Introduction
One of the most commonly used JavaScript libraries today is RequireJS. Every project I've been involved in recently has used RequireJS, or I've recommended adding RequireJS to them. In this article, I will describe what RequireJS is and some of its basic scenarios.
Asynchronous module definition (AMD)
Talking about RequireJS, you can’t get around mentioning what JavaScript modules are, and what AMD is.
JavaScript modules are just code snippets that follow SRP (Single Responsibility Principle) and expose a public API. In modern JavaScript development, you can encapsulate a lot of functionality in modules, and in most projects, each module has its own file. This makes life a bit difficult for JavaScript developers, because they need to constantly pay attention to the dependencies between modules and load these modules in a specific order, otherwise the runtime will throw errors.
The script tag is used when you want to load a JavaScript module. In order to load dependent modules, you have to load the dependent module first, and then load the dependent module. When using script tags, you need to arrange their loading in this specific order, and the scripts are loaded synchronously. You can use the async and defer keywords to make loading asynchronous, but you may lose the order of loading during the loading process. Another option is to bundle all the scripts together, but you still need to sort them in the correct order when bundling.
AMD is such a definition of a module so that the module and its dependencies can be loaded asynchronously but in the correct order.
CommonJS, is an attempt to standardize common JavaScript patterns. It contains the AMD definition which I recommend you read before continuing with this article. In ECMAScript 6, the next version of the JavaScript specification, there are specification definitions for output, input, and modules that will become part of the JavaScript language, and it won't be long. This is also what we want to say about RequireJS.
RequireJS?
RequireJS is a Javascript file and module framework that can be downloaded from http://requirejs.org/ or through Nuget if you use Visual Studio. It supports browser and server environments like node.js. Using RequireJS, you can sequentially read only the relevant dependent modules.
What RequireJS does is, when you use the script tag to load the dependencies you define, load these dependencies through the head.appendChild() function. When dependencies are loaded, RequireJS calculates the order of module definitions and calls them in the correct order. This means that all you need to do is use a "root" to read all the functionality you need, and RequireJS will do the rest. In order to use these features correctly, all modules you define need to use the RequireJS API, otherwise it will not work as expected.
RequireJS API exists under the namespace requirejs created when RequireJS is loaded. Its main API is mainly the following three functions:
- define– This function allows users to create modules. Each module has a unique module ID, which is used in the runtime function of RequireJS. The define function is a global function and does not require the use of the requirejs namespace.
- require– This function is used to read dependencies. Also it is a global function and does not need to use the requirejs namespace.
- config– This function is used to configure RequireJS.
Later, we will teach you how to use these functions, but first let us understand the loading process of RequireJS.
data-main attribute
After you download RequireJS, the first thing you have to do is understand how RequireJS starts to work. When RequireJS is loaded, it will use the data-main attribute to search for a script file (it should be the same script that loaded RequireJS using src). data-main needs to set a root path for all script files. Based on this root path, RequireJS will load all related modules. The following script is an example of using data-main:
Another way to define the root path is to use configuration functions, as we will see later. requireJs assumes that all dependencies are scripts, so you don't need to use the .js suffix when declaring a script dependency.
Configuration function
If you want to change the default configuration of RequireJS to use your own configuration, you can use the require.configh function. The config function needs to pass in an optional parameter object, which includes many configuration parameter options. Here are some configurations you can use:
- baseUrl - the root path used to load modules.
- paths - used to map module paths that do not exist under the root path.
- Shims - function dependencies and initialization functions configured outside the script/module that do not use RequireJS. Assuming underscore is not defined using RequireJS, but you still want to use it through RequireJS, then you need to define it as a shim in the configuration.
- deps - load dependency array
Here is an example of using configuration:
require.config({ //By default load any module IDs from scripts/app baseUrl: 'scripts/app', //except, if the module ID starts with "lib" paths: { lib: '../lib' }, // load backbone as a shim shim: { 'backbone': { //The underscore script dependency should be loaded before loading backbone.js deps: ['underscore'], // use the global 'Backbone' as the module name. exports: 'Backbone' } } });
In this example, the root path is set to scripts/app. Each module starting from lib is configured under the scripts/lib folder. Backbone loads a shim dependency.
Define modules with RequireJS
Modules are objects with internal implementation encapsulation, exposed interfaces and reasonably limited scope. ReuqireJS provides the define function for defining modules. By convention, only one module should be defined per Javascript file. The define function accepts a dependency array and a function containing the module definition. Usually the module definition function will receive the dependent modules in the previous array as parameters in order. For example, here is a simple module definition:
define(["logger"], function(logger) { return { firstName: “John", lastName: “Black“, sayHello: function () { logger.log(‘hello'); } } } );
We see that a module dependency array containing logger is passed to the define function, and the module will be called later. Similarly, we see that there is a parameter named logger in the defined module, which will be set to the logger module. Every module should return its API. In this example we have two properties (firstName and lastName) and a function (sayHello). Then, as long as the module you define later refers to this module by ID, you can use its exposed API.
Use the require function
Another very useful function in RequireJS is the require function. The require function is used to load module dependencies but does not create a module. For example: The following uses require to define a function that can use jQuery.
require(['jquery'], function ($) { //jQuery was loaded and can be used now });
Summary
In this article I introduced the RequireJS library, which is one of the library functions I use to create every Javascript project. It is not only used to load module dependencies and related commands, RequireJS helps us write modular JavaScript code, which is very beneficial to the scalability and reusability of the code.
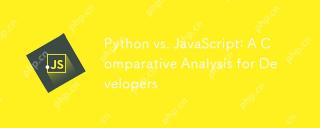
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
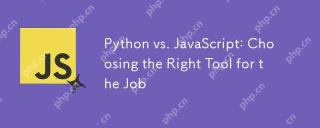
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
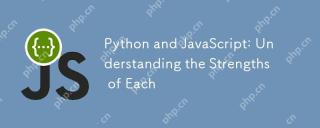
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
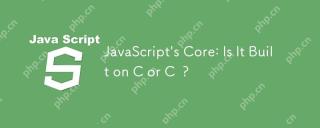
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
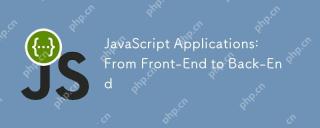
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
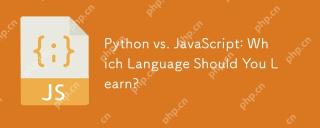
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
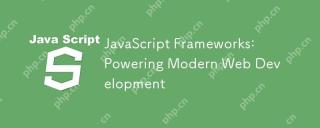
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
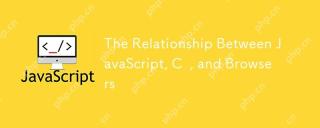
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
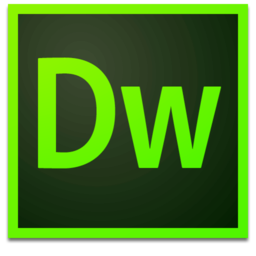
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
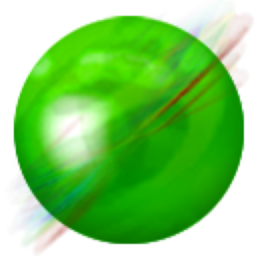
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
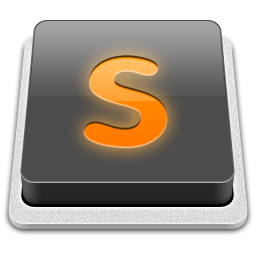
SublimeText3 Mac version
God-level code editing software (SublimeText3)
