


Optimizing Java Email Sending: Tips and Practical Methods to Improve Email Transmission Efficiency
Java email sending tips and best practices: Methods to improve email delivery efficiency require specific code examples
Abstract:
With the popularity of the Internet and information Communication is becoming more and more frequent, and email is widely used as a very important communication tool. In Java development, sending emails is a common task. This article will introduce some Java email sending tips and best practices, aiming to improve email delivery efficiency and optimize code implementation.
1. Use JavaMail API to send emails
JavaMail API is a Java open source framework specifically used to send and receive emails. It provides some core classes and interfaces to simplify the creation, sending and management of emails. process. The following is a sample code that uses the JavaMail API to send emails:
import java.util.*; import javax.mail.*; import javax.mail.internet.*; public class EmailSender { public static void main(String[] args) { // 配置SMTP服务器信息 Properties props = new Properties(); props.put("mail.smtp.auth", "true"); props.put("mail.smtp.host", "smtp.example.com"); props.put("mail.smtp.port", "587"); // 创建会话 Session session = Session.getDefaultInstance(props, new javax.mail.Authenticator() { protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication("your_username", "your_password"); } }); try { // 创建邮件消息 Message message = new MimeMessage(session); message.setFrom(new InternetAddress("sender@example.com")); message.setRecipients(Message.RecipientType.TO, InternetAddress.parse("recipient@example.com")); message.setSubject("邮件标题"); message.setText("邮件正文"); // 发送邮件 Transport.send(message); System.out.println("邮件发送成功!"); } catch (MessagingException e) { System.out.println("邮件发送失败:" + e.getMessage()); } } }
In the above code, you first need to configure the SMTP server information, including the authentication method, host name and port number of the SMTP server. Then, create a Session object and pass in the configuration information of the SMTP server and the user name and password for authentication.
Next, create a Message object and set the sender, recipient, subject and body. Finally, send the email through the send() method of the Transport class.
2. Connection pool technology improves email sending efficiency
In high concurrency situations, frequently creating and destroying email sending connections may reduce the efficiency of email delivery. At this time, you can consider using connection pool technology to improve efficiency by reusing existing connections.
The following is a sample code that uses the Apache Commons Pool library to implement connection pooling:
import org.apache.commons.pool2.ObjectPool; import org.apache.commons.pool2.impl.GenericObjectPool; import javax.mail.*; public class ConnectionPool { private static final int MAX_CONNECTIONS = 10; private static final String SMTP_HOST = "smtp.example.com"; private static final int SMTP_PORT = 587; private static final String USER_NAME = "your_username"; private static final String PASSWORD = "your_password"; public static void main(String[] args) { Properties props = new Properties(); props.put("mail.smtp.auth", "true"); props.put("mail.smtp.host", SMTP_HOST); props.put("mail.smtp.port", SMTP_PORT); GenericObjectPool<Transport> pool = new GenericObjectPool<>(new TransportFactory(props, USER_NAME, PASSWORD)); pool.setMaxTotal(MAX_CONNECTIONS); try { Transport transport = pool.borrowObject(); Message message = new MimeMessage(Session.getDefaultInstance(props, null)); // 设置邮件消息... transport.sendMessage(message, message.getAllRecipients()); pool.returnObject(transport); System.out.println("邮件发送成功!"); } catch (Exception e) { System.out.println("邮件发送失败:" + e.getMessage()); } } } class TransportFactory extends BasePooledObjectFactory<Transport> { private final Properties properties; private final String userName; private final String password; public TransportFactory(Properties properties, String userName, String password) { this.properties = properties; this.userName = userName; this.password = password; } @Override public Transport create() throws Exception { Session session = Session.getInstance(properties, new Authenticator() { @Override protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication(userName, password); } }); return session.getTransport(); } @Override public PooledObject<Transport> wrap(Transport transport) { return new DefaultPooledObject<>(transport); } @Override public void destroyObject(PooledObject<Transport> p) throws Exception { p.getObject().close(); } }
In the above code, first set the maximum number of connections, the host and port of the SMTP server, and the user name and password. Then, create a GenericObjectPool object, passing in the configuration information of the connection pool and a custom pool factory class TransportFactory. In the create() method of TransportFactory, create a Session object and return its Transport.
Next, obtain a Transport from the connection pool through the borrowObject() method and use it to send mail. Finally, the Transport object is returned to the connection pool through the returnObject() method.
By using the connection pool, you can effectively control the creation and destruction of email sending connections and improve the efficiency of email delivery.
Conclusion:
This article introduces some tips and best practices for sending Java emails, including using the JavaMail API to send emails and using connection pooling technology to improve email sending efficiency. We hope that these methods and code examples can help developers optimize the email delivery process and improve application performance.
The above is the detailed content of Optimizing Java Email Sending: Tips and Practical Methods to Improve Email Transmission Efficiency. For more information, please follow other related articles on the PHP Chinese website!
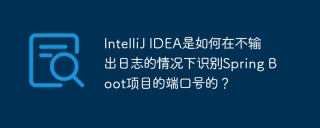
Start Spring using IntelliJIDEAUltimate version...
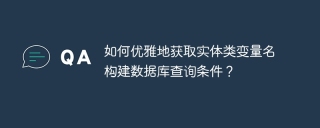
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
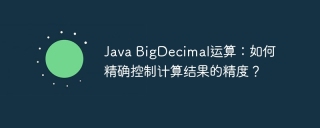
Java...
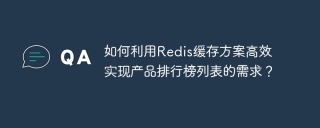
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
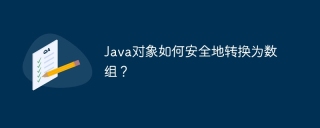
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
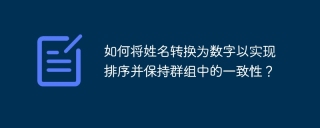
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
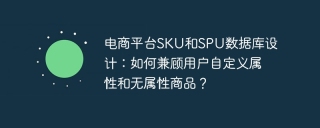
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
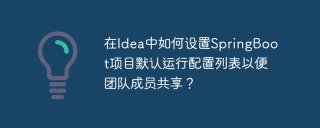
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
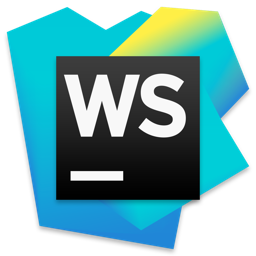
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
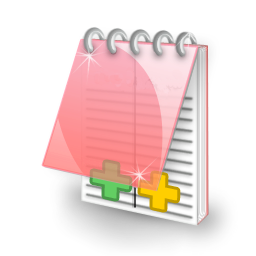
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software