Python: Automation, Scripting, and Task Management
Python excels in automation, scripting, and task management. 1) Automation: implement file backup through standard libraries such as os and shutil. 2) Scripting: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
introduction
What do you think of when we talk about Python? Is it its concise syntax or a powerful library ecosystem? Today we are going to explore in-depth the application of Python in automation, scripting and task management. Through this article, you will learn how Python can be the best in these fields and master some practical tips and best practices.
Review of basic knowledge
Python shines in automation and scripting mainly because of its ease of use and rich library support. Let's briefly review the relevant basics:
- Automation : refers to the automatic execution of repetitive tasks through programming to reduce manual intervention.
- Scripting : Write small programs to complete specific tasks, often used for system management or data processing.
- Task management : involves scheduling tasks, monitoring task status and processing task results.
Python's standard libraries such as os
, sys
and subprocess
provide powerful system operation capabilities, while third-party libraries such as schedule
and apscheduler
make task scheduling a breeze.
Core concept or function analysis
Python application in automation
Automation is a major strength of Python. Whether it is file processing, data collection or system management, Python can easily deal with it. Let's look at a simple automation example:
import os import shutil # Automatic file backup def backup_files(source_dir, backup_dir): if not os.path.exists(backup_dir): os.makedirs(backup_dir) for filename in os.listdir(source_dir): source_path = os.path.join(source_dir, filename) backup_path = os.path.join(backup_dir, filename) shutil.copy2(source_path, backup_path) # Use example source_directory = '/path/to/source' backup_directory = '/path/to/backup' backup_files(source_directory, backup_directory)
This simple script shows how Python automates file backups through standard libraries. It works by iterating over files in the source directory and copying them into the backup directory.
Python application in scripting
Scripting is another important application scenario in Python. Let's look at a simple script example for monitoring system resources:
import psutil def monitor_system(): cpu_percent = psutil.cpu_percent(interval=1) memory = psutil.virtual_memory() disk = psutil.disk_usage('/') print(f"CPU Usage: {cpu_percent}%") print(f"Memory Usage: {memory.percent}%") print(f"Disk Usage: {disk.percent}%") if __name__ == "__main__": monitor_system()
This script uses the psutil
library to get CPU, memory, and disk usage. It works by calling psutil
's API to get real-time data of system resources.
Python in task management
Task management is a natural extension of Python in automation and scripting. Let's look at a simple task scheduling example:
import schedule import time def job(): print("I'm working...") schedule.every(10).minutes.do(job) # Execute while True every 10 minutes: schedule.run_pending() time.sleep(1)
This script uses schedule
library to schedule tasks and executes job
functions every 10 minutes. It works by setting the execution frequency of tasks through schedule
library and constantly checking in the main loop whether there are tasks to be executed.
Example of usage
Basic usage
Let's look at a more complex automation example for batch processing of images:
from PIL import Image import os def resize_images(source_dir, target_dir, size): if not os.path.exists(target_dir): os.makedirs(target_dir) for filename in os.listdir(source_dir): if filename.endswith(('.png', '.jpg', '.jpeg')): with Image.open(os.path.join(source_dir, filename)) as img: img = img.resize(size, Image.LANCZOS) img.save(os.path.join(target_dir, filename)) # Use example source_directory = '/path/to/source' target_directory = '/path/to/target' resize_images(source_directory, target_directory, (300, 300))
This script uses the PIL
library to resize images in batches. It iterates over image files in the source directory, resizes them to the specified size, and saves them to the target directory.
Advanced Usage
Let's look at a more complex script example to monitor the availability of a website:
import requests from time import sleep import smtplib from email.mime.text import MIMEText def check_website(url): try: response = requests.get(url) response.raise_for_status() return True except requests.RequestException: return False def send_alert(email, subject, body): msg = MIMEText(body) msg['Subject'] = subject msg['From'] = 'alert@example.com' msg['To'] = email with smtplib.SMTP('smtp.example.com', 587) as server: server.starttls() server.login('username', 'password') server.send_message(msg) def monitor_website(url, email): While True: If not check_website(url): send_alert(email, 'Website Down', f'The website {url} is currently down.') sleep(60) # Check once a minute# Use example website_url = 'https://example.com' alert_email = 'user@example.com' monitor_website(website_url, alert_email)
This script uses the requests
library to check the availability of the website and uses the smtplib
library to send alert emails when the website is unavailable. It checks the availability of the website every minute through an infinite loop and sends an alert immediately when a problem is detected.
Common Errors and Debugging Tips
There are some common problems you may encounter when using Python for automation, scripting, and task management:
- Permissions Issue : Make sure your script has sufficient permissions to access and operate the file system.
- Dependency Issue : Make sure that all required libraries are installed correctly, it is recommended to use a virtual environment to manage dependencies.
- Network problem : When processing network requests, pay attention to handling timeouts and connection errors.
Debugging Tips:
- Logging : Use the
logging
module to record the script execution process to help locate problems. - Exception handling : Use
try-except
block to catch and handle possible exceptions to avoid script crashes. - Debugging tools : Use
pdb
or IDE's own debugging tools to execute code step by step and view variable status.
Performance optimization and best practices
In practical applications, how to optimize Python code to improve the efficiency of automation, scripting and task management?
- Using asynchronous programming : For I/O-intensive tasks, using the
asyncio
library can significantly improve performance. For example, when monitoring multiple websites, requests can be sent in parallel:
import asyncio import aiohttp async def check_website(session, url): try: async with session.get(url) as response: response.raise_for_status() return True except aiohttp.ClientError: return False async def monitor_websites(urls): async with aiohttp.ClientSession() as session: tasks = [check_website(session, url) for url in urls] results = await asyncio.gather(*tasks) for url, result in zip(urls, results): If not result: print(f'{url} is down') # Use example urls = ['https://example1.com', 'https://example2.com'] asyncio.run(monitor_websites(urls))
Code readability : Write clear and detailed code to improve the maintainability of the code. For example, add comments to explain complex logic using meaningful variable names and function names.
Modular design : divide the code into multiple modules or functions to improve the reusability and testability of the code. For example, encapsulate different task logic into independent functions for easy testing and maintenance.
Performance testing : Use
timeit
module or other performance testing tools to evaluate the execution efficiency of the code, identify bottlenecks and optimize. For example, compare the performance differences between different algorithm implementations:
import timeit def method1(): result = 0 for i in range(1000000): result = i return result def method2(): Return sum(range(1000000)) print("Method 1:", timeit.timeit(method1, number=10)) print("Method 2:", timeit.timeit(method2, number=10))
With these tips and best practices, you can better leverage Python to enable automation, scripting, and task management, improving productivity and code quality.
In practical applications, I have encountered a project that requires regular collection of data from multiple data sources and processing it. Due to the large amount of data and the high acquisition frequency, I used asynchronous programming to process data acquisition tasks in parallel, which greatly improved efficiency. At the same time, I also used logging and exception handling to ensure the stability and maintainability of the system.
Hopefully this article will provide you with some useful insights and practical experience to help you achieve greater success in Python automation, scripting and task management.
The above is the detailed content of Python: Automation, Scripting, and Task Management. For more information, please follow other related articles on the PHP Chinese website!
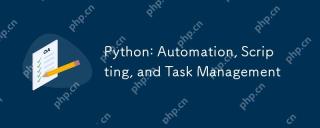
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
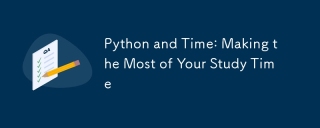
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
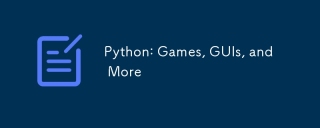
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
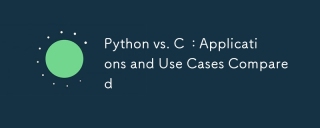
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
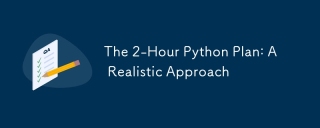
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
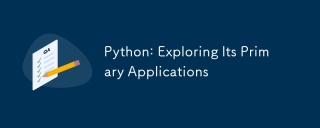
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
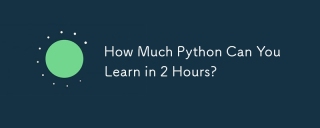
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
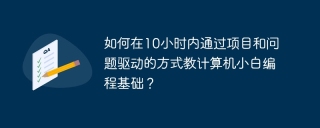
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.