Java and WebSocket: How to implement real-time weather forecasting
Java and WebSocket: How to implement real-time weather forecast
Introduction:
With the rapid development of technology, people's demand for real-time information is getting higher and higher. Weather forecast is one of the important information in our daily lives. If we can obtain the latest weather information in real time, it will bring great convenience to our lives. This article will introduce how to use Java and WebSocket technology to implement real-time weather forecasting functions, and provide specific code examples.
1. What is WebSocket?
WebSocket is a full-duplex communication protocol based on the TCP protocol. It can establish a persistent connection between the client and the server and achieve real-time two-way communication. Compared with the traditional HTTP protocol, WebSocket has lower latency and higher real-time performance, and is suitable for application scenarios that require high real-time performance.
2. Weather data acquisition
To realize the real-time weather forecast function, you first need to obtain real-time weather data. A common way is to use third-party weather APIs, such as Xinzhi Weather API, Hefeng Weather API, etc. By sending HTTP requests, we can get current weather conditions, temperature, wind strength and other information.
The following is a sample Java code that uses the Xinzhi Weather API to obtain weather data:
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class WeatherApi { public static void main(String[] args) { try { String url = "https://api.seniverse.com/v3/weather/now.json?key=your_api_key&location=beijing&language=zh-Hans&unit=c"; URL apiUrl = new URL(url); HttpURLConnection connection = (HttpURLConnection) apiUrl.openConnection(); connection.setRequestMethod("GET"); BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream())); StringBuilder response = new StringBuilder(); String line; while ((line = reader.readLine()) != null) { response.append(line); } reader.close(); System.out.println(response.toString()); } catch (Exception e) { e.printStackTrace(); } } }
In the code, we access the Xinzhi Weather API by sending an HTTP request, where your_api_key
needs to be replaced with your own API key, location
can be set to your city code.
3. WebSocket server construction
Next, we need to build a WebSocket server to establish a connection with the client and send real-time weather data to the client. There are many mature WebSocket libraries available in Java, such as Java-WebSocket, Tomcat WebSocket, etc. This article takes Java-WebSocket as an example.
First, we need to add the dependency of Java-WebSocket:
<dependency> <groupId>org.java-websocket</groupId> <artifactId>Java-WebSocket</artifactId> <version>1.5.1</version> </dependency>
Next, we start writing the code of the WebSocket server:
import org.java_websocket.WebSocket; import org.java_websocket.drafts.Draft_6455; import org.java_websocket.handshake.ClientHandshake; import org.java_websocket.server.WebSocketServer; import org.json.JSONObject; import java.net.InetSocketAddress; import java.util.concurrent.Executors; import java.util.concurrent.ScheduledExecutorService; import java.util.concurrent.TimeUnit; public class WeatherWebSocketServer extends WebSocketServer { private static final int WEATHER_UPDATE_INTERVAL = 1; // 每1分钟更新一次天气 public WeatherWebSocketServer(int port) { super(new InetSocketAddress(port), new Draft_6455()); } @Override public void onStart() { System.out.println("WebSocket服务器已启动"); scheduleWeatherUpdate(); } @Override public void onOpen(WebSocket conn, ClientHandshake handshake) { System.out.println("客户端已连接:" + conn.getRemoteSocketAddress()); } @Override public void onClose(WebSocket conn, int code, String reason, boolean remote) { System.out.println("客户端已断开连接:" + conn.getRemoteSocketAddress()); } @Override public void onMessage(WebSocket conn, String message) { System.out.println("收到客户端消息:" + message); } @Override public void onError(WebSocket conn, Exception ex) { System.out.println("WebSocket服务器出现错误:" + ex.getMessage()); } private void scheduleWeatherUpdate() { ScheduledExecutorService executor = Executors.newSingleThreadScheduledExecutor(); executor.scheduleAtFixedRate(() -> { String weatherData = getWeatherData(); broadcast(weatherData); }, 0, WEATHER_UPDATE_INTERVAL, TimeUnit.MINUTES); } private String getWeatherData() { // 调用前面获取天气数据的代码,返回当前天气数据 } private void broadcast(String message) { for (WebSocket conn : connections()) { conn.send(message); } } public static void main(String[] args) { int port = 8080; WeatherWebSocketServer server = new WeatherWebSocketServer(port); server.start(); System.out.println("WebSocket服务器已启动,监听端口:" + port); } }
In the code, we create a WebSocket server, and rewritten several key callback methods: onOpen
(triggered when the client connects), onClose
(triggered when the client disconnects), onMessage
(triggered when the client sends a message), onError
(triggered when an error occurs on the server).
We use ScheduledExecutorService
to update weather data regularly and send current weather data to all connected clients through the broadcast
method.
4. Client Implementation
Finally, we also need to write client code to accept real-time weather data sent by the server. The following is a simple HTML page as a demonstration:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>实时天气预报</title> </head> <body> <h1 id="实时天气预报">实时天气预报</h1> <div id="weatherData"></div> <script> var websocket = new WebSocket("ws://localhost:8080"); websocket.onopen = function() { console.log("建立WebSocket连接"); }; websocket.onmessage = function(event) { var weatherData = JSON.parse(event.data); document.getElementById("weatherData").innerHTML = "当前温度:" + weatherData.temperature + "℃"; }; websocket.onclose = function(event) { console.log("WebSocket连接已关闭"); }; </script> </body> </html>
In the above code, we create a WebSocket object and receive the weather data sent by the server by listening to the onmessage
event and display it on the HTML page.
5. Summary
Through the introduction of this article, we have learned how to use Java and WebSocket technology to implement real-time weather forecasting. We obtain real-time weather data through a third-party API and build a WebSocket server using Java-WebSocket to achieve real-time two-way communication with the client. Through a simple HTML page, we can receive real-time weather data and display it on the page.
It should be noted that the above code is just a simplified example, and more security and performance issues need to be considered in actual applications. I hope this article can help readers understand how to use Java and WebSocket to implement real-time weather forecasting, and provide some ideas and references for practical applications.
The above is the detailed content of Java and WebSocket: How to implement real-time weather forecasting. For more information, please follow other related articles on the PHP Chinese website!
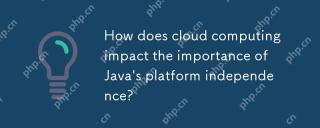
Cloud computing significantly improves Java's platform independence. 1) Java code is compiled into bytecode and executed by the JVM on different operating systems to ensure cross-platform operation. 2) Use Docker and Kubernetes to deploy Java applications to improve portability and scalability.
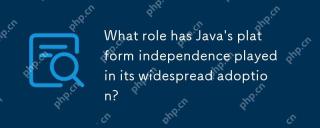
Java'splatformindependenceallowsdeveloperstowritecodeonceandrunitonanydeviceorOSwithaJVM.Thisisachievedthroughcompilingtobytecode,whichtheJVMinterpretsorcompilesatruntime.ThisfeaturehassignificantlyboostedJava'sadoptionduetocross-platformdeployment,s
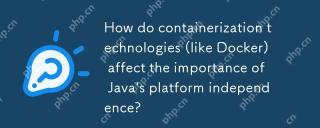
Containerization technologies such as Docker enhance rather than replace Java's platform independence. 1) Ensure consistency across environments, 2) Manage dependencies, including specific JVM versions, 3) Simplify the deployment process to make Java applications more adaptable and manageable.
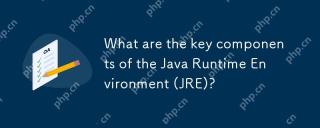
JRE is the environment in which Java applications run, and its function is to enable Java programs to run on different operating systems without recompiling. The working principle of JRE includes JVM executing bytecode, class library provides predefined classes and methods, configuration files and resource files to set up the running environment.
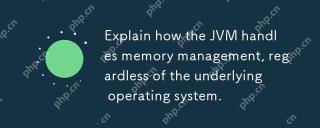
JVM ensures efficient Java programs run through automatic memory management and garbage collection. 1) Memory allocation: Allocate memory in the heap for new objects. 2) Reference count: Track object references and detect garbage. 3) Garbage recycling: Use the tag-clear, tag-tidy or copy algorithm to recycle objects that are no longer referenced.
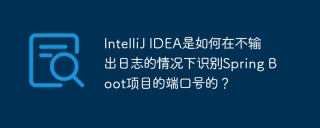
Start Spring using IntelliJIDEAUltimate version...
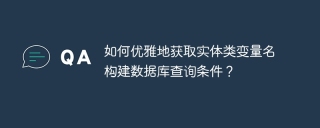
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
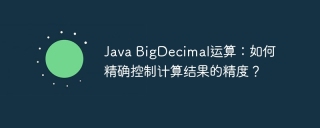
Java...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
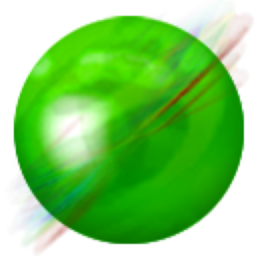
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
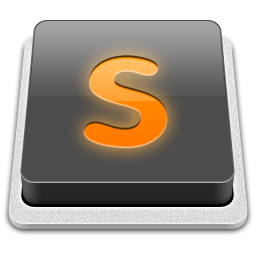
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
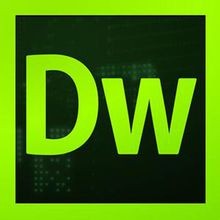
Dreamweaver CS6
Visual web development tools