


Deal with data sorting problems easily: simple and easy-to-understand pandas sorting guide
Simple and easy-to-understand pandas sorting tutorial: allows you to easily deal with data sorting problems, specific code examples are needed
In data analysis and processing, it is often necessary to perform data sorting Sort to better understand the characteristics and patterns of the data. In Python, the pandas library is one of the important tools for data analysis and processing. This tutorial explains how to use pandas to sort data quickly and flexibly, and provides specific code examples.
1. Basic concepts of data sorting
Before sorting, we need to understand the basic concepts of data sorting. In pandas, there are two main ways to sort data: sorting by row and sorting by column.
Sort by row: Sort the entire row of data according to the value of a certain column or columns. This can quickly find out the ranking of a certain column or columns of data.
Sort by column: Sort the entire column of data according to numerical size. This sorts the data according to a certain characteristic, making it easier to understand and analyze.
2. Sort by row
1. Sort by single column
First, we need to create a simple data set to demonstrate the process of data sorting.
import pandas as pd data = {'姓名': ['张三', '李四', '王五', '赵六'], '年龄': [25, 32, 28, 19], '分数': [80, 90, 85, 75]} df = pd.DataFrame(data)
Next, we can use the "sort_values" function to sort the data. By default, this function sorts ascending order by the specified column.
df_sorted = df.sort_values(by='年龄') print(df_sorted)
The running results are as follows:
姓名 年龄 分数 3 赵六 19 75 0 张三 25 80 2 王五 28 85 1 李四 32 90
You can see that after sorting by the "age" column, the data is sorted in ascending order.
2. Sort by multiple columns
If we need to sort by multiple columns, we only need to pass in multiple column names in the "by" parameter.
df_sorted = df.sort_values(by=['年龄', '分数']) print(df_sorted)
The running results are as follows:
姓名 年龄 分数 3 赵六 19 75 0 张三 25 80 2 王五 28 85 1 李四 32 90
As you can see, the data is first sorted by the "age" column, and then sorted by the "score" column.
3. Sort by column
Sort by column is mainly to sort the entire column of data according to numerical size in order to better understand and analyze the data.
1. Sort by column name
We can use the "sort_index" function to sort the columns. By default, this function sorts alphabetically by column name.
df_sorted = df.sort_index(axis=1) print(df_sorted)
The running results are as follows:
分数 年龄 姓名 0 80 25 张三 1 90 32 李四 2 85 28 王五 3 75 19 赵六
You can see that the data is sorted in alphabetical order by the column names "Score", "Age", and "Name".
2. Sort by column data
We can also sort based on the size of the column data, just pass in the column data in the "by" parameter.
df_sorted = df.sort_values(by='年龄', axis=1) print(df_sorted)
The running results are as follows:
姓名 分数 年龄 0 张三 80 25 1 李四 90 32 2 王五 85 28 3 赵六 75 19
As you can see, the data is first sorted by the "age" column, and then sorted by the corresponding column data.
4. Other sorting parameters
In addition to the basic sorting method, pandas also provides some other useful sorting parameters, such as ascending sorting, descending sorting, missing value processing, etc.
In the "sort_values" function, we can use the "ascending" parameter to specify ascending or descending sorting. By default, this parameter is "True", which sorts in ascending order.
df_sorted = df.sort_values(by='年龄', ascending=False) print(df_sorted)
The running results are as follows:
姓名 年龄 分数 1 李四 32 90 2 王五 28 85 0 张三 25 80 3 赵六 19 75
As you can see, the data is sorted in descending order according to the "age" column.
In addition to ascending and descending sorting, we can also handle missing values during the sorting process. In the "sort_values" function, we can use the "na_position" parameter to specify how missing values are handled. By default, this parameter is "last", which sorts missing values last; when this parameter is set to "first", it sorts missing values first.
data = {'姓名': ['张三', '李四', '王五', None], '年龄': [25, None, 28, 19], '分数': [80, 90, 85, 75]} df = pd.DataFrame(data) df_sorted = df.sort_values(by='年龄', na_position='first') print(df_sorted)
The running results are as follows:
姓名 年龄 分数 1 李四 NaN 90 3 None 19.0 75 0 张三 25.0 80 2 王五 28.0 85
You can see that when sorting by the "age" column, the missing values are placed first.
To sum up, this tutorial introduces a simple and easy-to-understand pandas sorting tutorial, including sorting by row and sorting by column, and provides specific code examples. By studying this tutorial, I believe you can easily deal with data sorting problems and use it flexibly in data analysis and processing.
The above is the detailed content of Deal with data sorting problems easily: simple and easy-to-understand pandas sorting guide. For more information, please follow other related articles on the PHP Chinese website!
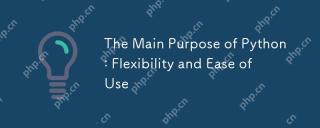
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
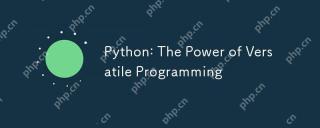
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
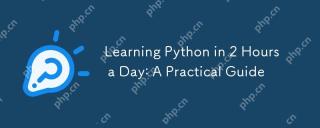
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.
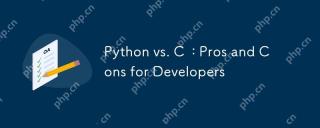
Python is suitable for rapid development and data processing, while C is suitable for high performance and underlying control. 1) Python is easy to use, with concise syntax, and is suitable for data science and web development. 2) C has high performance and accurate control, and is often used in gaming and system programming.
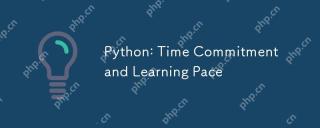
The time required to learn Python varies from person to person, mainly influenced by previous programming experience, learning motivation, learning resources and methods, and learning rhythm. Set realistic learning goals and learn best through practical projects.
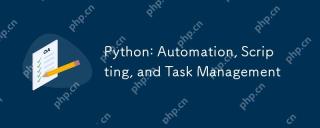
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
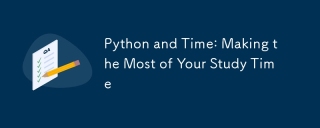
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
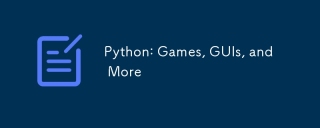
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
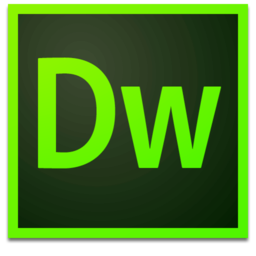
Dreamweaver Mac version
Visual web development tools
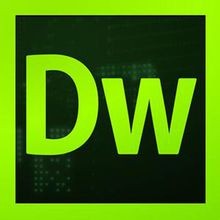
Dreamweaver CS6
Visual web development tools