Master the principles and steps of method calling in Java test classes
Understand the principles and steps of method invocation in Java test classes
In Java development, the writing of test classes is an important part of ensuring code quality and functional correctness. Among them, method invocation is one of the most common operations in test classes. An in-depth understanding of the principles and steps of method calls in Java test classes will help improve testing efficiency and write more robust test cases.
- Principle
In Java, method invocation is completed through object instances. Each Java class can create an object instance and then call methods in the class through the object instance. The principle of method calling is to call the method defined in the class to which the object belongs through the reference of the object instance. - Steps
In the test class, the steps for calling methods are as follows:
(1) Introduce the required dependency classes: The test class must first introduce the required dependencies kind. Dependencies determine the visibility of method calls.
import org.junit.Test; import com.example.MyClass;
(2) Create an object instance: Before calling a method, you need to create an object instance first. Object instances are the prerequisite for method calls.
MyClass myObject = new MyClass();
(3) Calling method: Call the required method through the object instance. Method calls need to meet access control permissions, and the method must be defined in the class being called.
myObject.myMethod();
(4) Test result assertion: In a test class, it is usually necessary to assert the results of method calls. Assertions verify whether the method is correct by judging whether the expected results are consistent with the actual results.
assertEquals(expectedResult, actualResult);
In step (4), you can use the assertion method provided by testing frameworks such as JUnit to determine whether the expected results and actual results are equal.
The following is a complete sample code that demonstrates the method calling process in the test class:
import org.junit.Test; import static org.junit.Assert.assertEquals; public class MyClassTest { @Test public void testMyMethod() { // 创建对象实例 MyClass myObject = new MyClass(); // 调用方法 int actualResult = myObject.myMethod(); // 预期结果 int expectedResult = 10; // 断言 assertEquals(expectedResult, actualResult); } }
In the above sample code, first by introducing org.junit.Test
and static org.junit.Assert.assertEquals
are two classes to use the test annotations and assertion methods provided by the JUnit framework. Then, a testMyMethod
method is created in the MyClassTest
class to test the invocation of the myMethod
method in the MyClass
class. In the test method, the object instance myObject
of MyClass
is first created, and then the myMethod
method is called, and the result is saved in the actualResult
variable middle. Subsequently, the expected result expectedResult
is declared, and finally the assertion method assertEquals
is used to determine the result.
Summary
It is very important for Java developers to understand the principles and steps of method calling in Java test classes. Through specific code example demonstrations, you can more clearly understand the process of method calling and the writing method of test classes. I hope that the introduction in this article can help readers better understand the principles and steps of method calling in Java test classes.
The above is the detailed content of Master the principles and steps of method calling in Java test classes. For more information, please follow other related articles on the PHP Chinese website!
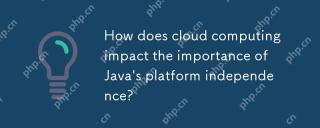
Cloud computing significantly improves Java's platform independence. 1) Java code is compiled into bytecode and executed by the JVM on different operating systems to ensure cross-platform operation. 2) Use Docker and Kubernetes to deploy Java applications to improve portability and scalability.
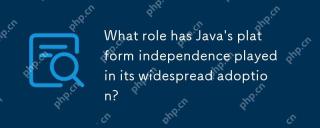
Java'splatformindependenceallowsdeveloperstowritecodeonceandrunitonanydeviceorOSwithaJVM.Thisisachievedthroughcompilingtobytecode,whichtheJVMinterpretsorcompilesatruntime.ThisfeaturehassignificantlyboostedJava'sadoptionduetocross-platformdeployment,s
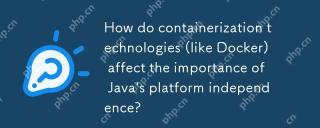
Containerization technologies such as Docker enhance rather than replace Java's platform independence. 1) Ensure consistency across environments, 2) Manage dependencies, including specific JVM versions, 3) Simplify the deployment process to make Java applications more adaptable and manageable.
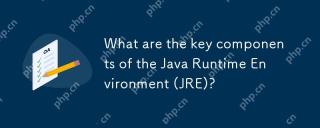
JRE is the environment in which Java applications run, and its function is to enable Java programs to run on different operating systems without recompiling. The working principle of JRE includes JVM executing bytecode, class library provides predefined classes and methods, configuration files and resource files to set up the running environment.
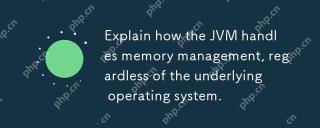
JVM ensures efficient Java programs run through automatic memory management and garbage collection. 1) Memory allocation: Allocate memory in the heap for new objects. 2) Reference count: Track object references and detect garbage. 3) Garbage recycling: Use the tag-clear, tag-tidy or copy algorithm to recycle objects that are no longer referenced.
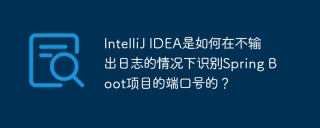
Start Spring using IntelliJIDEAUltimate version...
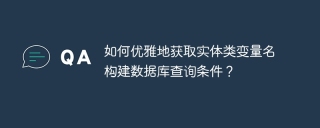
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
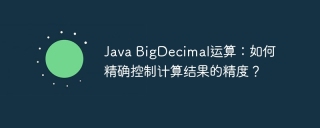
Java...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.