A lambda expression is an anonymous function that can be conveniently used to traverse a collection. In this article, we will introduce how to use lambda expressions to iterate over collections, and provide specific code examples.
In Python, the syntax format of lambda expression is as follows:
lambda parameter list: expression
The parameter list of lambda expression can contain one or more parameters, Separate with commas. The expression is the return value of the lambda function.
Let's look at a simple example. Suppose there is a list containing integers. We want to traverse the list and print out each element.
numbers = [1, 2, 3, 4, 5] # 使用lambda表达式遍历列表 for number in numbers: print(number)
The output result is:
1 2 3 4 5
In the above code, we use a for loop to traverse each element in the list, and then use the print function to print out the element.
In addition to using for loops, we can also use the built-in function map combined with lambda expressions to traverse the collection. The map function applies a lambda expression to each element of the collection and returns a new collection.
The following is an example of using map and lambda expressions to traverse a collection. We square all elements in a list.
numbers = [1, 2, 3, 4, 5] # 使用map和lambda表达式遍历列表并平方 squared_numbers = list(map(lambda x: x**2, numbers)) print(squared_numbers)
The output result is:
[1, 4, 9, 16, 25]
In the above code, we use the map function and lambda expression to square each element in the list. Finally, we use the list function to convert the result into a new list and print it out.
In addition to using the map function, we can also use the filter function combined with lambda expressions to traverse the collection and filter out elements that meet certain conditions.
The following is an example of using filter and lambda expressions to traverse a collection. We filter out all even numbers in a list.
numbers = [1, 2, 3, 4, 5] # 使用filter和lambda表达式遍历列表并筛选出偶数 even_numbers = list(filter(lambda x: x%2 == 0, numbers)) print(even_numbers)
The output result is:
[2, 4]
In the above code, we use the filter function and lambda expression to filter out even numbers in the list. Finally, we use the list function to convert the result into a new list and print it out.
Through the above code example, we can see the power of lambda expressions when traversing collections. It is concise and clear, and can help us complete traversal and filtering operations quickly.
In practical applications, we can flexibly use lambda expressions to traverse collections according to specific needs, thereby achieving more efficient and concise code.
The above is the detailed content of Loop over a collection using lambda expressions. For more information, please follow other related articles on the PHP Chinese website!
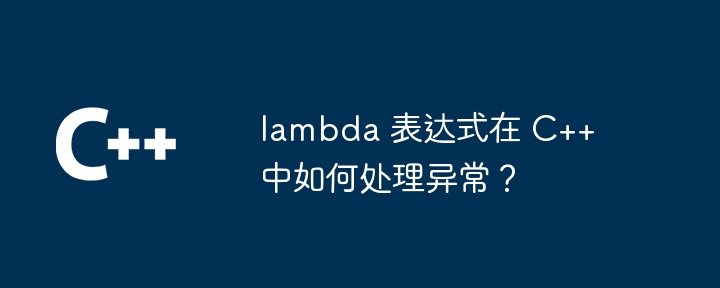
在C++中,使用Lambda表达式处理异常有两种方法:使用try-catch块捕获异常,并在catch块中处理或重新抛出异常。使用std::function类型的包装函数,其try_emplace方法可以捕获Lambda表达式中的异常。
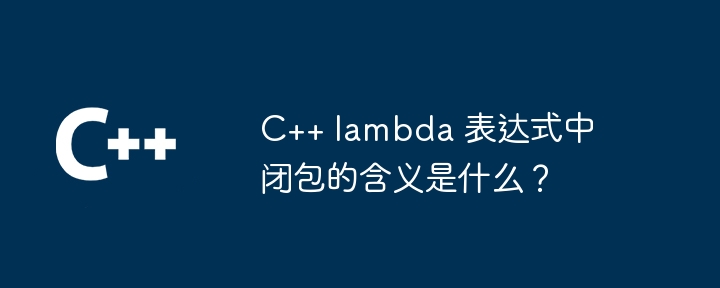
在C++中,闭包是能够访问外部变量的lambda表达式。要创建闭包,请捕获lambda表达式中的外部变量。闭包提供可复用性、信息隐藏和延迟求值等优势。它们在事件处理程序等实际情况中很有用,其中即使外部变量被销毁,闭包仍然可以访问它们。
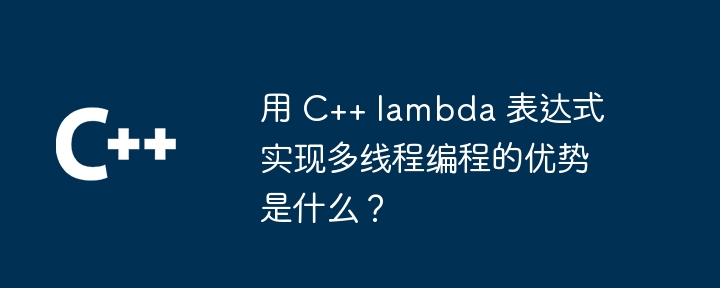
lambda表达式在C++多线程编程中的优势包括:简洁性、灵活性、易于传参和并行性。实战案例:使用lambda表达式创建多线程,在不同线程中打印线程ID,展示了该方法的简洁和易用性。
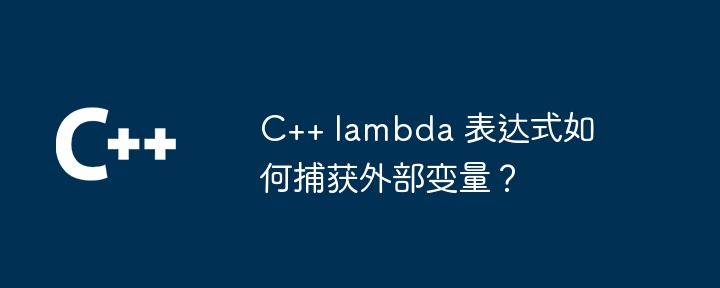
在C++中捕获外部变量的lambda表达式有三种方法:按值捕获:创建一个变量副本。按引用捕获:获得变量引用。同时按值和引用捕获:允许捕获多个变量,按值或按引用。
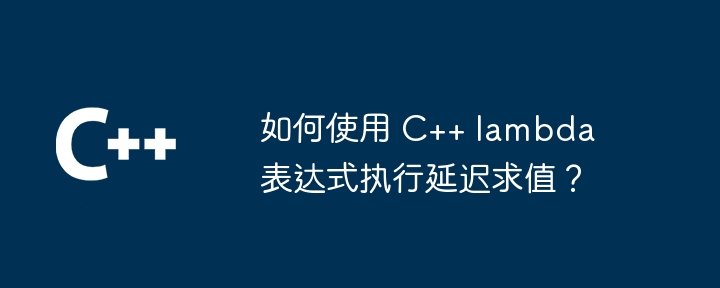
如何使用C++lambda表达式执行延迟求值?使用lambda表达式创建延迟求值的函数对象。延迟计算推迟到需要时才执行。仅当需要时才计算结果,提高性能。
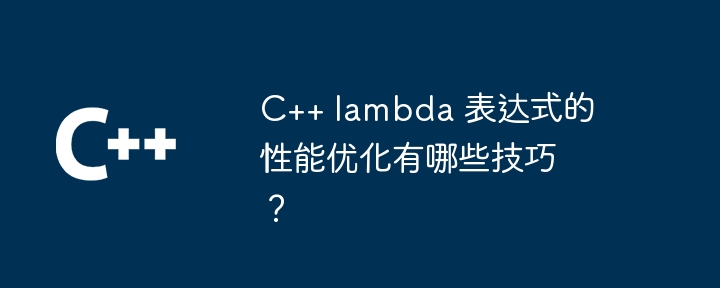
优化C++Lambda表达式的性能技巧包括:避免创建不必要的lambda对象通过std::bind显式捕获最小的对象使用std::move移动捕获的变量以避免复制优化lambda体,避免不必要的内存分配、重复计算和全局变量访问
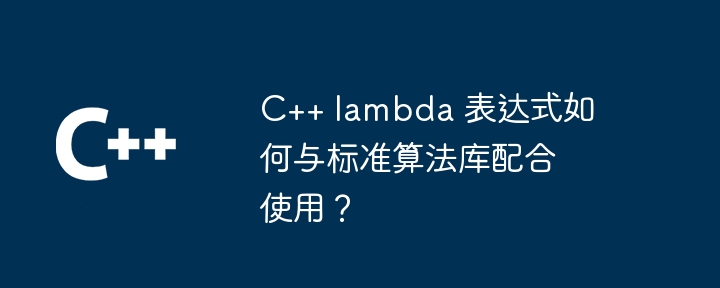
C++Lambda表达式与标准算法库紧密协作,允许创建匿名函数,简化对数据的处理。具体用途包括:排序向量:使用lambda表达式对元素进行排序。查找元素:使用lambda表达式在容器中查找特定元素。
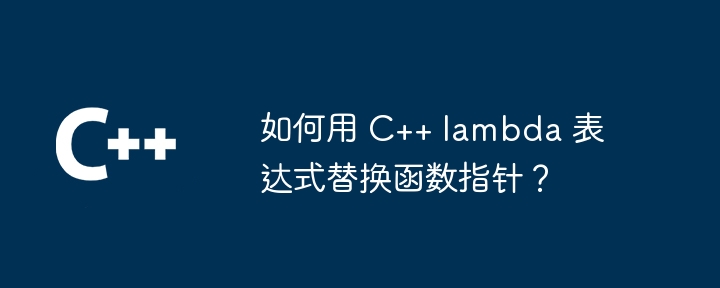
用lambda表达式替换函数指针可提升可读性、减少样板代码并提高重用性。具体而言,lambda表达式采用以下语法:[capturelist](parameterlist)->returntype{body},并可用于对向量排序等实战案例中,提升代码简洁性和可维护性。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
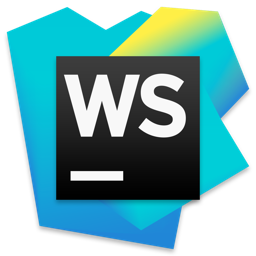
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
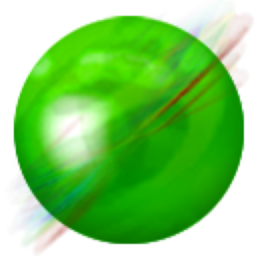
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
