How to Share Data Between Steps in Cucumber
There are several ways to share data between steps in Cucumber, each with its own advantages and disadvantages. The best approach depends on the complexity of your application and the nature of the data being shared.
1. Using Scenario Context: Cucumber provides a built-in mechanism for managing scenario context. This is arguably the cleanest and most maintainable method for passing data between steps within a single scenario. You can use the World
object (or a custom object injected into the World
) to store and retrieve data. This approach is particularly suitable for data that is relevant only to the current scenario.
# Example using Ruby and Cucumber # features/step_definitions/my_steps.rb Before do |scenario| @scenario_context = {} end Given("I have a username of {string}") do |username| @scenario_context[:username] = username end When("I login") do username = @scenario_context[:username] # ... use username to perform login ... end Then("I should see a welcome message") do # ... verify welcome message based on previous steps ... end
2. Using global variables (generally discouraged): While you can use global variables, this is generally discouraged due to the risk of unintended side effects and reduced code clarity. Global variables make it difficult to track the origin and usage of data, leading to maintenance nightmares as your test suite grows.
3. Passing data as arguments: This is a straightforward method for passing data directly between steps. However, it can lead to long step definitions with many arguments if you're passing multiple pieces of data.
# Example using Ruby and Cucumber Given("I have a username {string} and password {string}") do |username, password| # ... use username and password ... end
4. Using a data structure (e.g., Hash, Array): For more complex scenarios, you can create a data structure (like a hash or array) to store multiple pieces of data and pass it between steps. This approach is more organized than passing individual arguments but still requires careful management to avoid clutter.
What are the best practices for managing state and data across different Cucumber steps?
Best practices for managing state and data across Cucumber steps focus on maintainability, readability, and testability.
- Keep it concise: Avoid storing excessive amounts of data in the scenario context. Only store data that's absolutely necessary for subsequent steps. If data can be easily derived or retrieved elsewhere, don't store it unnecessarily.
- Use descriptive variable names: Clearly name variables to reflect the data they contain. This improves code readability and makes it easier to understand the flow of data.
- Avoid mutable state: Whenever possible, prefer immutable data structures. This reduces the risk of unintended modifications and makes debugging easier.
- Use a consistent approach: Choose a data sharing method (e.g., scenario context) and stick with it consistently throughout your project. Inconsistent approaches make the code harder to understand and maintain.
- Modularize your steps: Break down complex scenarios into smaller, more manageable steps. This reduces the amount of data that needs to be passed between steps and improves the overall structure of your tests.
- Test data separation: Separate your test data from your step definitions. This makes it easier to manage and update test data without modifying your step definitions. Consider using external files or databases to manage test data.
How can I avoid code duplication when passing data between Cucumber steps?
Code duplication when passing data can often stem from similar steps needing the same data. To avoid this:
- Refactor common logic: If multiple steps perform similar operations on the same data, extract this logic into helper methods or classes. This reduces redundancy and improves code maintainability.
- Use Page Objects (or similar patterns): Page Objects encapsulate interactions with specific parts of your application. This allows you to centralize data access and reduce duplication in your step definitions.
- Data tables: For scenarios involving multiple sets of input data, use Cucumber's data table feature to provide the data in a structured format. This reduces code duplication by avoiding the need to hardcode the data in multiple steps.
- Custom helper methods: Create custom helper methods to handle common data transformations or manipulations. This makes your step definitions more concise and easier to read.
What are the common pitfalls to avoid when sharing data between Cucumber steps, and how can I overcome them?
Several common pitfalls can arise when sharing data between Cucumber steps:
- Over-reliance on global state: Using global variables or excessively large scenario contexts leads to tightly coupled, difficult-to-maintain tests. Solution: Use the scenario context sparingly and favor passing data directly as arguments or using Page Objects where appropriate.
- Difficult debugging: Tracking data flow across multiple steps can be challenging. Solution: Use a debugger or logging to track data values and identify issues. Well-structured code and descriptive variable names also help.
- Fragile tests: Tests that depend heavily on the order of steps or the precise state of the application are prone to breakage. Solution: Design tests that are independent and robust to changes in the application's state.
- Data inconsistency: Data may be modified unexpectedly in one step, leading to errors in subsequent steps. Solution: Favor immutable data structures and ensure data modifications are carefully controlled and tracked.
- Unclear data flow: The flow of data between steps may not be immediately apparent, leading to confusion and errors. Solution: Use clear and descriptive variable names, comments, and modularize your steps to improve code readability. Consider using diagrams to visualize data flow.
By following these best practices and avoiding these pitfalls, you can create robust, maintainable, and reliable Cucumber tests that effectively share data between steps.
The above is the detailed content of How to Share Data Between Steps in Cucumber. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
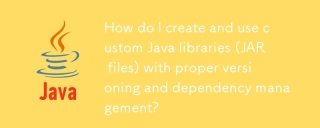
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
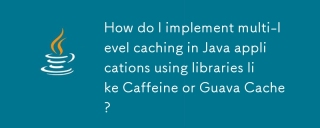
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
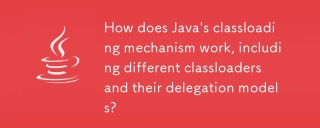
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
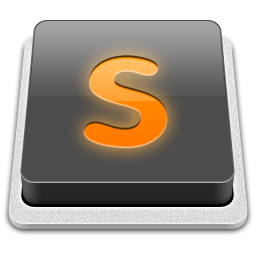
SublimeText3 Mac version
God-level code editing software (SublimeText3)