How to implement division and rounding in Golang
Practical method of division and rounding in Golang
In programming languages, division operation is one of the very common operations. However, in some cases we may want to get the result of integer division instead of the default floating point division result. In Golang, there are several ways to achieve this. This article will introduce some practical methods of division and rounding in Golang and provide specific code examples.
Method 1: Use type conversion
A simple method is to use type conversion to convert the floating point result into an integer. This can be achieved using the built-in int() function. Here is a sample code:
package main import "fmt" func main() { var dividend, divisor float64 = 10, 3 result := int(dividend / divisor) fmt.Println(result) // 输出:3 }
In this example, we use two floating point numbers dividend
and divisor
. By converting the division result to an integer, we get the desired integer result.
Method 2: Use the remainder operator
Another method is to use the remainder operator%
to calculate the integer part of the quotient. Here is a sample code:
package main import "fmt" func main() { var dividend, divisor int = 10, 3 result := dividend / divisor + dividend % divisor fmt.Println(result) // 输出:3 }
In this example, we use two integers dividend
and divisor
. By calculating the integer part of the quotient and adding the remainder, we obtain the desired integer result.
Method 3: Use the function in the math package
Another method is to use the function in the math package to implement division and rounding. Here is a sample code:
package main import ( "fmt" "math" ) func main() { var dividend, divisor float64 = 10, 3 result := math.Trunc(dividend / divisor) fmt.Println(result) // 输出:3 }
In this example, we use two floating point numbers dividend
and divisor
. By using the Trunc function from the math package, we get the desired integer result.
Summary
This article introduces three methods to implement division and rounding in Golang: using type conversion, using the remainder operation and using functions in the math package. Depending on your specific needs, you can choose a suitable method to achieve the desired integer result. Whether it is a simple type conversion or a more complex mathematical operation, you can choose the most suitable method according to the actual situation.
The above is the detailed content of How to implement division and rounding in Golang. For more information, please follow other related articles on the PHP Chinese website!
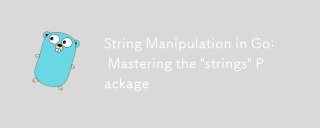
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
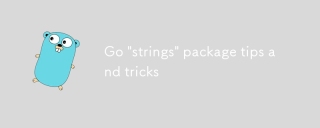
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
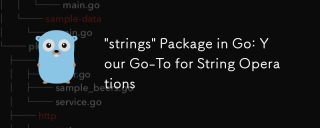
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
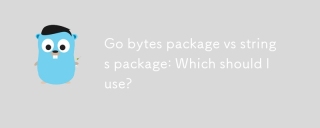
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
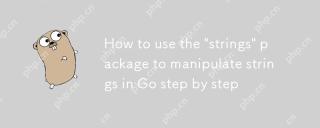
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
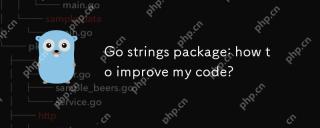
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
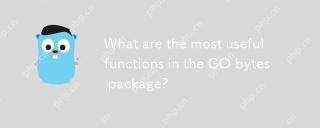
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
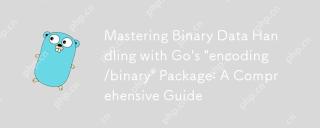
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
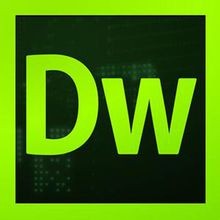
Dreamweaver CS6
Visual web development tools
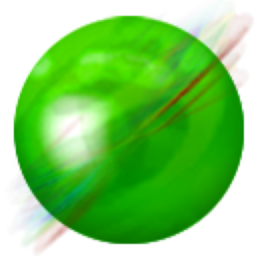
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
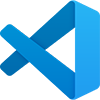
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
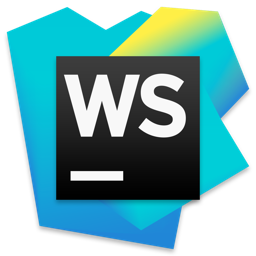
WebStorm Mac version
Useful JavaScript development tools
