


An analysis of the transitive closure algorithm: Depth-first search versus breadth-first search
Analysis of transitive closure algorithm: depth-first search vs breadth-first search
Introduction:
The transitive closure algorithm is an important algorithm in graph theory. Use Transitive closure for constructing relationship graphs. When implementing the transitive closure algorithm, two common search strategies are depth-first search (DFS) and breadth-first search (BFS). This article will introduce these two search strategies in detail and analyze their application in the transitive closure algorithm through specific code examples.
1. Depth-first search (DFS):
Depth-first search is a search strategy that first explores deep nodes and then backtracks to shallower nodes. In the transitive closure algorithm, we can use DFS to construct the transitive closure of the relationship graph. Below we use the following example code to illustrate the application of DFS in the transitive closure algorithm:
# 传递闭包算法-深度优先搜索 def dfs(graph, start, visited): visited[start] = True for neighbor in graph[start]: if not visited[neighbor]: dfs(graph, neighbor, visited) def transitive_closure_dfs(graph): num_nodes = len(graph) closure_table = [[0] * num_nodes for _ in range(num_nodes)] for node in range(num_nodes): visited = [False] * num_nodes dfs(graph, node, visited) for i in range(num_nodes): if visited[i]: closure_table[node][i] = 1 return closure_table
In the above code, we first define the DFS function for depth-first search. Next, we use DFS in the transitive_closure_dfs function to build a transitive closure. Specifically, we use a two-dimensional matrix closure_table to record the transitive closure relationship. After each DFS, we use the node corresponding to True in the visited array as the direct successor node of the original node, and mark the corresponding position as 1 in closure_table.
2. Breadth-first search (BFS):
Breadth-first search is a search strategy that first explores adjacent nodes and then expands outward layer by layer. In the transitive closure algorithm, we can also use BFS to construct the transitive closure of the relationship graph. Below we use the following example code to illustrate the application of BFS in the transitive closure algorithm:
from collections import deque # 传递闭包算法-广度优先搜索 def bfs(graph, start, visited): queue = deque([start]) visited[start] = True while queue: node = queue.popleft() for neighbor in graph[node]: if not visited[neighbor]: visited[neighbor] = True queue.append(neighbor) def transitive_closure_bfs(graph): num_nodes = len(graph) closure_table = [[0] * num_nodes for _ in range(num_nodes)] for node in range(num_nodes): visited = [False] * num_nodes bfs(graph, node, visited) for i in range(num_nodes): if visited[i]: closure_table[node][i] = 1 return closure_table
In the above code, we first define the BFS function for breadth-first search. Different from DFS, we use a queue to save nodes to be explored, and each time a node is explored, all its neighboring nodes that have not yet been visited are added to the queue. Similarly, BFS is used to build a transitive closure in the transitive_closure_bfs function. Specifically, we also use closure_table to record the transitive closure relationship, and mark the corresponding position as 1 based on the value of the visited array.
Conclusion:
Depth-first search and breadth-first search are two search strategies commonly used in transitive closure algorithms. Although they differ in implementation, they all play an important role in building transitive closures. This article introduces in detail the methods and steps of implementing the transitive closure algorithm through DFS and BFS through specific code examples. I hope this article can help readers better understand the application of depth-first search and breadth-first search in transitive closure algorithms.
The above is the detailed content of An analysis of the transitive closure algorithm: Depth-first search versus breadth-first search. For more information, please follow other related articles on the PHP Chinese website!
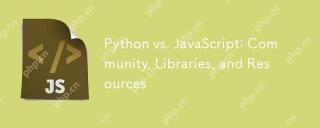
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
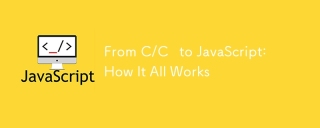
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
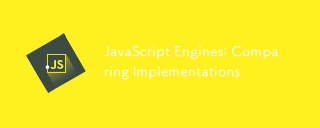
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
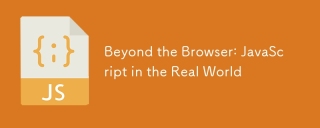
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
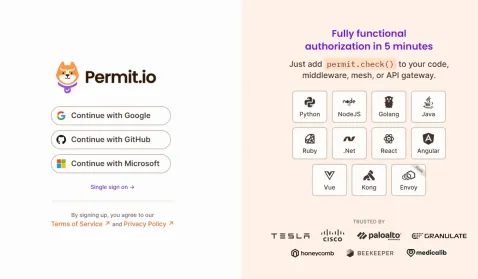
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
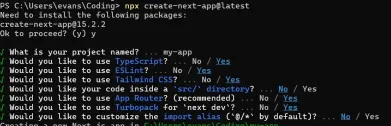
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
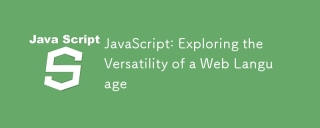
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
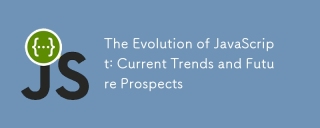
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
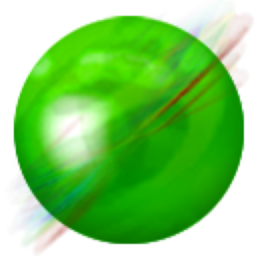
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
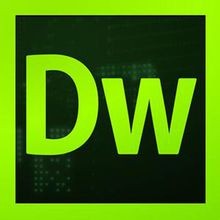
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version