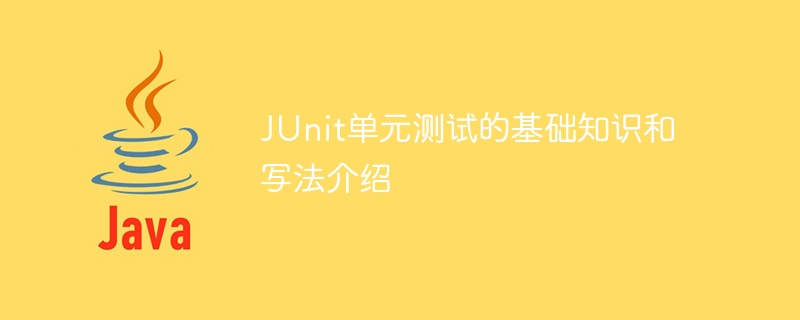
Introduction to the basic knowledge and writing methods of JUnit unit testing
Introduction:
Unit testing is a crucial part of software development, it can effectively verify The correctness and reliability of the code we write. JUnit is one of the most commonly used unit testing frameworks in Java. This article will introduce the basic knowledge and writing methods of JUnit unit testing, and provide specific code examples.
1. Basic knowledge of JUnit unit testing
- What is unit testing?
Unit testing is the testing of the smallest testable unit in the software. It usually tests independently tested functions or methods to verify whether it meets the expected design requirements. It is a white-box testing method designed to test the logical correctness and exception handling capabilities of the code.
- JUnit Introduction
JUnit is an open source Java unit testing framework, which was jointly developed by Kent Beck and Erich Gamma and others. JUnit provides a series of annotations and assertion methods to facilitate us to write and execute unit tests.
2. How to write JUnit unit tests
When writing JUnit unit tests, you usually follow the following steps:
- Import JUnit library
In Java projects , we need to import the JUnit library before we can start writing unit tests. Importing can be achieved by adding a JUnit dependency in the project's build file.
- Create a test class
Create a test class corresponding to the class being tested, and add the @Test annotation to the test class to mark the method as a test method. For example, we have a class called Calculator, we can create a test class called CalculatorTest.
- Writing test methods
In the test class, we can write multiple test methods to test the methods of the class under test. Each test method should be marked with the @Test annotation, and the method should be clearly named to indicate the expected behavior of the method under test. In the test method, we can use the assertion method to judge the return result of the method under test to verify its correctness.
- Run the test
In JUnit, we can run the test by right-clicking the test class and selecting "Run As" -> "JUnit Test". JUnit will automatically execute all test methods in the test class and display the test results in the test running window.
Specific code example:
-
Tested class
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
-
Testing class
import org.junit.Assert;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
Assert.assertEquals(5, result);
}
}
In the above example, we tested the add method of the Calculator class. In the testAdd method, we create a Calculator instance, then call the add method, and use the assertion method assertEquals to assert whether the return value of the method is equal to the expected value.
Conclusion:
The JUnit unit testing framework provides us with a simple and effective way to write and execute unit tests. By properly using JUnit's annotations and assertion methods, we can test our code more efficiently and improve software quality and reliability.
References:
- https://junit.org/junit5/docs/current/user-guide/
- https://www.baeldung. com/junit
The above is the detailed content of Introduction to the basic knowledge and writing methods of JUnit unit testing. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn