


A Comprehensive Guide to PHP SPL Data Structures: Solving Data Challenges
php editor Apple brings you the most comprehensive PHP SPL data structure guide to help you easily deal with data processing problems. SPL (Standard PHP Library) provides a series of powerful data structures and algorithms, including stacks, queues, heaps, linked lists, etc., to help developers improve data processing efficiency and code quality. This guide will introduce the characteristics, uses and practical applications of each data structure in detail, allowing you to quickly master the use of data structures and solve various data problems.
PHP SPL (Standard php Library) provides a rich set of built-in data structures for efficient management and processing of data. From queues to stacks to ordered arrays and dictionaries, SPL provides developers with a wide range of tools to solve complex data processing challenges and improve code Performance and maintainability.
queue
The queue follows the first-in-first-out (FIFO) principle, meaning that the oldest added data items are removed first. This is similar to queues in the real world, such as waiting in line for service.
$queue = new SplQueue(); $queue->enqueue("Item 1"); $queue->enqueue("Item 2"); $item = $queue->dequeue(); // 获取并移除第一个元素 echo $item; // 输出 "Item 1"
Stack
Stacks follow the last-in-first-out (LIFO) principle, meaning that the last added data item is removed first. This is similar to stacking items, with items added later being removed first.
$stack = new SplStack(); $stack->push("Item 1"); $stack->push("Item 2"); $item = $stack->pop(); // 获取并移除最上面的元素 echo $item; // 输出 "Item 2"
Ordered array
SplFixedArray provides a fixed-length ordered array. Unlike PHP's standard arrays, the size of SplFixedArray cannot be dynamically adjusted.
$array = new SplFixedArray(5); $array[0] = "Item 1"; $array[1] = "Item 2"; // ... ksort($array); // 对数组中的键进行排序 foreach ($array as $key => $value) { echo "$key: $value "; }
dictionary
SplObjectStorage provides a dictionary where the keys and values are objects. It allows developers to store and retrieve data based on custom properties.
class Person { public $name; public $age; } $storage = new SplObjectStorage(); $person1 = new Person(); $person1->name = "John Doe"; $person1->age = 30; $storage[$person1] = "Person 1"; $person2 = new Person(); $person2->name = "Jane Doe"; $person2->age = 25; $storage[$person2] = "Person 2"; foreach ($storage as $person) { echo "$person->name: $storage[$person] "; }
Advanced usage
The SPL data structure provides powerful methods and properties to support more advanced data processing functions:
- count(): Returns the number of elements in the data structure.
- offsetExists(): Checks whether the specified index /key exists.
- offsetGet(): Get the element at the specified index/key.
- offsetSet(): Sets or updates the element at the specified index/key.
- offsetUnset(): Remove the element at the specified index/key.
- serialize(): Serialize the data structure into a string .
- unserialize(): Deserialize a data structure from a string.
Best Practices
When using SPL data structures, following these best practices can improve performance and code quality:
- Select the most appropriate data structure based on data processing requirements.
- Always clear objects that are no longer needed to free up memory.
- Consider using additional features of SPL data structures, such as serialization and deserialization.
- Always perform appropriate validation of data to ensure data integrity and consistency.
Summarize
PHP SPL data structures provide the tools needed to build powerful, efficient data processing applications. By understanding and leveraging these data structures, developers can effectively manage data, solve business puzzles, and improve the overall performance and usability of their applications.
The above is the detailed content of A Comprehensive Guide to PHP SPL Data Structures: Solving Data Challenges. For more information, please follow other related articles on the PHP Chinese website!
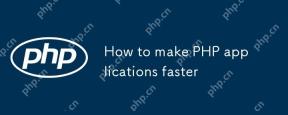
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
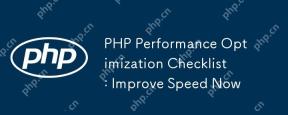
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
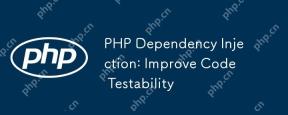
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
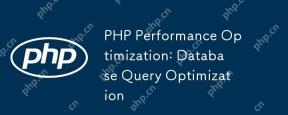
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
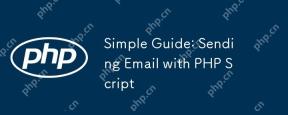
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
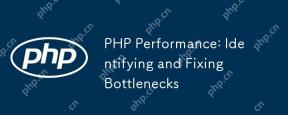
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
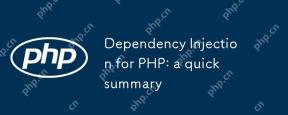
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
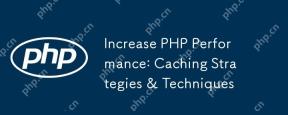
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
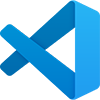
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
