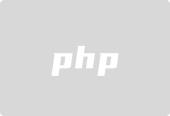
Basic optimization
-
Use the correct Python version: Newer versions of python are generally more performant, offer better memory management and built-in optimizations .
-
Choose the right library: Save time and improve performance by using purpose-built libraries instead of writing code from scratch.
-
Reduce the number of loops: If possible, avoid using nested loops. Using list comprehensions and generator expressions are more efficient alternatives.
Data structure optimization
-
Choose the right container: Lists are good for random access, dictionaries are good for fast key-value lookups, and tuples are good for immutable data.
-
Use pre-allocated memory: By pre-allocating the size of an array or list, you can reduce the overhead of memory allocation and defragmentation.
-
Using Numpy and Pandas: For scientific computing and data analysis, Numpy and pandas provide efficient array and data frame implementations.
Algorithm optimization
-
Use binary search: For sorted arrays, binary search provides a faster search algorithm than linear search.
-
Consider divide and conquer: Decomposing a large problem into smaller sub-problems can improve algorithm efficiency.
-
Use recursion or iteration: Depending on the circumstances, a recursive or iterative implementation may be more efficient.
Code optimization
-
Avoid unnecessary copies: Use pass by reference instead of pass by value to avoid creating unnecessary copies.
-
Use @property and @staticmethod: These decorators can optimize property access and static method calls.
-
Utilize multi-threading and parallel processing: For computationally intensive tasks, Multi-threading and parallel processing can increase the execution speed.
Advanced optimization
-
Using Cython and Numba: These tools can compile Python code into faster C extensions, improving the performance of numerical calculations and data processing.
-
Leveraging the CPython JIT: CPython’s JIT (just-in-time compiler) can identify and optimize certain blocks of code, thereby increasing execution speed.
-
Use a memory analyzer: Using tools such as Valgrind or Pympler, you can analyze memory usage and identify memory leaks or other problems.
Demo code
- Fast matrix multiplication using Numpy arrays:
import numpy as np
a = np.array([[1, 2], [3, 4]])
b = np.array([[5, 6], [7, 8]])
c = np.dot(a, b)# 高效矩阵乘法
- Use binary search for quick search:
def binary_search(arr, target):
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1# 未找到
- Use Cython to optimize numerical calculations:
import cython
@cython.cclass
class MyClass:
def __init__(self):
self.x = 0
def calculate(self, n):
for i in range(n):
self.x += i
in conclusion
By implementing these optimization techniques, developers can significantly improve the performance of their Python code, thereby improving application response times and the overall user experience. Remember, Performance optimization is an ongoing process that requires careful consideration of code structure, data structures, and algorithms. Through continuous optimization, developers can create Python applications that are efficient, reliable, and meet user needs.
The above is the detailed content of Python performance optimization practice: from basics to advanced. For more information, please follow other related articles on the PHP Chinese website!