Master the application of data structures in Go language
Understand the data structure and its application in Go language
As an open source, high-performance programming language, Go language has concise syntax, efficient concurrency model and A powerful type system, it is widely used in modern programming. As an important basic knowledge in computer science, data structure is also of great significance to the use and application of programming languages. This article will introduce common data structures in the Go language and illustrate its application scenarios through specific code examples.
1. Array
Array is one of the most common data structures in the Go language. It is a fixed-size container that can store elements of the same type. By accessing elements in the array through subscripts, operations such as traversing, searching, sorting, and modification can be performed.
The sample code is as follows:
package main import "fmt" func main() { var arr [5]int // 声明一个长度为5的整型数组 arr[0] = 1 // 修改元素的值 arr[2] = 3 fmt.Println(arr) // 输出整个数组 for i := 0; i < len(arr); i++ { fmt.Println(arr[i]) // 遍历数组并输出每个元素 } }
2. Slice
A slice is a dynamic length reference to an array, which provides a convenient, flexible and efficient way to process collection data. Through the operation of slices, operations such as dynamic growth, appending, deletion and interception can be achieved.
The sample code is as follows:
package main import "fmt" func main() { // 声明一个切片,并初始化其中的元素 nums := []int{1, 2, 3, 4, 5} fmt.Println(nums) // 输出整个切片 // 切片追加元素 nums = append(nums, 6) fmt.Println(nums) // 切片删除元素 nums = append(nums[:2], nums[3:]...) fmt.Println(nums) // 切片截取 subNums := nums[1:3] fmt.Println(subNums) }
3. LinkedList
The linked list is a common dynamic data structure. It consists of a series of nodes. Each node Both contain data and a pointer to the next node. Linked lists are suitable for insertion and deletion operations, but accessing elements requires traversing the linked list, which is less efficient.
The sample code is as follows:
package main import "fmt" type Node struct { data int next *Node } func printList(head *Node) { for head != nil { fmt.Println(head.data) head = head.next } } func main() { // 创建链表 head := &Node{data: 1} a := &Node{data: 2} b := &Node{data: 3} head.next = a a.next = b // 遍历链表并输出每个节点的值 printList(head) }
4. Stack
The stack is a last-in-first-out (LIFO) data structure, which is often used in programming to implement Scenarios such as expression evaluation, function calling and recursion. The stack can insert and delete data through operations such as push (into the stack) and pop (out of the stack).
The sample code is as follows:
package main import "fmt" type Stack struct { nums []int } func (s *Stack) Push(num int) { s.nums = append(s.nums, num) } func (s *Stack) Pop() int { if len(s.nums) == 0 { return -1 } num := s.nums[len(s.nums)-1] s.nums = s.nums[:len(s.nums)-1] return num } func main() { // 创建栈并进行操作 stack := Stack{} stack.Push(1) stack.Push(2) stack.Push(3) fmt.Println(stack.Pop()) fmt.Println(stack.Pop()) fmt.Println(stack.Pop()) }
5. Queue (Queue)
Queue is a first-in-first-out (FIFO) data structure, which is often used in programming to implement Scenarios such as task scheduling, message delivery and caching. Queues can insert and delete data through operations such as enqueue and dequeue.
The sample code is as follows:
package main import "fmt" type Queue struct { nums []int } func (q *Queue) Enqueue(num int) { q.nums = append(q.nums, num) } func (q *Queue) Dequeue() int { if len(q.nums) == 0 { return -1 } num := q.nums[0] q.nums = q.nums[1:] return num } func main() { // 创建队列并进行操作 queue := Queue{} queue.Enqueue(1) queue.Enqueue(2) queue.Enqueue(3) fmt.Println(queue.Dequeue()) fmt.Println(queue.Dequeue()) fmt.Println(queue.Dequeue()) }
The above are common data structures and their applications in the Go language. Through specific code examples, we can understand that different data structures are suitable for different scenarios. In actual programming, we can choose appropriate data structures for development according to specific needs to improve the performance and efficiency of the program.
The above is the detailed content of Master the application of data structures in Go language. For more information, please follow other related articles on the PHP Chinese website!
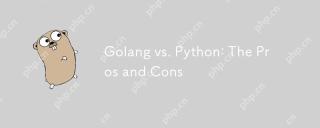
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
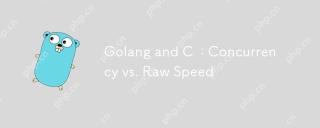
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
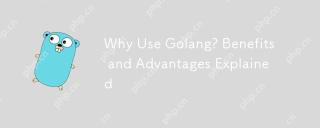
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
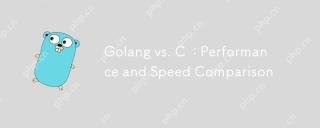
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
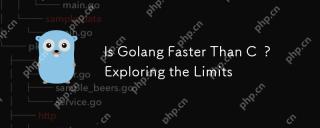
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
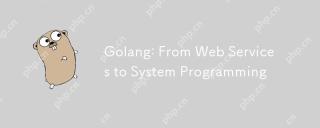
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
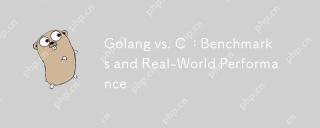
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
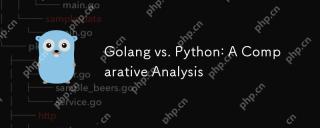
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.