


Concepts and applications of prototypes and prototype chains in programming
The concept of prototype and prototype chain and its application in programming
In programming, prototype and prototype chain are a very important and basic concept in JavaScript. They are widely used in JavaScript object-oriented programming to implement object inheritance and attribute sharing. This article will introduce the concepts of prototypes and prototype chains and demonstrate their application in programming through concrete code examples.
1. The concept of prototype
In JavaScript, each object has a link to another object, and this link is the prototype. A prototype is an ordinary object that contains some shared properties and methods. An object can access properties and methods that do not belong to itself through its prototype.
The following is a sample code that demonstrates how to create a prototype of an object:
// 创建一个原型对象 var prototypeObject = { speak: function() { console.log("Hello!"); } }; // 创建一个实例对象 var instanceObject = Object.create(prototypeObject); // 调用原型中的方法 instanceObject.speak(); // 输出: Hello!
In the above code, we first create a prototype object prototypeObject
, which contains A speak
method. Next, we use the Object.create()
method to create an instance object instanceObject
and set prototypeObject
to the prototype of instanceObject
. Finally, we access the speak
method in the prototype through instanceObject
.
2. The concept of prototype chain
Each object has a prototype object, and the prototype object itself can also have a prototype. This forms a prototype chain through which properties and methods can be inherited. When we try to access the properties or methods of an object, if the object itself does not find the corresponding properties or methods, it will search up the prototype chain until it finds or reaches the top of the prototype chain (usually Object.prototype
)until.
The following is a sample code that demonstrates the inheritance relationship of the prototype chain:
// 创建一个原型对象 var parent = { speak: function() { console.log("Hello from parent!"); } }; // 创建一个子对象,并将parent设置为其原型 var child = Object.create(parent); // 调用原型中的方法 child.speak(); // 输出: Hello from parent!
In the above code, we create a prototype object parent
, which contains a speak
method. Then, we use the Object.create()
method to create a child object child
and set parent
as the prototype of child
. In this way, the child
object inherits the speak
method in the parent
object through the prototype chain.
3. Application in programming
Prototypes and prototype chains are widely used in programming. Through prototypes, we can realize the inheritance relationship between objects, reduce repeated code, and improve code reusability. Through the prototype chain, we can share properties and methods, reduce memory consumption, and improve program execution efficiency.
The following is a sample code that demonstrates the application of prototype and prototype chain:
// 创建一个Animal对象 function Animal(name) { this.name = name; } // 通过原型添加方法 Animal.prototype.speak = function() { console.log("Hello, my name is " + this.name); }; // 创建一个Dog对象,并继承Animal对象 function Dog(name) { Animal.call(this, name); } // 设置Dog对象的原型为Animal对象的实例 Dog.prototype = Object.create(Animal.prototype); // 通过原型添加方法 Dog.prototype.bark = function() { console.log("Woof!"); }; // 创建一个Dog对象实例 var dog = new Dog("Tom"); // 调用继承自Animal的方法 dog.speak(); // 输出: Hello, my name is Tom // 调用自身定义的方法 dog.bark(); // 输出: Woof!
In the above code, we first define an Animal
object and assign it to Added speak
method. Next, we defined a Dog
object and inherited the properties in the Animal
object through the Animal.call()
method. Then, we set Dog.prototype
to an instance of Animal.prototype
, realizing the inheritance relationship of the prototype chain. Finally, we added the bark
method to the prototype of the Dog
object. Through this design, we can inherit the methods of the Animal
object when creating a Dog
object instance, and define our own methods in the Dog
object.
Summary:
Prototype and prototype chain are an important concept in JavaScript, and they are widely used in object-oriented programming. Through prototypes, we can realize the inheritance relationship between objects. Through the prototype chain, we can share properties and methods. In programming, rational use of prototypes and prototype chains can reduce code redundancy and improve code reusability and execution efficiency.
The above is the detailed content of Concepts and applications of prototypes and prototype chains in programming. For more information, please follow other related articles on the PHP Chinese website!
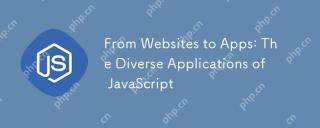
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
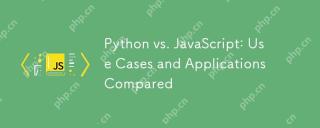
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
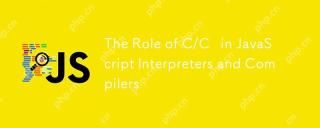
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
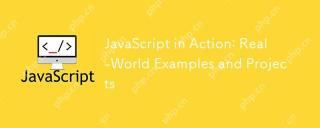
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
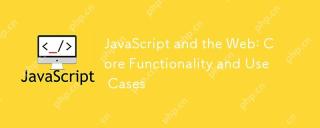
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
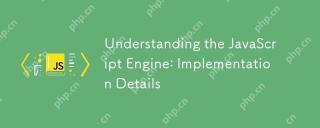
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
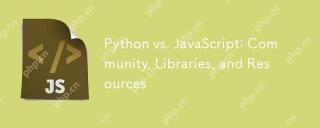
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
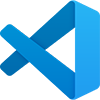
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor