Web Storage is a very important feature introduced by HTML5. It can store data locally on the client, similar to HTML4 cookies, but its functions are much more powerful than cookies. The cookie size is limited to 4KB. Web Storage officially recommends that each Website 5MB.
Web Storage is divided into two types :
sessionStorage
localStorage
It can be clearly seen from the literal meaning that sessionStorage saves the data in the session and the browser is closed. That’s gone; localStorage always saves data locally on the client;
Whether it is sessionStorage or localStorage, the APIs that can be used are the same, and the commonly used ones are as follows (taking localStorage as an example):
Save data: localStorage.setItem(key,value);
Read data: localStorage.getItem(key);
Delete single data: localStorage.removeItem(key);
Delete all data: localStorage.clear ();
Get the key of an index: localStorage.key(index);
As above, both key and value must be strings. In other words, the web Storage API can only operate on strings.
Next, we develop a simple address book applet through Web Storage to demonstrate the use of relevant APIs; we want to implement the following functions:
Enter contacts. Contacts have two fields: name and mobile phone number. , use the mobile phone number as the key to store it in localStorage;
Find the owner according to the mobile phone number;
List all currently saved contact information;
First, prepare a simple HTML page, As follows :
Web Storage of HTML5 Local Storage
The interface is shown as follows:
To save contacts, you only need to simply implement the following JS method:
//Save data
function save(){
var mobilephone = document .getElementById("mobilephone").value;
var user_name = document.getElementById("user_name").value;
localStorage.setItem(mobilephone,user_name);
}
To find the owner, implement the following JS method:
//Find data
function find(){
var search_phone = document.getElementById("search_phone").value;
var name = localStorage.getItem( search_phone);
var find_result = document.getElementById("find_result");
find_result.innerHTML = search_phone "The owner is: " name;
}
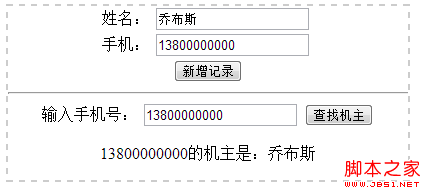
To display all saved contact information, you need to use the localStorage.key(index) method, as follows:
//Extract all objects stored in localStorage and display them on the interface
function loadAll(){
var list = document.getElementById("list");
if(localStorage.length>0){
var result = "
";
result = "Name | < td>Mobile phone number
";
for(var i=0;ivar mobilephone = localStorage.key(i);
var name = localStorage.getItem(mobilephone);
result = "" name " | " mobilephone " |
";
}
result = "
";
list.innerHTML = result;
}else{
list.innerHTML = "The data is currently empty, start adding now Contact Bar";
}
}
The effect is as follows:
Problem: The above demo only has 2 fields, name and mobile phone number , if you want to store richer contact information, such as company name, home address, etc., how to achieve it? Doesn't Web Storage only handle strings? At this time, you can use JSON's stringify() method to convert complex objects into strings and store them in Web Storage; when reading from Web Storage, you can use JSON's parse() method to convert them into JSON objects;
The following is a simple demonstration of adding the JS code for saving contacts with company attributes:
//Save data
function save(){
var contact = new Object;
contact.user_name = document.getElementById("user_name"). value;
contact.mobilephone = document.getElementById("mobilephone").value;
contact.company = document.getElementById("company").value;
var str = JSON.stringify(contact) ;
localStorage.setItem(contact.mobilephone,str);
loadAll();
}
//Extract all objects stored in localStorage and display them on the interface
function loadAll(){
var list = document.getElementById("list");
if(localStorage.length>0){
var result = "
" ;
result = "Name | Mobile phone | Company |
";
for(var i=0;ivar mobilephone = localStorage.key(i);
var str = localStorage.getItem(mobilephone);
var contact = JSON .parse(str);
result = "" contact.user_name " | " contact.mobilephone " | " contact .company " |
";
}
result = "
";
list.innerHTML = result;
}else{
list.innerHTML = "The data is currently empty, start adding contacts quickly";
}
}
The effect is as follows:
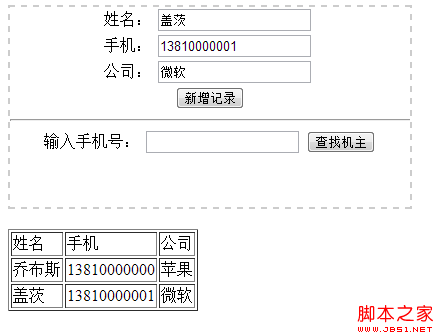