Header-based version control on Golang is an efficient way to manage code versions during development. By adding version information at the beginning of code files, developers can easily track code changes and version history. This version control method is not only easy to use, but also suitable for projects of all sizes. PHP editor Xigua will introduce in detail the usage and precautions of header-based version control on Golang in this article to help developers better manage and maintain code. Whether you are a beginner or an experienced developer, this article will provide you with valuable reference and guidance. Let’s explore this interesting and practical version control technology together!
Question content
I want to use gin to implement header-based version control on go. I'm thinking of using a middleware function to do this on the router.
The client will call the same api url and the version will be in a custom http header like this:
Call version 1 Get /users/12345678 Accepted version: v1
Calling version 2: Get /users/12345678 Accepted version: v2
Therefore, the router can recognize the header and call the specific version. Something like this:
router := gin.Default() v1 := router.Group("/v1") v1.Use(VersionMiddleware()) v1.GET("/user/:id", func(c *gin.Context) { c.String(http.StatusOK, "This is the v1 API") }) v2 := router.Group("/v2") v2.Use(VersionMiddleware()) v2.GET("/user/:id", func(c *gin.Context) { c.String(http.StatusOK, "This is the v2 API") }) func VersionMiddleware() gin.HandlerFunc { return func(c *gin.Context) { version := c.Request.Header.Get(configuration.GetConfigValue("VersionHeader")) // Construct the new URL path based on the version number path := fmt.Sprintf("/%s%s", version, c.Request.URL.Path) // Modify the request URL path to point to the new version-specific endpoint c.Request.URL.Path = path c.Next() } }
Solution
Please check the code snippet below. I use reverseproxy to redirect to a given version. You need to carefully verify a given version. Otherwise it will result in recursive calls.
Note: I used two versions of /user
get (/v1/user
and /v2/user
) .
Sample code
package main import ( "net/http" "net/http/httputil" "regexp" "github.com/gin-gonic/gin" ) func main() { router := gin.default() router.use(versionmiddleware()) v1 := router.group("/v1") v1.get("/user", func(c *gin.context) { c.string(http.statusok, "this is the v1 api") }) v2 := router.group("/v2") v2.get("/user", func(c *gin.context) { c.string(http.statusok, "this is the v2 api") }) router.run(":8082") } func versionmiddleware() gin.handlerfunc { return func(c *gin.context) { // you need to check c.request.url.path whether // already have a version or not, if it has a valid // version, return. regex, _ := regexp.compile("/v[0-9]+") ver := regex.matchstring(c.request.url.path) if ver { return } version := c.request.header.get("accept-version") // you need to validate given version by the user here. // if version is not a valid version, return error // mentioning that given version is invalid. director := func(req *http.request) { r := c.request req.url.scheme = "http" req.url.host = r.host req.url.path = "/"+ version + r.url.path } proxy := &httputil.reverseproxy{director: director} proxy.servehttp(c.writer, c.request) } }
or
You can achieve this using the gin wrapper below.
- Example
package main import ( "net/http" "github.com/gin-gonic/gin" "github.com/udayangaac/stackoverflow/golang/75860989/ginwrapper" ) func main() { engine := gin.default() router := ginwrapper.newrouter(engine) defaultrouter := router.default() defaultrouter.get("/profile",func(ctx *gin.context) { }) v1 := router.withversion("/v1") v1.get("/user",func(ctx *gin.context) { ctx.string(http.statusok, "this is the profile v1 api") }) v2 := router.withversion("/v2") v2.get("/user",func(ctx *gin.context) { ctx.string(http.statusok, "this is the profile v2 api") }) engine.run(":8082") }
- Package
package ginwrapper import ( "fmt" "net/http" "github.com/gin-gonic/gin" ) type router struct { router *gin.engine versiongroups map[string]*gin.routergroup } type versionedrouter struct { version string router } func newrouter(router *gin.engine) *router { return &router{ router: router, versiongroups: make(map[string]*gin.routergroup), } } func (a *router) default() versionedrouter { return versionedrouter{router: *a } } func (a *router) withversion(version string) versionedrouter { if _,ok := a.versiongroups[version]; ok { panic("cannot initialize same version multiple times") } a.versiongroups[version] = a.router.group(version) return versionedrouter{router: *a,version:version } } func (vr versionedrouter) get(relativepath string, handlers ...gin.handlerfunc) { vr.handle(http.methodget,relativepath,handlers...) } // note: you need to follow the same for other http methods. // as an example, we can write a method for post http method as below, // // func (vr versionedrouter) post(relativepath string, handlers ...gin.handlerfunc) { // vr.handle(http.methodpost,relativepath,handlers...) // } func (vr versionedrouter)handle(method,relativepath string, handlers ...gin.handlerfunc) { if !vr.isrouteexist(method,relativepath) { vr.router.handle(method,relativepath,func(ctx *gin.context) { version := ctx.request.header.get("accept-version") if len(version) == 0 { ctx.string(http.statusbadrequest,"accept-version header is empty") } ctx.request.url.path = fmt.sprintf("/%s%s", version, ctx.request.url.path) vr.router.handlecontext(ctx) }) } versionedrelativepath := vr.version + relativepath if !vr.isrouteexist(method,versionedrelativepath) { vr.router.handle(method,versionedrelativepath,handlers... ) } } func (a versionedrouter) isrouteexist(method,relativepath string) bool { for _,route := range a.router.routes() { if route.method == method && relativepath == route.path { return true } } return false }
Example request
/v1/user
curl --location 'localhost:8082/user' \ --header 'accept-version: v1'
/v2/user
curl --location 'localhost:8082/user' \ --header 'Accept-version: v2'
The above is the detailed content of Header based versioning on golang. For more information, please follow other related articles on the PHP Chinese website!
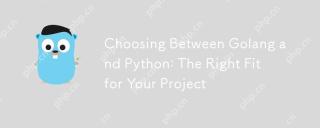
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
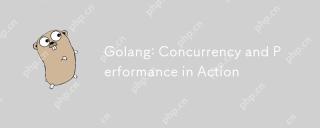
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
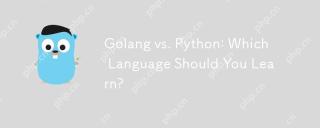
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
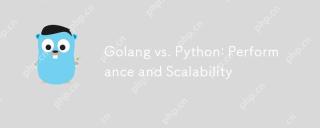
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
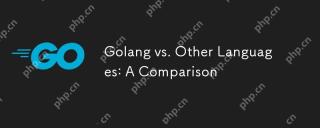
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
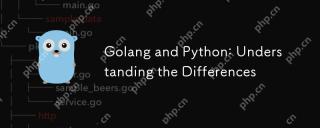
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
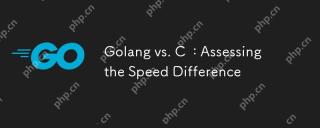
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
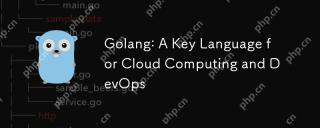
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
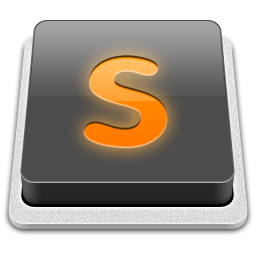
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.