An in-depth analysis of the three different forms of Java factory pattern
Java factory pattern is a design pattern for creating objects. It abstracts the object creation process so that it can decide which specific class to instantiate at runtime. It makes the code more maintainable, flexible and extensible by separating the instantiation logic from the client code. There are three common variants of the factory pattern, namely simple factory pattern, factory method pattern and abstract factory pattern. These three variants will be analyzed in detail below and specific code examples will be provided.
1. Simple Factory Pattern
Simple Factory Pattern (Simple Factory Pattern) is also called the static factory pattern. It is the simplest form of the factory pattern. In the simple factory pattern, we put the logic of creating objects in a simple factory class, and create objects by calling static methods of the factory class. The simple factory pattern is suitable for situations where the types of objects created are relatively small and do not change frequently.
// 抽象产品类 public abstract class Product { public abstract void use(); } // 具体产品类A public class ProductA extends Product { @Override public void use() { System.out.println("Product A is used."); } } // 具体产品类B public class ProductB extends Product { @Override public void use() { System.out.println("Product B is used."); } } // 简单工厂类 public class SimpleFactory { public static Product createProduct(String type) { if ("A".equals(type)) { return new ProductA(); } else if ("B".equals(type)) { return new ProductB(); } else { throw new IllegalArgumentException("Unsupported product type: " + type); } } } // 测试代码 public class Client { public static void main(String[] args) { Product productA = SimpleFactory.createProduct("A"); productA.use(); // Output: Product A is used. Product productB = SimpleFactory.createProduct("B"); productB.use(); // Output: Product B is used. } }
2. Factory Method Pattern
Factory Method Pattern delegates the instantiation logic to subclasses and delays the creation of objects to subclasses. Each specific product corresponds to a specific factory, and the client creates the product by calling the method of the specific factory. The factory method pattern is suitable for situations where there are many types of objects created and require flexible expansion.
// 抽象产品类 public abstract class Product { public abstract void use(); } // 具体产品类A public class ProductA extends Product { @Override public void use() { System.out.println("Product A is used."); } } // 具体产品类B public class ProductB extends Product { @Override public void use() { System.out.println("Product B is used."); } } // 抽象工厂类 public abstract class Factory { public abstract Product createProduct(); } // 具体工厂类A public class FactoryA extends Factory { @Override public Product createProduct() { return new ProductA(); } } // 具体工厂类B public class FactoryB extends Factory { @Override public Product createProduct() { return new ProductB(); } } // 测试代码 public class Client { public static void main(String[] args) { Factory factoryA = new FactoryA(); Product productA = factoryA.createProduct(); productA.use(); // Output: Product A is used. Factory factoryB = new FactoryB(); Product productB = factoryB.createProduct(); productB.use(); // Output: Product B is used. } }
3. Abstract Factory Pattern
Abstract Factory Pattern is a further extension of the factory method pattern. In the abstract factory pattern, a specific factory can create a series of related product. The client creates products by calling the methods of specific factories. Different specific factories can create different product combinations. The abstract factory pattern is suitable for situations where a series of related products need to be created and consistency needs to be maintained.
// 抽象产品类A public abstract class ProductA { public abstract void use(); } // 具体产品类A1 public class ProductA1 extends ProductA { @Override public void use() { System.out.println("Product A1 is used."); } } // 具体产品类A2 public class ProductA2 extends ProductA { @Override public void use() { System.out.println("Product A2 is used."); } } // 抽象产品类B public abstract class ProductB { public abstract void use(); } // 具体产品类B1 public class ProductB1 extends ProductB { @Override public void use() { System.out.println("Product B1 is used."); } } // 具体产品类B2 public class ProductB2 extends ProductB { @Override public void use() { System.out.println("Product B2 is used."); } } // 抽象工厂类 public abstract class AbstractFactory { public abstract ProductA createProductA(); public abstract ProductB createProductB(); } // 具体工厂类1 public class ConcreteFactory1 extends AbstractFactory { @Override public ProductA createProductA() { return new ProductA1(); } @Override public ProductB createProductB() { return new ProductB1(); } } // 具体工厂类2 public class ConcreteFactory2 extends AbstractFactory { @Override public ProductA createProductA() { return new ProductA2(); } @Override public ProductB createProductB() { return new ProductB2(); } } // 测试代码 public class Client { public static void main(String[] args) { AbstractFactory factory1 = new ConcreteFactory1(); ProductA productA1 = factory1.createProductA(); productA1.use(); // Output: Product A1 is used. ProductB productB1 = factory1.createProductB(); productB1.use(); // Output: Product B1 is used. AbstractFactory factory2 = new ConcreteFactory2(); ProductA productA2 = factory2.createProductA(); productA2.use(); // Output: Product A2 is used. ProductB productB2 = factory2.createProductB(); productB2.use(); // Output: Product B2 is used. } }
Through the above code examples, you can clearly see the specific implementation methods and application scenarios of the simple factory pattern, factory method pattern and abstract factory pattern. In actual development, we can choose a suitable factory pattern to create objects according to specific needs to achieve code maintainability, flexibility and scalability.
The above is the detailed content of An in-depth analysis of the three different forms of Java factory pattern. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
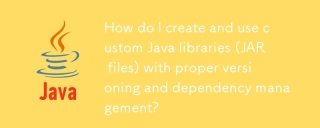
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
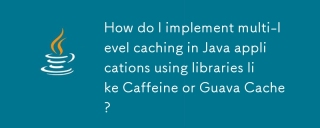
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
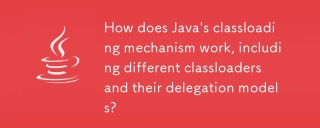
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
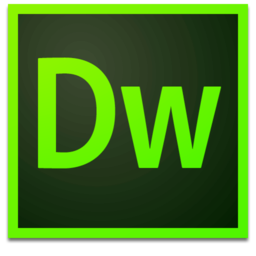
Dreamweaver Mac version
Visual web development tools
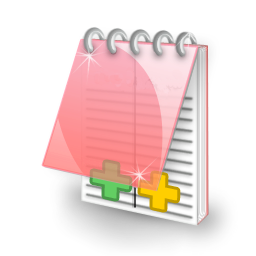
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor
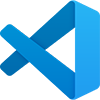
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
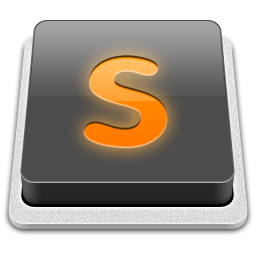
SublimeText3 Mac version
God-level code editing software (SublimeText3)