


Beginner's Guide to Java JPA: Easily Understand the Basics of the Persistence Framework
#The "Java JPA Beginner's Guide" launched by php editor Baicao is designed to help beginners easily understand the basics of the persistence framework. The persistence framework is an important part of Java development, and mastering its basic concepts is crucial to learning Java programming. Through this guide, readers will be able to quickly get started with JPA and master its core concepts, laying a solid foundation for future Java development.
1. Entity
Entity is the core concept of JPA. An entity is a row of data in database, which consists of a set of attributes. Properties are columns of an entity.
2. Persistent entities
Persistent entities are entities associated with records in the database. Persistent entities can be managed through the EntityManager interface. The EntityManager interface allows you to save, update, and delete persistent entities.
3. Query language
JPA provides a query language called JPQL (Java Persistence Query Language). JPQL is a language similar to sql, but it allows you to use Java objects to query data.
4. Transaction Management
JPA provides a way to manage transactions. A transaction is a set of atomic operations that either all succeed or all fail. JPA uses the EntityManager interface to manage transactions.
The following is a demo code that shows how to use JPA to query and update data:
EntityManagerFactory emf = Persistence.createEntityManagerFactory("my-persistence-unit"); EntityManager em = emf.createEntityManager(); // 创建一个实体 Entity entity = new Entity(); entity.setName("John Doe"); entity.setAge(25); // 将实体保存到数据库 em.persist(entity); // 提交事务 em.getTransaction().commit(); // 查询实体 TypedQuery<Entity> query = em.createQuery("SELECT e FROM Entity e", Entity.class); List<Entity> entities = query.getResultList(); // 更新实体 Entity entityToUpdate = entities.get(0); entityToUpdate.setAge(26); // 提交事务 em.getTransaction().commit(); // 关闭实体管理器工厂 em.close(); emf.close();
Hope this guide is helpful to you. If you have any questions please feel free to ask, thanks for reading.
The above is the detailed content of Beginner's Guide to Java JPA: Easily Understand the Basics of the Persistence Framework. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
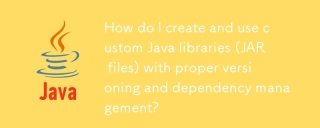
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
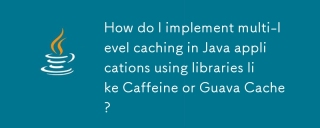
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
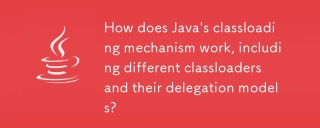
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
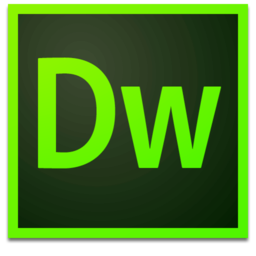
Dreamweaver Mac version
Visual web development tools
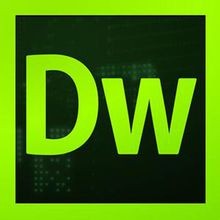
Dreamweaver CS6
Visual web development tools