How to correctly define and initialize a Java array requires specific code examples
In Java programming, an array is a commonly used data structure used to store the same type of element. Properly defining and initializing arrays is key to writing efficient and reliable code. This article details how to correctly define and initialize Java arrays and provides specific code examples.
-
Define array
The syntax for defining an array in Java is as follows:dataType[] arrayName; 或 dataType arrayName[];
Among them, dataType represents the data type of the elements in the array, and arrayName is the name of the array.
For example, if we want to define an array containing 5 integers, we can use the following code:
int[] numbers; 或 int numbers[];
At this point, we have successfully defined an array of integers.
- Initialize the array
After defining the array, you need to initialize the array, that is, assign values to the array elements. There are two ways to initialize arrays: static initialization and dynamic initialization.
2.1 Static initialization
Static initialization is to directly assign a value to the array while defining the array. The syntax is as follows:
dataType[] arrayName = {value1, value2, value3, ...};
Among them, value1, value2, value3 are the elements in the array Value, which can be of any data type. Note that the number of elements in curly braces {} during static initialization determines the length of the array.
The following is a sample code for static initialization:
int[] numbers = {1, 2, 3, 4, 5};
The above code defines an integer array numbers and assigns it values {1, 2, 3, 4, 5}.
2.2 Dynamic initialization
Dynamic initialization is to assign values to the array elements one by one after defining the array. The syntax is as follows:
dataType[] arrayName = new dataType[length];
Among them, length represents the length of the array, that is, the number of elements in the array. number.
The following is a sample code for dynamic initialization:
int[] numbers = new int[5]; numbers[0] = 1; numbers[1] = 2; numbers[2] = 3; numbers[3] = 4; numbers[4] = 5;
The above code first defines an integer array numbers with a length of 5, and then assigns values to the array elements one by one. Note that array subscripts start at 0, so numbers[0] represents the first element in the array.
- Definition and initialization of multi-dimensional arrays
In addition to one-dimensional arrays, Java also supports multi-dimensional arrays. A multidimensional array can be thought of as an array of arrays. The methods of defining and initializing multidimensional arrays are similar to those of one-dimensional arrays.
The following is a sample code for the definition and initialization of a two-dimensional array:
int[][] matrix = {{1, 2}, {3, 4}, {5, 6}};
The above code defines a two-dimensional integer array matrix containing 3 rows and 2 columns, and assigns a value to it .
- Traverse the array
In actual programming, we often need to traverse the array to operate on the array elements. Java provides a variety of ways to traverse arrays, such as using for loops, foreach loops, etc.
The following is a sample code that uses a for loop to traverse a one-dimensional array:
int[] numbers = {1, 2, 3, 4, 5}; for (int i = 0; i < numbers.length; i++) { System.out.println(numbers[i]); }
The above code traverses the integer array numbers through a for loop and outputs the array elements in sequence.
- Summary
This article introduces how to correctly define and initialize arrays in Java programming and provides specific code examples. When using an array, please note that the subscript of the array starts from 0, and the length of the array is fixed and cannot be changed. By using arrays rationally, we can store and process large amounts of data more efficiently and improve program execution efficiency. I hope this article will help everyone understand and use Java arrays.
The above is the detailed content of Correct way to define and initialize Java arrays. For more information, please follow other related articles on the PHP Chinese website!
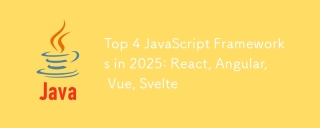
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
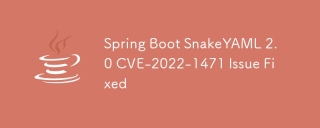
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
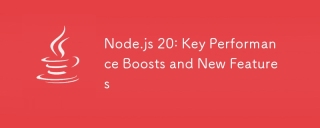
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
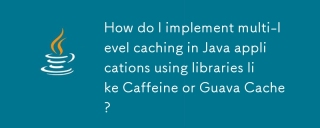
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
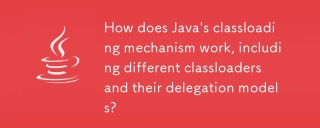
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
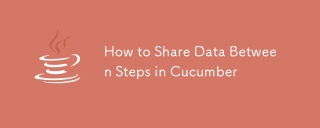
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
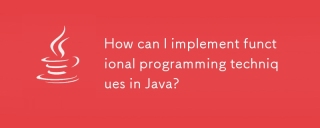
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
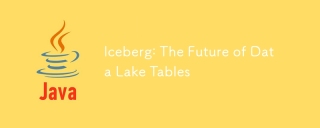
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
