


Detailed explanation of numpy functions: from entry to proficiency
Introduction:
In the field of data science and machine learning, numpy is a very important Python library. It provides efficient and powerful multi-dimensional array manipulation tools, making processing large-scale data easy and fast. This article will introduce in detail some of the most commonly used functions in the numpy library, including array creation, indexing, slicing, operations, and transformations, and will also give specific code examples.
1. Array creation
-
Use the numpy.array() function to create an array.
import numpy as np # 创建一维数组 arr1 = np.array([1, 2, 3, 4, 5]) print(arr1) # 创建二维数组 arr2 = np.array([[1, 2, 3], [4, 5, 6]]) print(arr2) # 创建全0/1数组 arr_zeros = np.zeros((2, 3)) print(arr_zeros) arr_ones = np.ones((2, 3)) print(arr_ones) # 创建指定范围内的数组 arr_range = np.arange(0, 10, 2) print(arr_range)
2. Array indexing and slicing
-
Use index to access array elements.
import numpy as np arr = np.array([1, 2, 3, 4, 5]) print(arr[0]) print(arr[2:4])
-
Use Boolean indexing to select elements that meet the condition.
import numpy as np arr = np.array([1, 2, 3, 4, 5]) print(arr[arr > 3])
3. Array operations
-
Basic operations on arrays.
import numpy as np arr1 = np.array([1, 2, 3]) arr2 = np.array([4, 5, 6]) # 加法 print(arr1 + arr2) # 减法 print(arr1 - arr2) # 乘法 print(arr1 * arr2) # 除法 print(arr1 / arr2) # 矩阵乘法 print(np.dot(arr1, arr2))
-
Aggregation operations on arrays.
import numpy as np arr = np.array([1, 2, 3, 4, 5]) # 求和 print(np.sum(arr)) # 求最大值 print(np.max(arr)) # 求最小值 print(np.min(arr)) # 求平均值 print(np.mean(arr))
4. Array transformation
-
Use the reshape() function to change the shape of the array.
import numpy as np arr = np.arange(10) print(arr) reshaped_arr = arr.reshape((2, 5)) print(reshaped_arr)
-
Use the flatten() function to convert a multi-dimensional array into a one-dimensional array.
import numpy as np arr = np.array([[1, 2, 3], [4, 5, 6]]) print(arr) flatten_arr = arr.flatten() print(flatten_arr)
Conclusion:
This article provides a detailed introduction to some common functions of the numpy library, including operations such as array creation, indexing, slicing, operations, and transformations. The powerful functions of the numpy library can help us process large-scale data efficiently and improve the efficiency of data science and machine learning. I hope this article can help readers better understand and apply the functions of the numpy library, and be able to use them flexibly in practice.
Reference:
- https://numpy.org/doc/stable/reference/
The above is the detailed content of Numpy function: comprehensive analysis and in-depth application. For more information, please follow other related articles on the PHP Chinese website!
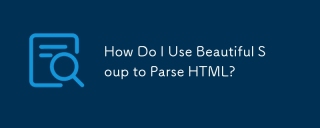
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
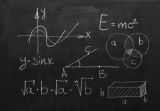
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
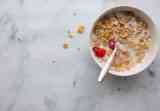
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
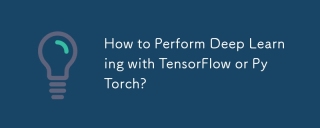
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
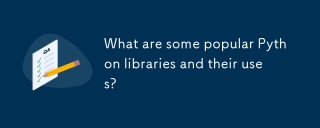
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
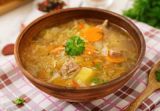
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
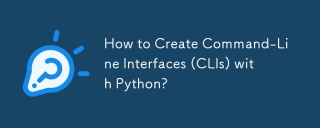
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
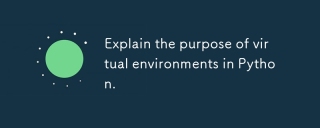
The article discusses the role of virtual environments in Python, focusing on managing project dependencies and avoiding conflicts. It details their creation, activation, and benefits in improving project management and reducing dependency issues.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
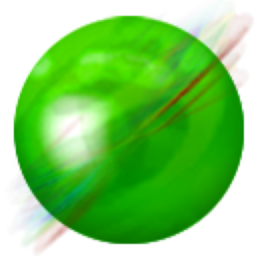
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
