


Explore the Linux caching mechanism: detailed explanation of memory, disk and file system caching
In-depth understanding of Linux cache mechanism: memory cache, disk cache and file system cache
Introduction: In Linux system, cache is an important mechanism for Accelerate data access and improve system performance. This article will delve into the three caching mechanisms in Linux: memory caching, disk caching, and file system caching, and provide specific code examples to help readers better understand and use these caching mechanisms.
1. Memory cache
Memory cache means that the Linux system caches file data on the disk in the memory to reduce frequent reads and writes to the disk, thereby speeding up data access. The memory cache in the Linux system mainly consists of page cache. When an application reads a file, the operating system reads the contents of the file into the page cache and stores them in memory. The next time the file is read, the operating system first checks whether the cache data for the file exists in the page cache. If it exists, it reads it directly from the cache instead of accessing the disk again. This mechanism can significantly improve file access speed.
The following is a simple C code example that shows how to use the memory cache:
#include <stdio.h> #include <stdlib.h> #include <fcntl.h> #include <unistd.h> #include <sys/types.h> #include <sys/stat.h> #include <sys/mman.h> int main() { int fd; struct stat sb; char *file_data; // 打开文件 fd = open("test.txt", O_RDONLY); if (fd == -1) { perror("open"); exit(1); } // 获取文件大小 if (fstat(fd, &sb) == -1) { perror("fstat"); exit(1); } // 将文件映射到内存中 file_data = mmap(NULL, sb.st_size, PROT_READ, MAP_PRIVATE, fd, 0); if (file_data == MAP_FAILED) { perror("mmap"); exit(1); } // 通过内存访问文件内容 printf("%s", file_data); // 解除内存映射 if (munmap(file_data, sb.st_size) == -1) { perror("munmap"); exit(1); } // 关闭文件 close(fd); return 0; }
The above code uses the mmap function to map the file into memory and access the file content through the pointer file_data. In this way, the contents of the file will be cached in memory, and the file contents can be read directly the next time it is accessed, without the need to access the disk again.
2. Disk cache
In addition to the memory cache, the Linux system also has an important caching mechanism which is the disk cache. Disk caching means that Linux uses part of the memory as a cache for disk I/O to improve the performance of disk access. When an application performs a disk read or write operation, the operating system first caches the data in memory and then writes the data to disk. This mechanism can reduce frequent access to the disk and improve disk read and write efficiency.
The following is a simple C code example that shows how to use the disk cache:
#include <stdio.h> #include <stdlib.h> #include <fcntl.h> #include <unistd.h> int main() { int fd; char buffer[512]; // 打开文件 fd = open("test.txt", O_WRONLY | O_CREAT, S_IRUSR | S_IWUSR); if (fd == -1) { perror("open"); exit(1); } // 写入文件 write(fd, buffer, sizeof(buffer)); // 刷新文件缓冲 fsync(fd); // 关闭文件 close(fd); return 0; }
The above code uses the write function to write data to the file, and refreshes the file buffer through the fsync function. In this way, data is first cached in memory and then written to disk uniformly. This mechanism can significantly improve disk write performance.
3. File system cache
The file system cache refers to the cache used by the file system in the Linux system and is used to accelerate file system access. The file system cache mainly consists of file system data structures and metadata (such as file permissions, creation time, etc.). When an application performs file system operations, the operating system caches relevant data in memory to improve file system access speed.
The following is a simple C code example that shows how to use the file system cache:
#include <stdio.h> #include <stdlib.h> #include <fcntl.h> #include <unistd.h> #include <sys/types.h> #include <sys/stat.h> int main() { int fd; // 打开文件 fd = open("test.txt", O_RDONLY); if (fd == -1) { perror("open"); exit(1); } // 修改文件权限 if (fchmod(fd, S_IRUSR | S_IWUSR) == -1) { perror("fchmod"); exit(1); } // 关闭文件 close(fd); return 0; }
The above code uses the fchmod function to modify the permissions of the file. In this way, file-related information will be cached in memory and can be used directly in subsequent file accesses, improving the efficiency of file operations.
Conclusion:
This article deeply explores the three caching mechanisms in Linux: memory cache, disk cache and file system cache, and provides specific code examples. By understanding and using these caching mechanisms, you can improve system performance and speed up data access. I hope this article will help readers understand and apply the Linux caching mechanism.
The above is the detailed content of Explore the Linux caching mechanism: detailed explanation of memory, disk and file system caching. For more information, please follow other related articles on the PHP Chinese website!
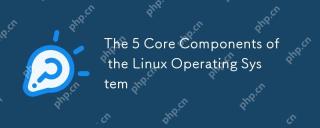
The five core components of the Linux operating system are: 1. Kernel, 2. System libraries, 3. System tools, 4. System services, 5. File system. These components work together to ensure the stable and efficient operation of the system, and together form a powerful and flexible operating system.
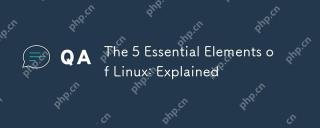
The five core elements of Linux are: 1. Kernel, 2. Command line interface, 3. File system, 4. Package management, 5. Community and open source. Together, these elements define the nature and functionality of Linux.
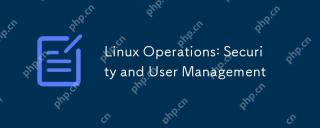
Linux user management and security can be achieved through the following steps: 1. Create users and groups, using commands such as sudouseradd-m-gdevelopers-s/bin/bashjohn. 2. Bulkly create users and set password policies, using the for loop and chpasswd commands. 3. Check and fix common errors, home directory and shell settings. 4. Implement best practices such as strong cryptographic policies, regular audits and the principle of minimum authority. 5. Optimize performance, use sudo and adjust PAM module configuration. Through these methods, users can be effectively managed and system security can be improved.
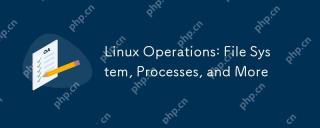
The core operations of Linux file system and process management include file system management and process control. 1) File system operations include creating, deleting, copying and moving files or directories, using commands such as mkdir, rmdir, cp and mv. 2) Process management involves starting, monitoring and killing processes, using commands such as ./my_script.sh&, top and kill.
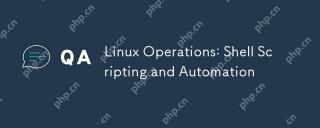
Shell scripts are powerful tools for automated execution of commands in Linux systems. 1) The shell script executes commands line by line through the interpreter to process variable substitution and conditional judgment. 2) The basic usage includes backup operations, such as using the tar command to back up the directory. 3) Advanced usage involves the use of functions and case statements to manage services. 4) Debugging skills include using set-x to enable debugging mode and set-e to exit when the command fails. 5) Performance optimization is recommended to avoid subshells, use arrays and optimization loops.
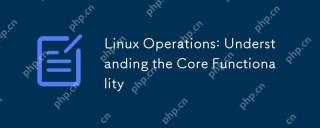
Linux is a Unix-based multi-user, multi-tasking operating system that emphasizes simplicity, modularity and openness. Its core functions include: file system: organized in a tree structure, supports multiple file systems such as ext4, XFS, Btrfs, and use df-T to view file system types. Process management: View the process through the ps command, manage the process using PID, involving priority settings and signal processing. Network configuration: Flexible setting of IP addresses and managing network services, and use sudoipaddradd to configure IP. These features are applied in real-life operations through basic commands and advanced script automation, improving efficiency and reducing errors.
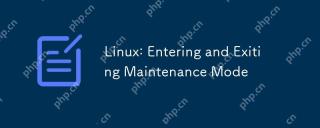
The methods to enter Linux maintenance mode include: 1. Edit the GRUB configuration file, add "single" or "1" parameters and update the GRUB configuration; 2. Edit the startup parameters in the GRUB menu, add "single" or "1". Exit maintenance mode only requires restarting the system. With these steps, you can quickly enter maintenance mode when needed and exit safely, ensuring system stability and security.
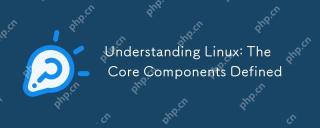
The core components of Linux include kernel, shell, file system, process management and memory management. 1) Kernel management system resources, 2) shell provides user interaction interface, 3) file system supports multiple formats, 4) Process management is implemented through system calls such as fork, and 5) memory management uses virtual memory technology.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
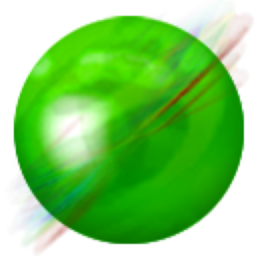
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version
