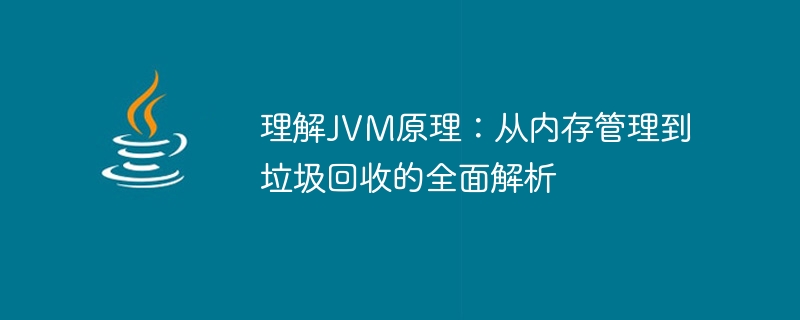
Understand the principles of JVM: a comprehensive analysis from memory management to garbage collection
With the widespread application of the Java language, the Java Virtual Machine (JVM) has become the core of Java program execution important environment. Understanding JVM principles is very important for Java developers, which can help programmers optimize code and adjust performance. This article will comprehensively analyze the JVM's memory management and garbage collection mechanism, and provide specific code examples to help readers better understand.
- JVM Overview
JVM is one of the core components of Java program execution. It is responsible for translating Java bytecode (.class file) into machine code and executing it. The JVM is independent of hardware and operating systems, which makes Java programs cross-platform.
- JVM memory structure
The memory structure of JVM mainly includes the following parts:
- Method Area: used to store metadata information of classes, such as classes, methods, field information.
- Heap: used to store object instances.
- Stack (Stack): used to store local variables, operand stacks and other data for method calls.
- Program Counter: used to record the address of the bytecode instruction executed by the current thread.
- Native Method Stack: used to store data related to local method calls.
The following is a simple code example that demonstrates the memory structure of the JVM:
public class MemoryStructureExample {
// 静态方法区
static String staticVar = "Static variable";
public static void main(String[] args) {
// 程序计数器
int pc = 0;
// 栈
int localVar = 10;
int result = add(5, 3);
System.out.println("Result: " + result);
// 堆
Object obj = new Object();
System.out.println(obj.toString());
}
// 方法区
public static int add(int a, int b) {
return a + b;
}
}
- JVM Memory Management
The JVM automatically manages memory through the garbage collection mechanism, providing With the function of automatic memory allocation and release, developers do not need to manually manage memory. The memory management of JVM mainly includes the following aspects:
- Heap memory management: Object instances dynamically created in Java programs are stored in the heap. The JVM automatically recycles no longer used objects through the garbage collector to free up memory space. The initial size and maximum size of the Java heap can be set through the
-Xms
and -Xmx
parameters.
- Stack memory management: The stack is used to store data such as local variables and operand stacks for method calls. Each thread creates a stack frame when executing a method to store method-related data. When the method is executed, the corresponding stack frame will be destroyed. The size of the stack can be set through the
-Xss
parameter.
- Method area and runtime constant pool management: The method area in the JVM is used to store metadata information of the class. The runtime constant pool is part of the method area and is used to store string constants and symbol references. The JVM uses the garbage collector to garbage collect the method area and release class information and constants that are no longer used.
- Garbage collection algorithm
There are two main types of JVM garbage collection algorithms: mark-clear algorithm and copy algorithm.
- Mark-clear algorithm: This algorithm marks objects that are no longer used and then clears them. But this algorithm has an obvious shortcoming, which will generate a lot of memory fragmentation.
- Copy algorithm: This algorithm divides the memory into two areas, namely Eden space and Survivor space. The object is first allocated to the Eden space. When the Eden space is insufficient, the Minor GC is triggered and the surviving objects are copied to the Survivor space. After multiple collections, surviving objects will be copied to the old generation. This algorithm reduces memory fragmentation, but wastes some memory space.
- Garbage Collector
JVM provides a variety of garbage collectors for performing garbage collection operations. Common garbage collectors include serial collectors, parallel collectors and CMS collectors.
- Serial Collector: The serial collector is the simplest garbage collector, using a single thread for garbage collection. Suitable for low-load application scenarios in single-core processors or multi-core processors.
- Parallel Collector: The parallel collector uses multiple threads for garbage collection and can take full advantage of multi-core processors. Suitable for high-load application scenarios in multi-core processors.
- CMS collector (Concurrent Mark and Sweep Collector): The CMS collector is a low-pause garbage collector that performs garbage collection through two stages: concurrent marking and concurrent clearing. Suitable for application scenarios with high pause time requirements.
The following is a code example that demonstrates the garbage collection mechanism of the JVM:
public class GarbageCollectionExample {
public static void main(String[] args) {
for (int i = 0; i < 1000000; i++) {
Object obj = new Object();
System.gc();
}
}
}
With the above code example, objects can be created in a loop and called after each object creation System.gc()
method triggers garbage collection operation.
Summary:
This article comprehensively analyzes the memory management and garbage collection mechanism of JVM. By understanding the JVM's memory structure, memory management and garbage collection algorithms, as well as common garbage collectors, developers can help developers better optimize code and adjust performance to improve application execution efficiency. The memory structure and garbage collection mechanism of JVM are demonstrated through specific code examples. I hope it will be helpful for readers to understand the principles of JVM.
The above is the detailed content of An in-depth exploration of the inner workings of the JVM: a detailed analysis from memory processing to garbage collection. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn