Analyzing the mystery of Golang multi-threaded programming
Decrypting the secrets of multi-threaded programming in Golang requires specific code examples
In today's software development field, multi-threaded programming has become a common need. Multi-threaded programming can make full use of the advantages of multi-core processors to improve the running efficiency and response speed of the program. However, multi-threaded programming also brings some challenges, such as thread safety, synchronization and resource contention.
Golang is an open source programming language that natively supports multi-threaded programming and provides a powerful concurrency model. This article will reveal the mysteries of multi-threaded programming in Golang and provide some specific code examples to help readers understand and apply.
- goroutine
Goroutine in Golang is a lightweight thread that can create thousands of goroutines in a program without causing significant overhead. We can use the keyword go to create a goroutine and use anonymous functions to wrap the code blocks that need to be run.
package main import "fmt" func main() { go func() { fmt.Println("Hello, World!") }() // 等待goroutine执行完成 time.Sleep(time.Second) }
In the above example, a goroutine is created using the go keyword, which will asynchronously execute the anonymous function fmt.Println("Hello, World!") in the background. Note that in order to ensure that the goroutine execution is completed, the main thread needs to wait for a certain period of time. We use the time.Sleep function to pause for one second.
- channel
Golang uses channels to implement communication between goroutines. Channel is a type-safe, concurrency-safe data structure that can be used for read and write operations. We can use the built-in make function to create a channel and use the
package main import "fmt" func main() { ch := make(chan int) go func() { ch <- 42 }() value := <-ch fmt.Println(value) }
In the above example, we created an integer channel and sent the value 42 to the channel in a goroutine. In the main thread, we use the
- Concurrency safety
In multi-threaded programming, resource competition is a very common problem. In order to solve the problem of resource competition, Golang provides mutex locks and read-write locks.
Mutex is an exclusive lock that allows only one goroutine to access the locked resource. We can use Mutex from the sync package to create a mutex and use its Lock and Unlock methods to lock and unlock resources.
package main import ( "fmt" "sync" ) var ( count int mutex sync.Mutex ) func main() { for i := 0; i < 1000; i++ { go increment() } // 等待所有goroutine执行完成 time.Sleep(time.Second) fmt.Println(count) } func increment() { mutex.Lock() count++ mutex.Unlock() }
In the above example, we use the mutex lock mutex to protect access to the shared variable count. In the increment function, use the mutex.Lock and mutex.Unlock methods to lock and unlock when updating the count variable.
Read-write lock (RWMutex) is a more flexible lock that allows multiple goroutines to read shared resources at the same time, but only allows one writing goroutine to perform write operations. We can use RWMutex in the sync package to create a read-write lock, and use its RLock and RUnlock methods for read operations, and its Lock and Unlock methods for write operations.
- select statement
In concurrent programming, it is often necessary to wait for one or more of multiple goroutines to complete a certain task before continuing execution. Golang provides select statements to solve this problem.
The select statement is used to select one of multiple communication operations for execution. Once a certain communication operation can be executed, the remaining communication operations will be ignored. We can use the select statement to wait for read and write operations on the channel, as well as timeout operations, etc.
package main import ( "fmt" "time" ) func main() { ch1 := make(chan string) ch2 := make(chan string) go func() { time.Sleep(time.Second) ch1 <- "Hello" }() go func() { time.Sleep(2 * time.Second) ch2 <- "World" }() for i := 0; i < 2; i++ { select { case msg1 := <-ch1: fmt.Println(msg1) case msg2 := <-ch2: fmt.Println(msg2) } } }
In the above example, we created two string type channels and sent data to these two channels in two goroutines. In the main thread, we use the select statement to wait for data in these two channels. Once the data is readable, it will be printed.
The above are some mysteries and practical skills of multi-threaded programming in Golang. Through features such as goroutines, channels, mutex locks, read-write locks, and select statements, we can easily write concurrency-safe programs and take advantage of the performance advantages of multi-core processors. I hope the above examples can help readers better understand and apply multi-threaded programming in Golang.
The above is the detailed content of Analyzing the mystery of Golang multi-threaded programming. For more information, please follow other related articles on the PHP Chinese website!
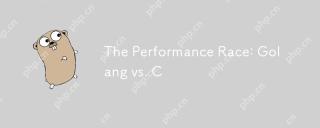
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
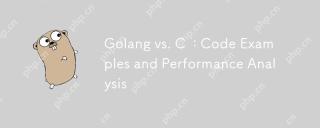
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
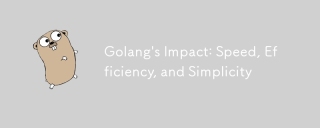
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
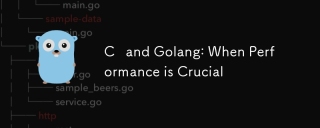
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
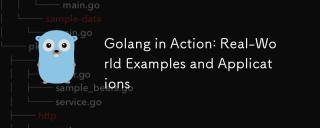
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
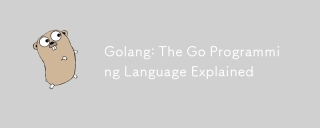
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
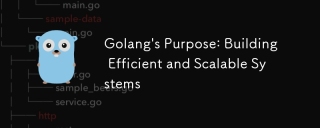
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
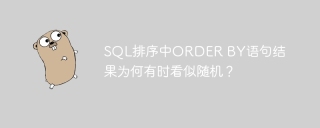
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
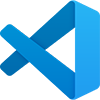
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.