


Explore the internal implementation mechanism of object methods wait and notify in Java
In-depth understanding of object methods in Java: the underlying implementation principles of wait and notify require specific code examples
Object methods in Javawait
and notify
are the key methods used to implement inter-thread communication, and their underlying implementation principles involve the monitor mechanism of the Java virtual machine. This article will delve into the underlying implementation principles of these two methods and provide specific code examples.
First, let’s understand the basic uses of wait
and notify
. The function of the wait
method is to cause the current thread to release the object's lock and enter the waiting state until other threads call the object's notify
method to wake it up. The notify
method is used to wake up a thread waiting on the object and make it re-enter the runnable state.
Below we use specific code examples to illustrate the use and underlying implementation principles of the wait
and notify
methods.
public class WaitNotifyExample { public static void main(String[] args) { final Object lock = new Object(); // 线程A Thread threadA = new Thread(() -> { synchronized (lock) { try { System.out.println("ThreadA: 开始执行"); lock.wait(); // 线程A进入等待状态 System.out.println("ThreadA: 被唤醒,继续执行"); } catch (InterruptedException e) { e.printStackTrace(); } } }); // 线程B Thread threadB = new Thread(() -> { synchronized (lock) { System.out.println("ThreadB: 开始执行"); lock.notify(); // 唤醒等待的线程A System.out.println("ThreadB: 调用notify方法,结束"); } }); threadA.start(); threadB.start(); } }
In the above code, we create an example of waiting to wake up, where thread A enters the waiting state by calling the wait
method, and thread B by calling the notify
Method wakes up thread A. By running the above code, we can see that thread A continues execution after being awakened from the wait state.
After understanding the basic usage, let’s explore the underlying implementation principles of wait
and notify
. In the Java language, each object has a monitor associated with it, which is actually part of the Object Header. When a thread calls the wait
method of an object, the thread will release the object's lock and enter the waiting state, and add itself to the object's waiting queue. When other threads call the object's notify
method, the JVM will select a thread from the waiting queue to wake it up. Note that the awakened thread will continue to wait to acquire the object's lock. Only after it acquires the lock can it return from the wait
method to continue execution.
When executing the wait
and notify
methods, you must first obtain the lock of the object, otherwise an IllegalMonitorStateException
exception will be thrown. Therefore, these two methods must be used within a synchronized
block or method.
It should be noted that the wait
method and the notify
method can only operate on the same object, even different instances of the same class. In addition, the notify
method can only wake up one thread in the waiting queue. If there are multiple threads waiting, the specific thread to wake up is uncertain.
To sum up, the wait
and notify
methods are important methods to achieve inter-thread communication in Java, and their underlying implementation principles involve the monitor of the Java virtual machine. mechanism. By properly using the wait
and notify
methods, we can achieve synchronization and mutual exclusion between multiple threads, thereby ensuring thread safety while improving program performance.
I hope this article will help you understand the underlying implementation principles of object methods wait
and notify
in Java. If you still have questions about this, you can continue to learn more about it.
The above is the detailed content of Explore the internal implementation mechanism of object methods wait and notify in Java. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
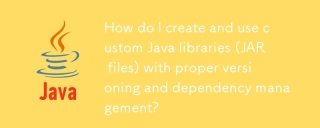
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
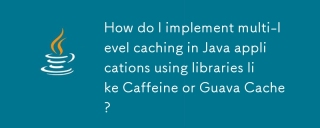
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
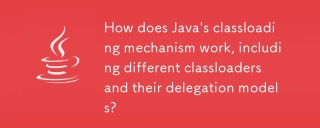
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
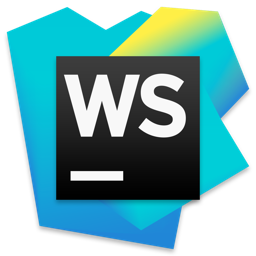
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
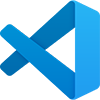
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor