The reason why prototypes and prototype chains exist is to achieve inheritance and sharing of object properties in the JavaScript language. In JavaScript, everything is an object, including functions. Every object has a property called a prototype that points to another object, which is called a prototype object. Objects can inherit properties and methods from prototype objects.
The advantage of implementing shared properties and methods through prototypes is to save memory. Consider an object A, which has some properties and methods, then create object B and make it inherit from object A. If the properties and methods are copied directly to object B, then each instance of B will have the same properties and methods, causing a waste of memory. Through the prototype, all B instances can share the properties and methods of the A object, and only need to save a copy of the prototype object.
The prototype chain refers to the mechanism by which objects are linked together through prototypes. If a property or method of an object cannot be found on the object itself, JavaScript will continue searching along the prototype chain until it is found or not found. This mechanism allows objects to inherit and share properties and methods, realizing the inheritance relationship between objects.
The following is a specific code example to illustrate the concept of prototype and prototype chain:
// 通过构造函数创建一个对象 function Animal(name) { this.name = name; } // 在Animal的原型对象上添加一个方法 Animal.prototype.sayHello = function() { console.log("Hello, I'm " + this.name); }; // 创建一个Animal实例 var animal = new Animal("Tom"); animal.sayHello(); // 输出: Hello, I'm Tom // 创建另一个对象,它继承自Animal function Cat(name, color) { Animal.call(this, name); // 调用Animal的构造函数 this.color = color; } // 使用Object.create方法将Cat的原型对象指向Animal的原型对象 Cat.prototype = Object.create(Animal.prototype); Cat.prototype.constructor = Cat; // 在Cat的原型对象上添加一个方法 Cat.prototype.sayMeow = function() { console.log("Meow, I'm " + this.name); }; // 创建一个Cat实例 var cat = new Cat("Kitty", "White"); cat.sayHello(); // 输出: Hello, I'm Kitty cat.sayMeow(); // 输出: Meow, I'm Kitty
In the above code, Animal is a constructor, which has a prototype object prototype. Cat inherits from Animal, and points Cat's prototype object to Animal's prototype object by calling the Object.create method. In this way, the Cat instance will inherit the properties and methods of Animal, and can add new methods on its own prototype object.
Through the mechanism of prototype and prototype chain, JavaScript realizes inheritance and attribute sharing between objects, improving the efficiency and maintainability of the program.
The above is the detailed content of What is the purpose of prototypes and prototype chains?. For more information, please follow other related articles on the PHP Chinese website!
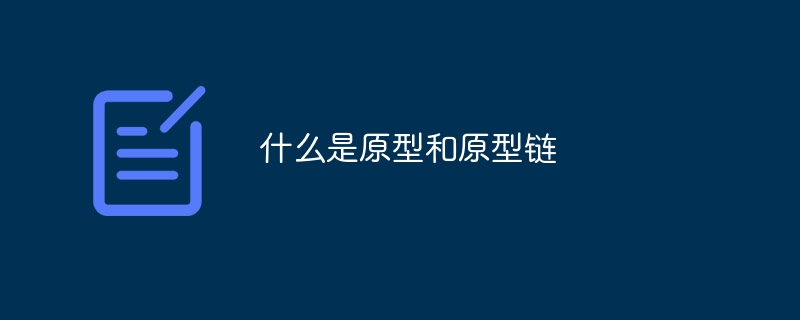
原型,js中的一个对象,用于定义其他对象的属性和方法,每个构造函数都有一个prototype属性,这个属性是一个指针,指向一个原型对象,当创建新对象时,这个新对象会从其构造函数的prototype属性继承属性和方法。原型链,当试图访问一个对象的属性时,js会首先检查这个对象是否有这个属性,如果没有,那么js会转向这个对象的原型,如果原型对象也没有这个属性,会继续查找原型的原型。
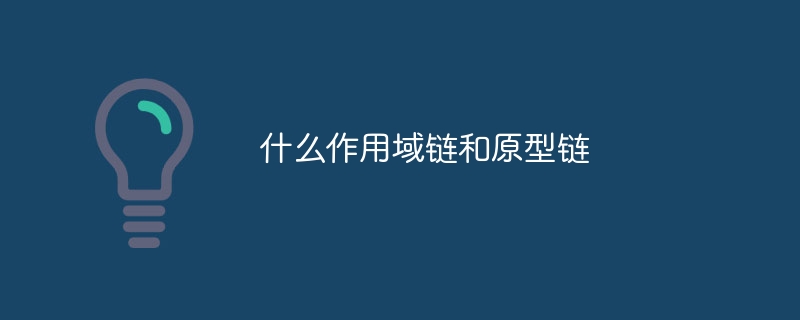
作用域链和原型链是JavaScript中两个重要的概念,分别对应着作用域和继承两个核心特性:1、作用域链是JavaScript中用来管理变量访问和作用域的机制,其形成是由函数创建时所处的执行上下文环境和词法作用域决定的;2、原型链是JavaScript中实现继承的机制,基于对象之间的原型关系,当访问对象的属性或方法时,如果该对象本身没有定义,会沿着原型链向上查找。
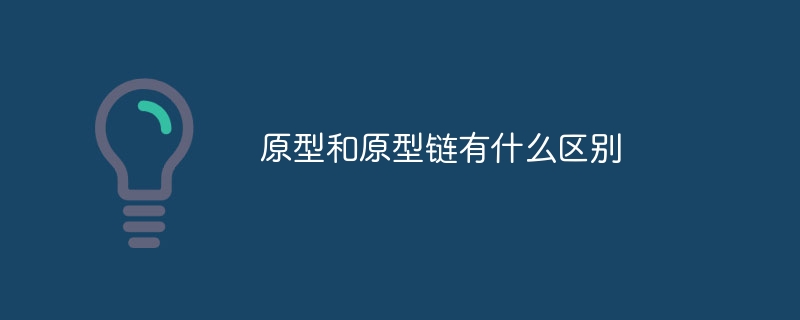
原型和原型链的区别是:1、原型是每个对象都具有的属性,包含了一些共享的属性和方法,用于实现对象之间的属性和方法的共享和继承,而原型链是一种通过对象之间的原型关系来实现继承的机制,定义了对象之间的继承关系,使得对象可以共享原型对象的属性和方法;2、原型的作用是定义对象的共享属性和方法,使得多个对象可以共享同一个原型对象的属性和方法,而原型链的作用是实现对象之间的继承关系等等。
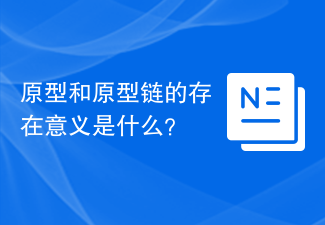
原型和原型链存在的原因是为了实现JavaScript语言中的继承和对象属性的共享。在JavaScript中,一切皆为对象,包括函数。每个对象都有一个属性,称为原型(prototype),它指向另一个对象,该对象被称为原型对象。对象可以从原型对象中继承属性和方法。通过原型实现共享属性和方法的好处是节省内存。考虑一个对象A,它有一些属性和方法,然后创建对象B并使
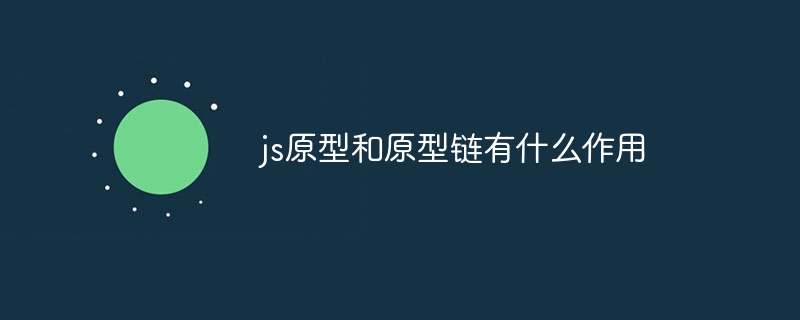
js原型和原型链的作用是实现对象的继承,节省内存空间,并提高代码的性能和可维护性。详细介绍:1、实现对象的继承,原型和原型链允许创建一个对象,并使其继承另一个对象的属性和方法,当创建一个新的对象时,可以将其原型指向另一个对象,这样新对象就可以访问到原型对象上的属性和方法;2、节省内存和提高性能,在JavaScript中,每个对象都有一个原型,通过原型链,对象可以共享原型上等等。
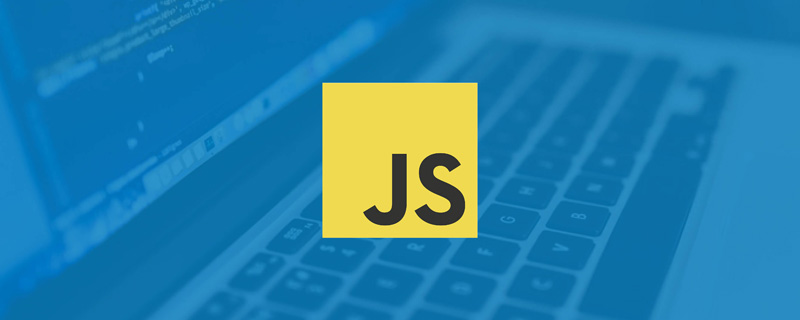
原型链,简单理解就是原型组成的链。当访问一个对象的某个属性时,会先在这个对象本身属性上查找,如果没有找到,则会去它的__proto__隐式原型上查找,即它的构造函数的prototype,如果还没有找到就会再在构造函数的prototype的__proto__中查找,这样一层一层向上查找就会形成一个链式结构,被称为原型链。
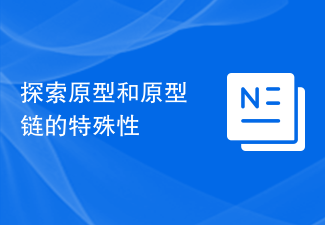
原型和原型链的独特之处探究在JavaScript中,原型(prototype)和原型链(prototypechain)是非常重要的概念。理解原型和原型链的独特之处可以帮助我们更好地理解JavaScript中的继承和对象创建。原型是JavaScript中每个对象都拥有的一个属性,它指向一个其他对象,用于共享属性和方法。每个JavaScript对象都有一个原型
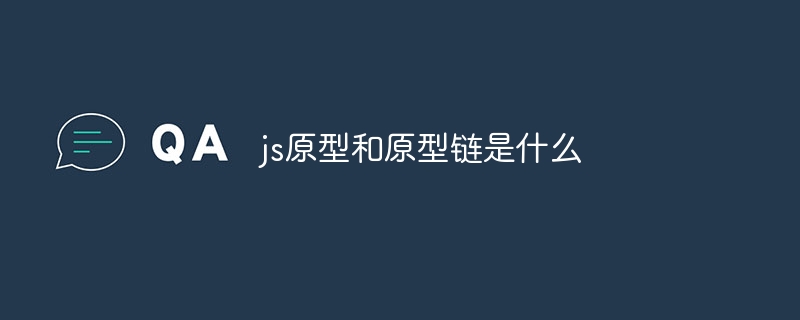
js原型和原型链是:1、原型,所有的函数默认都有一个“prototype”这样公有且不可枚举的属性,它会指向另一个对象,这个对象就是原型。2、原型链,当访问对象的属性或方法时,首先对象会从自身去找,找不到就会往原型中去找,也就是它构造函数的“prototype”中,如果原型中找不到,即构造函数中也没有该属性,就会往原型后面的原型上去找,这样就形成了链式的结构,称为原型链。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
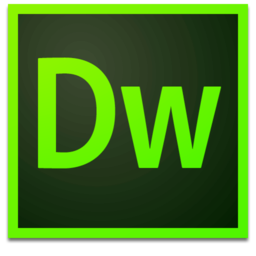
Dreamweaver Mac version
Visual web development tools
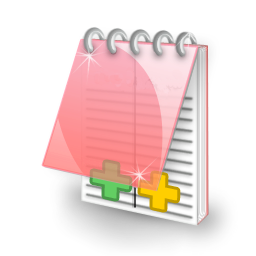
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.