Analysis of the role and importance of cookies in Java
Guide to using cookies in Java: Why is it so important?
Introduction:
Cookie is a technology widely used in Web development, used to transfer and store user data between the client and the server. It helps the server identify users and provide personalized services for each user. For Java developers, it is very important to understand the usage and principles of cookies. This article will introduce the basic concepts and usage of Cookies, and provide some specific Java code examples to help readers better understand and apply Cookies.
1. Basic concepts and principles of Cookie
1.1 What is Cookie?
Cookie is a small text file set by the server in the HTTP response header, which is stored on the user's browser. It can track and store user information, such as the user's login status or preferences.
1.2 How Cookie Works
When a user visits a website, the server sets the Cookie by adding the Set-Cookie field in the HTTP response header. The browser will save the cookie and automatically send it to the server in subsequent requests. The server can determine the user's identity and request based on the information in the cookie.
2. How to use Cookie
2.1 Setting Cookie
In Java, you can use the addCookie method of HttpServletResponse to set Cookie. The following is an example:
Cookie cookie = new Cookie("username", "John"); response.addCookie(cookie);
2.2 Get Cookie
In Java, you can use the getCookies method of HttpServletRequest to obtain all Cookies sent by the client. The following is an example:
Cookie[] cookies = request.getCookies(); if (cookies != null) { for (Cookie cookie : cookies) { String name = cookie.getName(); String value = cookie.getValue(); // 处理Cookie的逻辑 } }
2.3 Delete Cookie
In Java, you can delete cookies by setting the maximum cookie lifetime to 0. The following is an example:
Cookie cookie = new Cookie("username", ""); cookie.setMaxAge(0); response.addCookie(cookie);
3. Common application scenarios of cookies
3.1 User login authentication
By setting a cookie containing user information after the user successfully logs in, the user can automatically log in. When the user visits the website again, the server can determine the user's identity based on the information in the cookie and automatically log in the user.
3.2 Remembering user preferences
Remembering user preferences can be achieved by setting a cookie containing user preferences after the user sets preferences. When the user visits the website again, the server can load the user's preferences based on the information in the cookie.
3.3 Shopping cart
By setting a cookie containing product information after the user adds products to the shopping cart, the user's shopping cart contents can be remembered. When the user visits the website again, the server can load the user's shopping cart based on the information in the cookie.
4. Cookie security considerations
4.1 Cookie security issues
If cookies are maliciously tampered with, it may lead to user privacy leaks or security issues. In order to protect the security of cookies, you can use the HTTPS protocol to transmit cookies, and perform operations such as encrypting and signing cookies.
4.2 Cookie security settings
In Java, you can use the setSecure method of Cookie to set the Cookie to only be transmitted under an HTTPS connection. You can use the setHttpOnly method of Cookie to set the Cookie so that it can only be accessed by the server and not by JavaScript.
Conclusion:
Cookie is a very important technology in Java Web development. Mastering the usage and principles of cookies can help developers implement functions such as user authentication and personalized services. By setting cookie security settings, you can improve cookie security and prevent malicious attacks. I hope that the guidelines for using cookies in Java introduced in this article will be helpful to readers.
The above is the detailed content of Analysis of the role and importance of cookies in Java. For more information, please follow other related articles on the PHP Chinese website!
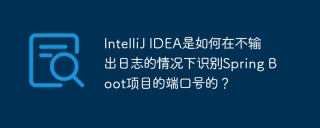
Start Spring using IntelliJIDEAUltimate version...
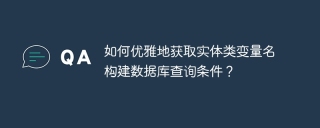
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
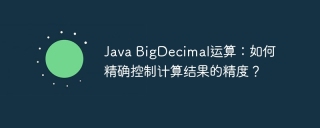
Java...
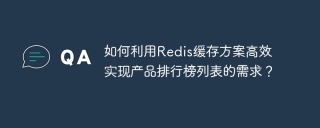
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
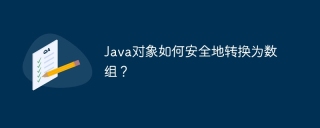
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
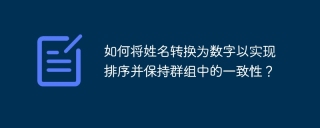
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
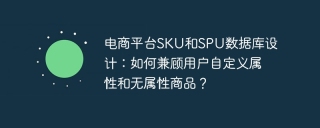
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
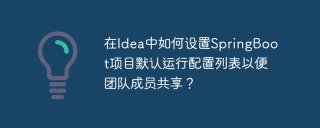
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
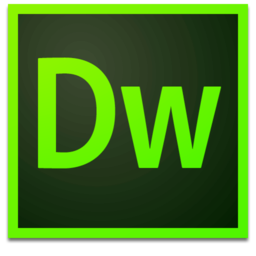
Dreamweaver Mac version
Visual web development tools
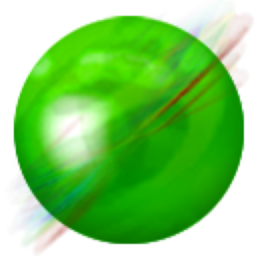
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software