


Should I use copy on private slice fields before returning them from getter?
In object-oriented programming, the getter method is usually used to obtain the value of a private member variable. However, sometimes there is a question: Should I use copying of private slice fields before returning them from the getter method? The answer to this question is not absolute and depends on the specific circumstances. In some cases, copying a private slice field can prevent external code from modifying the field, thereby protecting data consistency and security. However, in some scenarios with high performance requirements, the copy operation may cause additional overhead. In this case, you can consider directly returning the reference to the slice field. Therefore, you need to weigh the pros and cons based on the specific situation and choose the appropriate way to handle the return of private slice fields.
Question content
If struct
has a private slice field that requires a getter, should the slice be copied before returning it?
I want to confirm if it is safe to return private slices directly from the getter, since I don't want the caller to be able to modify them via the getter's return value.
From my testing so far, it seems that the slices returned are not linked to the private slice fields.
However, I'm not sure if this works in all scenarios, and I don't want to use copy
if it's not needed.
This is what I tried:
package main import "fmt" type basket struct { fruits []string } func (b *basket) fruits() []string { return b.fruits } func (b *basket) addfruit(fruit string) { b.fruits = append(b.fruits, fruit) } func main() { basket := &basket{} basket.addfruit("apple") basket.addfruit("banana") basket.addfruit("orange") fruits := basket.fruits() fmt.println(fruits) // [apple banana orange] fruits = append(fruits, "mango") fruits = append(fruits, "lemon") fruits = append(fruits, "pineapple") fmt.println(fruits) // [apple banana orange mango lemon pineapple] fmt.println(basket.fruits()) // [apple banana orange] }
The following is what the getter of copy
looks like:
func (b *Basket) Fruits() []string { result := make([]string, len(b.fruits)) copy(result, b.fruits) return result }
Workaround
As @kostix mentioned in the comments, it depends on the scenario.
For the one in the OP, we want to use copy
because we want to separate the getter's output from the private field so the caller can't modify it.
The above is the detailed content of Should I use copy on private slice fields before returning them from getter?. For more information, please follow other related articles on the PHP Chinese website!
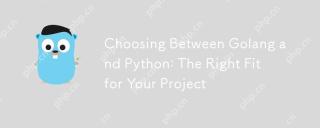
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
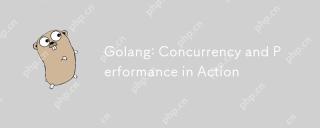
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
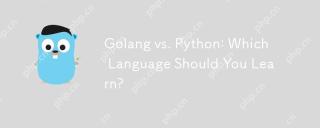
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
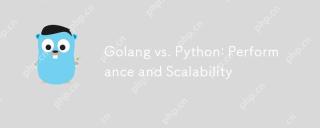
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
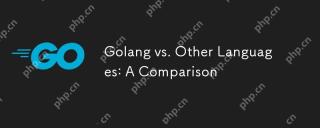
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
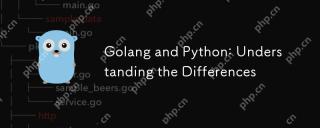
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
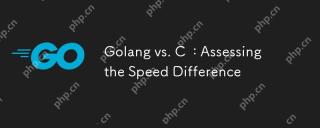
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
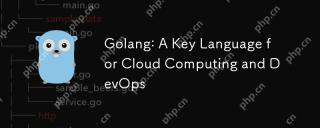
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
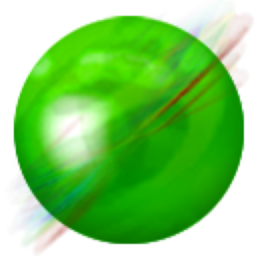
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
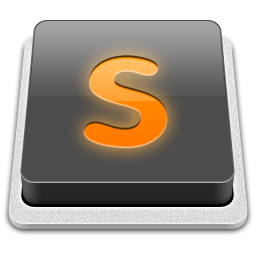
SublimeText3 Mac version
God-level code editing software (SublimeText3)
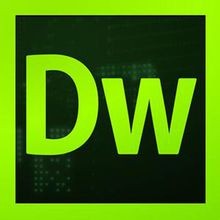
Dreamweaver CS6
Visual web development tools