Deciphering the underlying operating principles of Kafka message queue
The implementation mechanism of Kafka message queue
Kafka is a distributed publish-subscribe messaging system that allows producers to publish messages to topics , consumers can subscribe to these topics and receive messages. Kafka uses partitions to store messages, and each partition has a replica set. Each replica in the replica set stores data for that partition and can handle write requests from producers and read requests from consumers.
Kafka uses ZooKeeper to manage the metadata of the cluster, including topics, partitions, and replica sets. ZooKeeper is also used to coordinate producers and consumers. Producers use ZooKeeper to find partitions for a topic, and consumers use ZooKeeper to find partitions for subscribed topics.
Kafka message queue implementation code example
// 创建一个生产者 Producer<String, String> producer = new KafkaProducer<>(properties); // 创建一个主题 producer.createTopic("my-topic"); // 向主题发送消息 producer.send(new ProducerRecord<>("my-topic", "Hello, Kafka!")); // 关闭生产者 producer.close(); // 创建一个消费者 Consumer<String, String> consumer = new KafkaConsumer<>(properties); // 订阅主题 consumer.subscribe(Arrays.asList("my-topic")); // 轮询主题中的消息 while (true) { ConsumerRecords<String, String> records = consumer.poll(100); for (ConsumerRecord<String, String> record : records) { System.out.println(record.key() + ": " + record.value()); } } // 关闭消费者 consumer.close();
In-depth analysis of the implementation mechanism of Kafka message queue
Kafka uses partitioning to To store messages, each partition has a replica set. Each replica in the replica set stores data for that partition and can handle write requests from producers and read requests from consumers. Kafka uses ZooKeeper to manage the cluster's metadata, including topics, partitions, and replica sets. ZooKeeper is also used to coordinate producers and consumers. Producers use ZooKeeper to find partitions for a topic, and consumers use ZooKeeper to find partitions for subscribed topics.
Kafka uses a mechanism called "replication factor" to ensure message reliability. Replication factor refers to the number of replicas in the replica set. If one replica fails, the other replicas can continue to provide service. Kafka also uses a mechanism called "consistency levels" to ensure the orderliness of messages. The consistency level can be set to "all" or "one". If the consistency level is set to "all", the message must be successfully replicated by all replicas to be considered committed. If the consistency level is set to "one", a message can be considered committed as long as it has been successfully replicated by one replica.
Kafka uses a mechanism called a "partition key" to ensure even distribution of messages. The partition key is a field of a message that determines in which partition the message is stored. Kafka uses an algorithm called a "hash function" to calculate the hash value of the partition key and then distributes the messages into different partitions based on the hash value.
Kafka uses a mechanism called "offsets" to track where consumers read messages. The offset is a number that indicates how many messages the consumer has read. Consumers use offsets to tell Kafka where to start reading messages.
Kafka uses a mechanism called "commit offset" to ensure that consumers do not read messages repeatedly. When the consumer finishes reading a batch of messages, it submits the offsets to Kafka. Kafka stores committed offsets in ZooKeeper. When the consumer next reads a message, it will start reading from the committed offset.
Advantages of Kafka message queue
- High throughput: Kafka can handle millions of messages per second.
- Low latency: Kafka’s latency is very low, usually only a few milliseconds.
- Reliability: Kafka uses replication factors and consistency levels to ensure message reliability.
- Scalability: Kafka can easily scale to thousands of nodes.
- Persistence: Kafka stores messages on disk, so even if a failure occurs, messages are not lost.
Disadvantages of Kafka message queue
- Complexity: The configuration and management of Kafka is relatively complex.
- Learning curve: Kafka’s learning curve is relatively steep.
- Cost: Kafka is a commercial software that requires payment to use.
Applicable scenarios for Kafka message queue
- Real-time data processing: Kafka is very suitable for processing real-time data, such as log data, sensor data and financial data .
- Stream processing: Kafka is well suited for stream processing, such as machine learning and fraud detection.
- Messaging: Kafka is great for messaging, such as emails, text messages, and social media messages.
- Event-driven architecture: Kafka is very suitable for event-driven architecture, such as microservice architecture and IoT architecture.
The above is the detailed content of Deciphering the underlying operating principles of Kafka message queue. For more information, please follow other related articles on the PHP Chinese website!
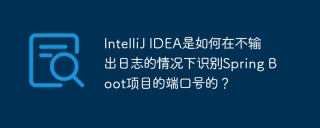
Start Spring using IntelliJIDEAUltimate version...
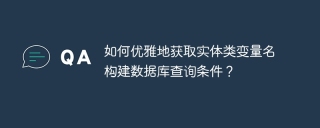
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
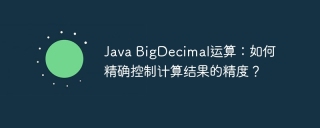
Java...
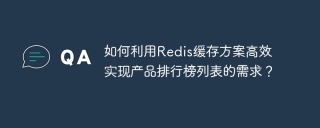
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
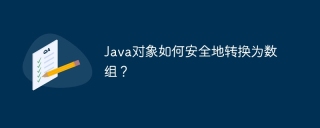
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
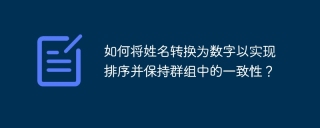
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
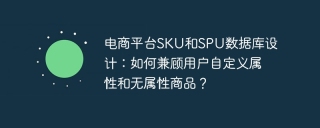
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
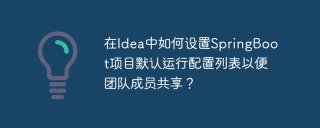
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
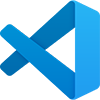
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
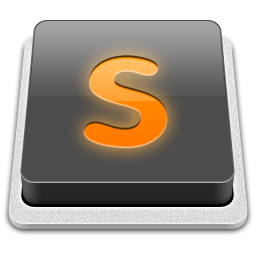
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
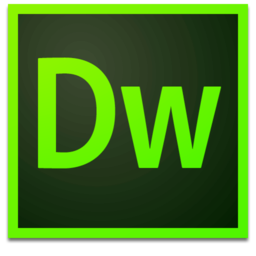
Dreamweaver Mac version
Visual web development tools