


Comprehensive list of commonly used functions in the Numpy library: quick start and practice guide
The Numpy library is one of the most commonly used data processing libraries in Python. It is widely loved by data analysts for its efficient and convenient operation methods. In the Numpy library, there are many commonly used functions that can help us complete data processing tasks quickly and efficiently. This article will introduce some commonly used Numpy functions, and provide code examples and practical application scenarios so that readers can get started with the Numpy library faster.
1. Create an array
- numpy.array
Function prototype: numpy.array(object, dtype=None, copy=True, order= 'K', subok=False, ndmin=0)
Function description: Convert objects such as lists into arrays.
Code example:
import numpy as np a = np.array([1, 2, 3]) print(a) # 输出 [1 2 3]
- numpy.zeros
Function prototype: numpy.zeros(shape, dtype=float, order='C')
Function description: Create an all-zero array of the specified shape.
Code example:
import numpy as np a = np.zeros((2, 3)) print(a) # 输出 [[0. 0. 0.] # [0. 0. 0.]]
- numpy.ones
Function prototype: numpy.ones(shape, dtype=None, order='C')
Function description: Create an all-one array of the specified shape.
Code example:
import numpy as np a = np.ones((2, 3)) print(a) # 输出 [[1. 1. 1.] # [1. 1. 1.]]
- numpy.arange
Function prototype: numpy.arange(start, stop, step, dtype=None)
Function description: Create an arithmetic sequence array.
Code example:
import numpy as np a = np.arange(0, 10, 2) print(a) # 输出 [0 2 4 6 8]
2. Array operations
- numpy.reshape
Function prototype: numpy.reshape(a , newshape, order='C')
Function description: Convert array a into a new array of specified shape.
Code example:
import numpy as np a = np.array([1, 2, 3, 4, 5, 6]) b = a.reshape((2, 3)) print(b) # 输出 [[1 2 3] # [4 5 6]]
- numpy.transpose
Function prototype: numpy.transpose(a, axes=None)
Function description: Transpose the array.
Code example:
import numpy as np a = np.array([[1, 2, 3], [4, 5, 6]]) b = np.transpose(a) print(b) # 输出 [[1 4] # [2 5] # [3 6]]
- numpy.concatenate
Function prototype: numpy.concatenate((a1, a2, ...), axis= 0)
Function description: perform splicing operation on arrays.
Code example:
import numpy as np a = np.array([[1, 2], [3, 4]]) b = np.array([[5, 6], [7, 8]]) c = np.concatenate((a, b), axis=0) print(c) # 输出 [[1 2] # [3 4] # [5 6] # [7 8]]
3. Array calculation
- numpy.abs
Function prototype: numpy.abs(x , args, *kwargs)
Function description: Calculate the absolute value of each element in the array.
Code example:
import numpy as np a = np.array([-1, 2, -3]) b = np.abs(a) print(b) # 输出 [1 2 3]
- numpy.round
Function prototype: numpy.round(a, decimals=0, out=None)
Function description: Round the elements in the array.
Code example:
import numpy as np a = np.array([1.3, 2.6, 3.2]) b = np.round(a) print(b) # 输出 [1. 3. 3.]
- numpy.sum
Function prototype: numpy.sum(a, axis=None)
Function description: Calculate the sum of each element in the array.
Code example:
import numpy as np a = np.array([[1, 2], [3, 4]]) b = np.sum(a, axis=0) print(b) # 输出 [4 6]
4. Commonly used mathematical functions
- numpy.exp
Function prototype: numpy.exp(x , args, *kwargs)
Function description: Calculate the exponential function value of each element in the array.
Code example:
import numpy as np a = np.array([1, 2, 3]) b = np.exp(a) print(b) # 输出 [ 2.71828183 7.3890561 20.08553692]
- numpy.log
Function prototype: numpy.log(x, args, *kwargs )
Function description: Calculate the natural logarithm of each element in the array.
Code example:
import numpy as np a = np.array([1, 2, 3]) b = np.log(a) print(b) # 输出 [0. 0.69314718 1.09861229]
- numpy.sqrt
Function prototype: numpy.sqrt(x, args, *kwargs )
Function description: Calculate the square root of each element in the array.
Code example:
import numpy as np a = np.array([1, 4, 9]) b = np.sqrt(a) print(b) # 输出 [1. 2. 3.]
5. Practical application scenarios
- Simulating polynomial function
import numpy as np import matplotlib.pyplot as plt x = np.linspace(-5, 5, num=50) y = np.power(x, 3) - 3 * np.power(x, 2) + 2 * x + 1 plt.plot(x, y) plt.show()
- Array weighted sum
import numpy as np a = np.array([1, 2, 3, 4]) b = np.array([0.1, 0.2, 0.3, 0.4]) result = np.sum(a * b) print(result) # 输出 2.0
- Sort arrays
import numpy as np a = np.array([3, 2, 1, 4]) b = np.sort(a) print(b) # 输出 [1 2 3 4]
Summary:
This article introduces some common functions and application scenarios of the Numpy library. Including the creation, operation, calculation of arrays, and some mathematical functions. We can use these functions flexibly according to actual application scenarios to make data processing more efficient and convenient. It is recommended that readers write the code themselves and practice it to deepen their understanding and mastery of the Numpy library.
The above is the detailed content of Comprehensive list of commonly used functions in the Numpy library: quick start and practice guide. For more information, please follow other related articles on the PHP Chinese website!
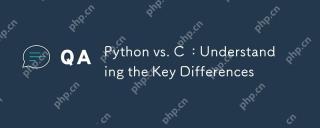
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
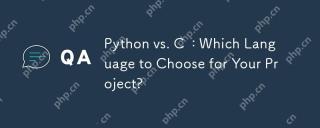
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
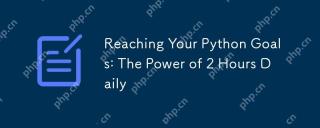
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
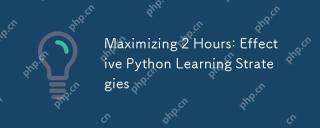
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
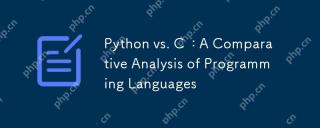
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
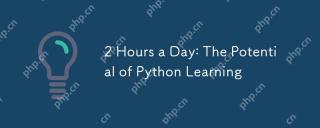
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
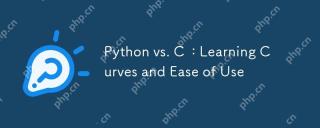
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
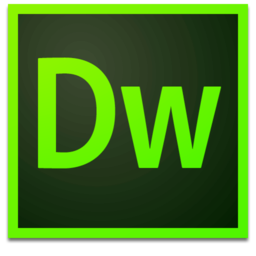
Dreamweaver Mac version
Visual web development tools
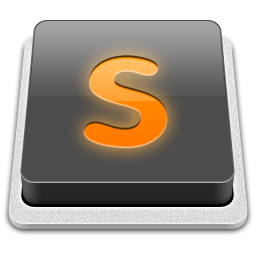
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
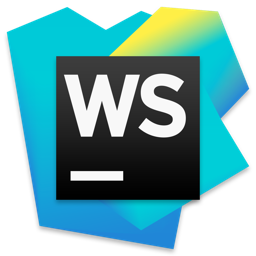
WebStorm Mac version
Useful JavaScript development tools