Basic syntax and usage of Java interface classes
Introduction:
In the Java programming language, the interface (interface) is a special class that is used It defines a set of related methods but does not provide implementation details. An interface can be thought of as a contract or contract that stipulates specifications that other classes must adhere to. Interface classes play the role of defining behavior, allowing classes to implement multiple interfaces, thereby achieving multiple inheritance. This article will introduce the basic syntax and usage of Java interface classes and provide specific code examples.
1. Definition of interface class
The interface class is declared using the keyword interface. The specific syntax is as follows:
接口修饰符 interface 接口名 { // 声明接口中的方法 }
Example:
public interface Drawable { void draw(); }
The above code defines a name It is an interface class of Drawable, which only declares a draw method with no return value and no parameters.
2. Implementation of interface class
Other classes can implement the methods defined in the interface class by using the keyword implements. The specific syntax is as follows:
class 类名 implements 接口名 { // 实现接口中的方法 }
Example:
public class Circle implements Drawable { public void draw() { // 实现绘制圆形的代码 } }
The above code defines a class named Circle, which implements the Drawable interface and implements the draw method.
3. Multiple implementations of interface classes
In Java, a class can implement multiple interfaces, separated by commas. The specific syntax is as follows:
class 类名 implements 接口名1, 接口名2, ... { // 实现接口中的方法 }
Example:
public class Square implements Drawable, Resizable { public void draw() { // 实现绘制正方形的代码 } public void resize() { // 实现调整正方形大小的代码 } }
The above code defines a class named Square, which implements two interfaces: Drawable and Resizable, and implements the draw and resize methods respectively.
4. Inheritance of interface classes
Interface classes can also inherit other interface classes by using the keyword extends. The specific syntax is as follows:
接口修饰符 interface 子接口名 extends 父接口名 { // 声明子接口中的方法 }
Example:
public interface Resizable extends Drawable { void resize(); }
The above code defines an interface class named Resizable, which inherits the Drawable interface and declares a resize method.
Summary:
Interface class is a powerful grammatical feature in Java that can be implemented by classes and provides a convention and standard method to make the code more modular and extensible. Through interface classes, polymorphism can be achieved, making the program more flexible. In actual development, we should subdivide relevant methods as needed and define them as interfaces to improve the maintainability and readability of the code.
The above is the basic syntax and usage of Java interface classes. I hope this article can help you understand the concepts and usage of interface classes.
The above is the detailed content of Basic syntax and usage of Java interfaces. For more information, please follow other related articles on the PHP Chinese website!
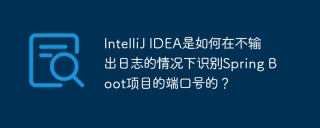
Start Spring using IntelliJIDEAUltimate version...
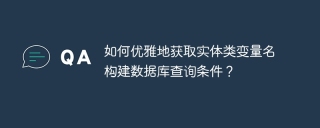
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
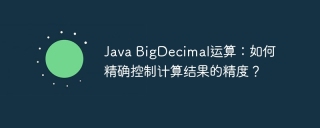
Java...
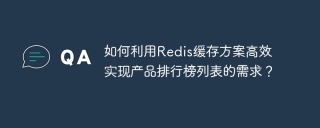
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
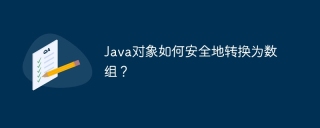
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
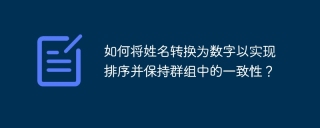
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
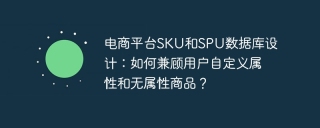
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
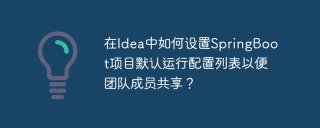
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
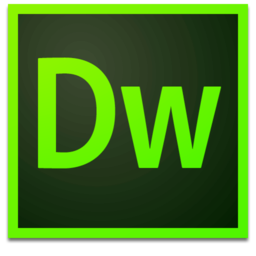
Dreamweaver Mac version
Visual web development tools
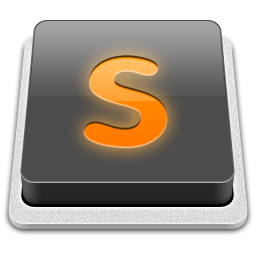
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
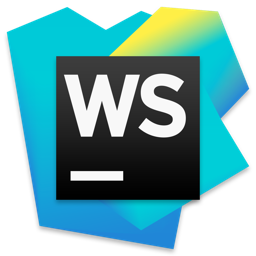
WebStorm Mac version
Useful JavaScript development tools