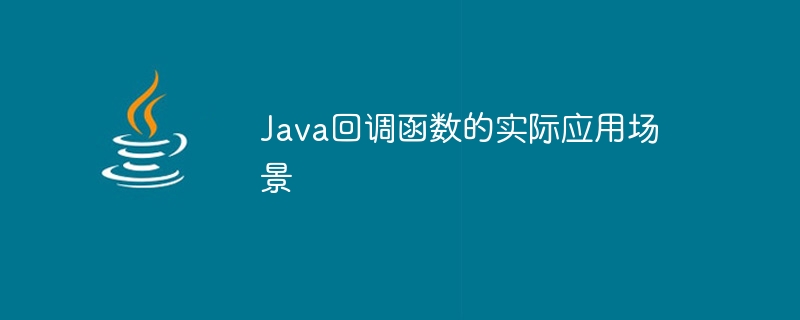
Practical application of Java callback function
The callback function is a function that is called after the asynchronous operation is completed. It allows you to perform long-running operations without blocking the main thread. Callback functions are useful in many different situations, including:
-
Network requests: When you send a network request to the server, you can specify a callback function after the request completes . This allows you to continue performing other tasks without waiting for a response from the server.
-
File I/O: When you read data from or write data to a file, you can specify a callback function after the operation is completed. This allows you to continue performing other tasks without waiting for the file I/O operation to complete.
-
Timer: When you set a timer, you can specify a callback function after the timer expires. This allows you to perform periodic tasks without blocking the main thread.
Code example of callback function
The following is a simple Java example using callback function:
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class CallbackExample {
public static void main(String[] args) {
// 创建一个线程池
ExecutorService executorService = Executors.newFixedThreadPool(1);
// 创建一个Callable任务
Callable<String> task = () -> {
// 模拟一个长时间运行的操作
Thread.sleep(5000);
// 返回结果
return "Hello, world!";
};
// 提交任务到线程池
Future<String> future = executorService.submit(task);
// 注册一个回调函数
future.addCallback(new Callback<String>() {
@Override
public void onSuccess(String result) {
// 任务成功完成时调用
System.out.println("Task completed successfully: " + result);
}
@Override
public void onFailure(Throwable throwable) {
// 任务失败时调用
System.out.println("Task failed: " + throwable.getMessage());
}
});
// 关闭线程池
executorService.shutdown();
}
}
In this example, we create a Callable task, which Simulate a long-running operation. Then we submit the task to the thread pool and register a callback function. When the task is completed, the callback function will be called and the result will be printed.
Advantages of callback functions
There are many advantages to using callback functions, including:
- ##Improving performance: Callback functions can improve performance because They allow you to perform long-running operations without blocking the main thread. This allows your application to continue to respond to user interaction even if long-running operations are executing in the background.
- Improve scalability: Callback functions can improve scalability because they allow you to delegate long-running operations to multiple threads. This allows your application to handle more concurrent requests.
- Improve code readability and maintainability: Callback functions can improve code readability and maintainability because they allow you to combine long-running operations with your application's Other parts separate. This makes your application easier to understand and maintain.
Disadvantages of callback functions
There are also some disadvantages to using callback functions, including:
- Increased code complexity: Callback Functions can increase code complexity because you need to manage the registration and invocation of callback functions.
- May cause callback hell: If you nest too many callback functions, it may cause callback hell. This is a situation where one callback function calls another callback function and so on. This makes it difficult to track the order in which the code was executed, and can lead to errors.
Conclusion
Callback functions are a powerful tool that can be used to improve application performance, scalability, and code readability. However, you also need to be careful when using callback functions to avoid increased code complexity and callback hell.
The above is the detailed content of Scenarios for practical application of Java callback functions. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn