


In-depth analysis of the definition and characteristics of Java thread state
Introduction:
In Java programming, threads are an important concept. Threads allow us to handle multiple tasks at the same time, improving program execution efficiency. Thread status refers to the different states of a thread at different points in time. This article will provide an in-depth analysis of the definition and characteristics of Java thread status, and provide specific code examples to help readers better understand and apply it.
1. Definition of thread state
In the thread life cycle, the thread may experience multiple states. Java defines 6 thread states, namely: New (new), Runnable (runnable) , Blocked, Waiting, Timed Waiting and Terminated.
1.1 New (new) state
When a thread object is created through the new keyword, but the start() method has not been called, the thread is in the new state. The thread in this state has not yet started execution and does not occupy CPU resources.
1.2 Runnable (runnable) state
When the thread enters the runnable state, it means that the thread is ready and can be scheduled for execution by the JVM. In the runnable state, the thread may be scheduled by the JVM, or it may be blocked and waiting for some reasons.
1.3 Blocked state
When a thread is waiting to acquire a lock to enter a synchronized code block, the thread is in a blocked state. The thread will be temporarily suspended in this state, waiting for other threads to release the lock. Threads will not occupy CPU resources when blocked.
1.4 Waiting (waiting) state
When the thread calls the wait() method, it will enter the waiting state. In the waiting state, the thread will wait until other threads call the notify() or notifyAll() method to wake up the thread. Threads do not occupy CPU resources in the waiting state.
1.5 Timed Waiting state
Similar to the waiting state, when a thread calls a waiting method with a timeout, it will enter the timed waiting state. The thread will wait for a period of time in this state. When the timeout reaches or other threads call the notify() or notifyAll() method, the thread will be awakened. Threads will not occupy CPU resources in the scheduled waiting state.
1.6 Terminated state
When the thread completes execution or terminates due to an exception, the thread enters the terminated state. A thread in the terminated state will no longer perform any tasks.
2. Thread state conversion
2.1 Code example
In order to better understand the thread state conversion, a code example is given below:
public class ThreadStateDemo implements Runnable { public void run() { synchronized (this) { try { Thread.sleep(2000); wait(); } catch (InterruptedException e) { e.printStackTrace(); } } } public static void main(String[] args) { ThreadStateDemo obj = new ThreadStateDemo(); Thread thread = new Thread(obj); System.out.println("线程状态: " + thread.getState()); // 打印线程状态 thread.start(); System.out.println("线程状态: " + thread.getState()); // 打印线程状态 try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } synchronized (obj) { obj.notify(); } try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("线程状态: " + thread.getState()); // 打印线程状态 } }
2.2 Analysis Description
In the above code, we created a ThreadStateDemo class that implemented the Runnable interface and implemented the run() method. In the run() method, we use the synchronized keyword to lock the object, and call the sleep() and wait() methods to simulate different state transitions of the thread.
In the main() method, we create a thread object and obtain the thread state through the getState() method. Before the thread starts, the status of the thread is NEW, indicating that the thread is in a new state. After the thread is started, the thread enters the RUNNABLE state, indicating that the thread is in a runnable state. Subsequently, we use the sleep() method to make the thread sleep for 1 second to simulate the running of the thread. Then, wake up the waiting thread through the notify() method. Finally, after the thread execution is completed, the thread enters the terminated state.
By running the above code, we can observe the conversion process of thread state, thereby gaining a deeper understanding of the relationship between thread state and code execution.
Conclusion:
Six thread states are defined in Java, namely New (new), Runnable (runnable), Blocked (blocked), Waiting (waiting), Timed Waiting (timing waiting) and Terminated. With appropriate code examples, we can better understand the definition and characteristics of these states. Understanding thread status helps us better manage and schedule multi-threaded tasks and improve program execution efficiency. In actual development, we should reasonably handle thread state conversion according to business needs and thread interaction rules to achieve code security and efficiency.
The above is the detailed content of An in-depth discussion of the definition and characteristics of Java thread state. For more information, please follow other related articles on the PHP Chinese website!
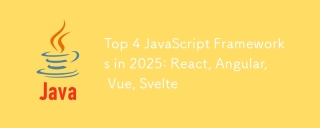
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
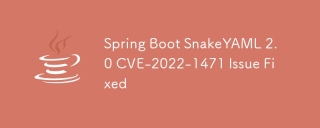
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
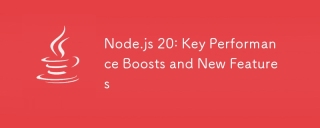
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
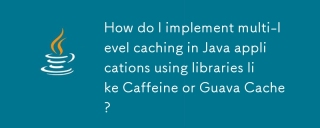
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
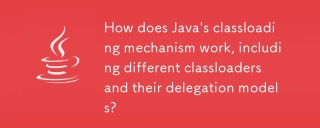
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
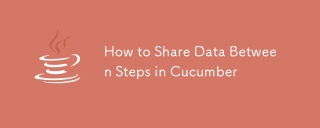
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
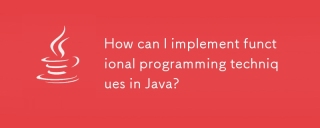
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
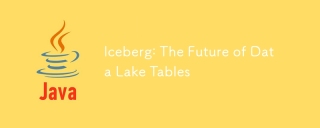
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
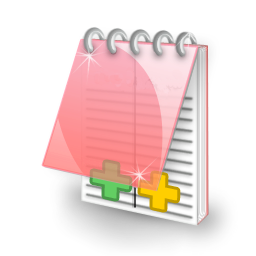
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Linux new version
SublimeText3 Linux latest version