


Improvement methods for Go language programs that efficiently handle large-capacity data
Methods to optimize Go language programs to handle large-volume data require specific code examples
Overview:
With the continuous growth of data scale, large-scale data Processing has become an important topic in modern software development. As an efficient and easy-to-use programming language, Go language can also well meet the needs of large-capacity data processing. This article will introduce some methods to optimize Go language programs to handle large volumes of data, and provide specific code examples.
1. Batch processing of data
When processing large-capacity data, one of the common optimization methods is to use batch processing of data. The traditional way of processing data one by one may cause large performance overhead. With the help of the concurrency mechanism of the Go language, we can process data in batches to improve processing efficiency.
Code example:
package main import ( "fmt" ) func processData(data []string) { for _, item := range data { // 处理单条数据 fmt.Println(item) } } func batchProcessData(data []string, batchSize int) { total := len(data) for i := 0; i < total; i += batchSize { end := i + batchSize if end > total { end = total } batch := data[i:end] go processData(batch) } } func main() { data := []string{"data1", "data2", "data3", "data4", "data5", "data6", "data7", "data8", "data9", "data10", "data11", "data12"} batchProcessData(data, 3) // 等待所有批次处理完成 select {} }
In the above code, we define the processData
function to process a single piece of data, and the batchProcessData
function to process the data Batch processing according to the specified batch size. In the main
function, we define a set of data, and then call the batchProcessData
function, specifying a batch size of 3. The batchProcessData
function will divide the data into several batches and execute the processData
function concurrently for processing.
2. Use buffer channels
The channel (Channel) in the Go language can be used for communication between coroutines. Combined with the characteristics of the buffer channel, we can further optimize the efficiency of large-capacity data processing.
Code example:
package main import ( "fmt" ) func processData(data []string, output chan<- string) { for _, item := range data { // 处理单条数据 fmt.Println(item) output <- item } } func main() { data := []string{"data1", "data2", "data3", "data4", "data5", "data6", "data7", "data8", "data9", "data10", "data11", "data12"} output := make(chan string, 3) // 创建一个缓冲通道 go processData(data, output) // 接收处理结果 for result := range output { // 处理结果 fmt.Println("处理结果:", result) } }
In the above code, we define the processData
function to process a single piece of data and send the processing result to the output channel. In the main
function, we create a buffer channel output
and call go processData
to start a new coroutine to process data. In the main thread, use the range
loop to continuously receive the processing results from the channel output
and process them.
3. Use concurrent atomic operations
In concurrent scenarios, using mutex locks to protect shared resources is a common operation, but mutex locks are expensive. The Go language provides methods related to atomic operations, which can optimize the processing of large-capacity data through atomic operations.
Code example:
package main import ( "fmt" "sync" "sync/atomic" ) func processData(data []int64, count *int64, wg *sync.WaitGroup) { defer wg.Done() for _, item := range data { // 处理单条数据 fmt.Println(item) atomic.AddInt64(count, 1) } } func main() { data := []int64{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12} var count int64 var wg sync.WaitGroup wg.Add(len(data)) for _, item := range data { go processData([]int64{item}, &count, &wg) } wg.Wait() fmt.Println("处理总数:", count) }
In the above code, we use the WaitGroup in the sync package to synchronize the coroutine that processes data. In the processData
function, we use the atomic.AddInt64
method to atomically increase the counter count
, avoiding the overhead of a mutex lock.
Conclusion:
Optimizing Go language programs to handle large volumes of data is an important technical task. By using batch processing of data, buffered channels, and concurrent atomic operations, we can effectively improve the performance and throughput of the program. In actual development, only by selecting appropriate optimization methods based on specific needs and scenarios, and making adjustments and improvements based on actual conditions, can the best performance optimization results be achieved.
The above is the detailed content of Improvement methods for Go language programs that efficiently handle large-capacity data. For more information, please follow other related articles on the PHP Chinese website!
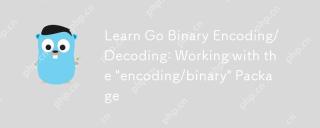
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
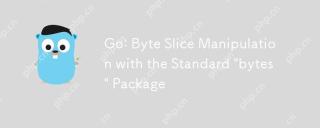
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
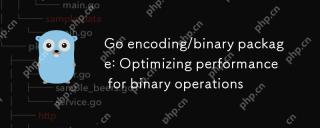
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
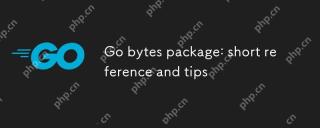
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
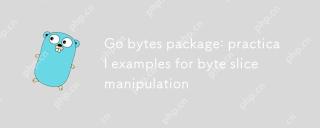
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.
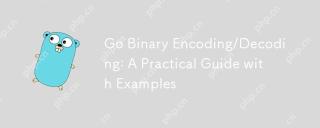
Go's encoding/binary package is a tool for processing binary data. 1) It supports small-endian and large-endian endian byte order and can be used in network protocols and file formats. 2) The encoding and decoding of complex structures can be handled through Read and Write functions. 3) Pay attention to the consistency of byte order and data type when using it, especially when data is transmitted between different systems. This package is suitable for efficient processing of binary data, but requires careful management of byte slices and lengths.
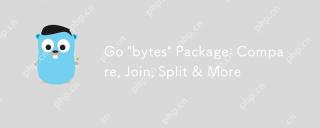
The"bytes"packageinGoisessentialbecauseitoffersefficientoperationsonbyteslices,crucialforbinarydatahandling,textprocessing,andnetworkcommunications.Byteslicesaremutable,allowingforperformance-enhancingin-placemodifications,makingthispackage
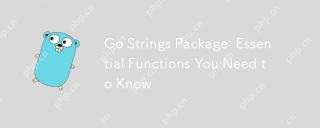
Go'sstringspackageincludesessentialfunctionslikeContains,TrimSpace,Split,andReplaceAll.1)Containsefficientlychecksforsubstrings.2)TrimSpaceremoveswhitespacetoensuredataintegrity.3)SplitparsesstructuredtextlikeCSV.4)ReplaceAlltransformstextaccordingto


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
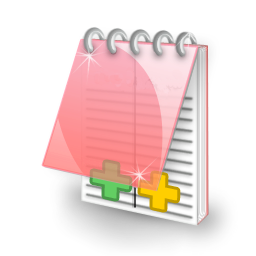
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor
