content of Improve code maintainability: adopt PHP design patterns. For more information, please follow other related articles on the PHP Chinese website!
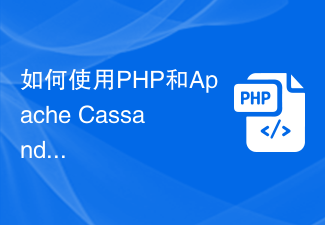
在现代互联网时代,数据极为重要。然而,随着互联网用户数量持续增长,传统的数据存储方案可能无法应对不断增长的数据量和并发读写请求。在这种环境下,需要一种可扩展的数据存储方案,这就是NoSQL数据库的主要优势之一。ApacheCassandra是一款开源的NoSQL数据库,具有极高的可扩展性和可用性,被广泛应用于大型分布式系统中。本篇文章将介绍如何使用PHP和
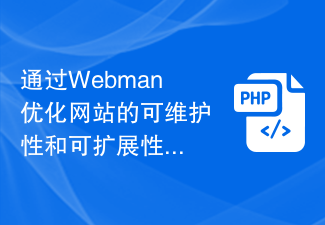
通过Webman优化网站的可维护性和可扩展性引言:在当今的数字时代,网站作为一种重要的信息传播和交流方式,已经成为了企业、组织和个人不可或缺的一部分。而随着互联网技术的不断发展,为了应对日益复杂的需求和变化的市场环境,我们需要对网站进行优化,提高其可维护性和可扩展性。本文将介绍如何通过Webman工具来优化网站的可维护性和可扩展性,并附上代码示例。一、什么是
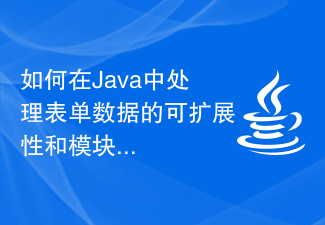
如何在Java中处理表单数据的可扩展性和模块化设计?引言:在Web应用程序开发中,表单数据处理是一个非常重要的环节。处理表单数据的有效性、可靠性和安全性对于应用程序的稳定性和用户体验至关重要。在Java中,我们可以使用多种方法来处理和验证表单数据。然而,为了使我们的代码具有良好的可扩展性和模块化设计,我们需要采取适当的策略和设计模式。本文将介绍如何在Java
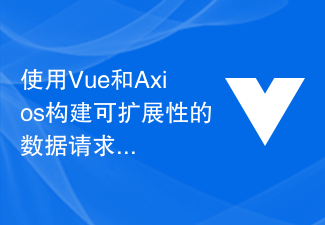
使用Vue和Axios构建可扩展性的数据请求模块在前端开发中,经常需要与后端交互获取数据。为了提高代码的可维护性和可扩展性,我们可以使用Vue和Axios来构建一个灵活的数据请求模块。Axios是一个基于Promise的HTTP客户端,它可以用于浏览器和Node.js。Axios提供了一套简洁而强大的API,可以轻松地发送HTTP请求。而Vue是一种用于构建
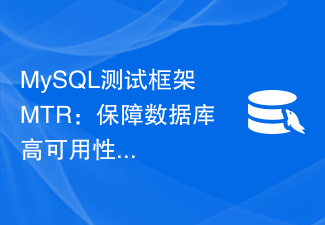
MySQL测试框架MTR:保障数据库高可用性与可扩展性的实用指南引言:对于任何一个数据驱动型应用程序来说,数据库是其核心组成部分之一。而对于大型应用程序来说,高可用性和可扩展性是至关重要的。为了保障这两个关键特性,MySQL提供了一个强大的测试框架,即MySQL测试框架(MTR)。本文将介绍MTR框架的基本概念,并通过实际代码示例演示如何使用MTR来保证数据
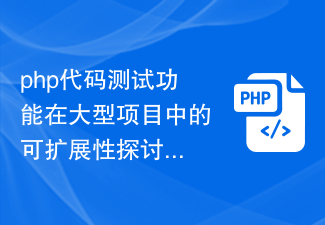
php代码测试功能在大型项目中的可扩展性探讨在开发一个大型项目时,代码的可测试性是至关重要的。测试可以帮助我们发现潜在的问题,并确保代码的正确性和稳定性。而可扩展性是指在项目的演进过程中,代码能够轻松地适应新的需求和变化。在本文中,我们将探讨php代码测试功能在大型项目中的可扩展性,并提供一些代码示例。首先,我们来讨论测试的重要性。在一个大型项目中,代码的复
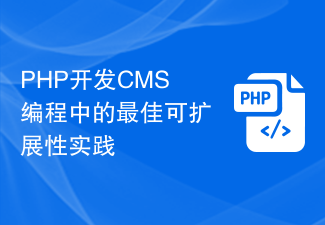
PHP开发CMS编程中的最佳可扩展性实践在当今的数字时代,内容管理系统(CMS)已成为了许多网站的重要组成部分。然而,开发一个可靠、灵活、可扩展的CMS并非易事。在开发过程中,我们必须考虑到许多因素,包括性能、可维护性和可扩展性。在本文中,我将介绍一些PHP开发CMS编程中的最佳可扩展性实践,帮助您更好地构建可扩展的CMS。使用适当的架构选择正确的架构对于实
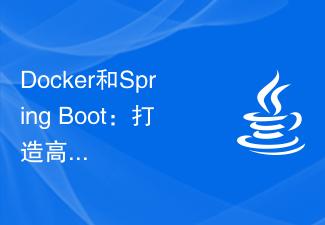
Docker和SpringBoot:打造高可用性和高可扩展性的应用架构引言:随着云计算和大数据时代的到来,应用的可用性和可扩展性成为企业关注的焦点。为了实现高可用性和高可扩展性,使用Docker容器和SpringBoot框架是一种明智的选择。本文将介绍如何使用这两个工具来构建一个具有高可用性和可扩展性的应用架构,并提供相应的代码示例。一、Docker容器


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
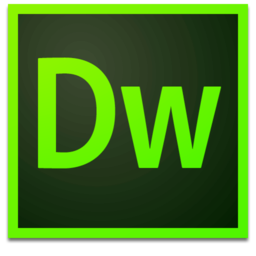
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Linux new version
SublimeText3 Linux latest version
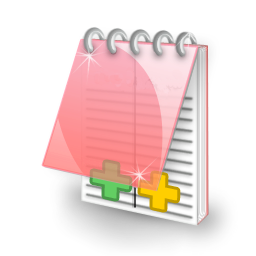
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function