


WebSocket and JavaScript: Creating an efficient real-time data interaction system
Introduction:
In modern Web applications, real-time data interaction is becoming more and more important . The traditional HTTP request-response model has some limitations. For example, each request requires re-establishing the connection, the server can only actively send data, and the client cannot actively obtain the latest updates. The emergence of WebSocket technology fills these gaps and provides developers with a real-time, two-way, low-latency data interaction method. This article will introduce how to use WebSocket and JavaScript to create an efficient real-time data interaction system, and provide specific code examples.
1. What is WebSocket
WebSocket is a protocol for full-duplex communication over a single TCP connection, which allows real-time data exchange between the client and the server. Through WebSocket, the browser can establish a long-term connection with the server and send messages in both directions. The server can actively send data to the client instead of just responding to requests. In addition, WebSocket provides a more efficient way to transfer data by reducing the overhead and latency of HTTP connections.
2. Steps to use WebSocket
- Establishing a WebSocket connection
First, we need to create a WebSocket object in JavaScript and specify the server address to connect to. The code example is as follows:
var ws = new WebSocket("ws://example.com/socket");
- Listening to WebSocket events
Once the WebSocket connection is established, we can listen to some events to respond when the connection status changes or new messages are received. processing. The following are some commonly used WebSocket events:
- open: triggered when the connection is successfully established.
- message: Triggered when a message sent by the server is received.
- close: Triggered when the connection is closed.
Code example:
ws.onopen = function() { console.log("WebSocket连接已建立"); }; ws.onmessage = function(event) { var message = event.data; console.log("收到消息:" + message); }; ws.onclose = function(event) { console.log("连接已关闭"); };
- Sending and receiving messages
Use the send method of the WebSocket object to send messages to the server, and the server receives messages through the onmessage event of WebSocket . The following is a simple example showing how to send and receive messages:
ws.send("Hello Server!"); ws.onmessage = function(event) { var message = event.data; console.log("收到消息:" + message); };
- Close WebSocket connection
When the WebSocket connection is no longer needed, we should actively close it to release resources and terminate the connection. You can use the close method of the WebSocket object to close the connection:
ws.close();
3. Real-time data interaction example using WebSocket
The following is an example of a real-time chat room using WebSocket, showing how to use WebSocket to implement Real-time messaging.
First, we create a WebSocket object and specify the connected server address:
var ws = new WebSocket("ws://example.com/chatroom");
Then, we listen to the open, message and close events of WebSocket:
ws.onopen = function() { console.log("WebSocket连接已建立"); }; ws.onmessage = function(event) { var message = event.data; console.log("收到消息:" + message); // 在页面上展示新消息 displayMessage(message); }; ws.onclose = function(event) { console.log("连接已关闭"); };
On the page , we can provide an input box and a send button, and the user can enter a message and click the send button. We can send messages to the server through the following code:
var inputElement = document.getElementById("message-input"); var sendButton = document.getElementById("send-button"); sendButton.onclick = function() { var message = inputElement.value; ws.send(message); inputElement.value = ""; };
At the same time, we can define a function for displaying new messages, such as adding new messages to the chat record list:
function displayMessage(message) { var messageList = document.getElementById("message-list"); var listItem = document.createElement("li"); listItem.textContent = message; messageList.appendChild(listItem); }
When the server receives a new message, it sends the message to all connected clients through the send method of WebSocket, and then the client receives the new message through the onmessage event and displays it on the page.
Conclusion:
Through WebSocket and JavaScript, we can easily implement a real-time data interaction system. WebSocket provides an efficient and low-latency data transmission method, making real-time data interaction possible. Developers can use WebSocket to build various real-time applications based on actual needs, such as chat rooms, instant messaging, real-time data monitoring, etc. Creative use of WebSocket can provide users with a better user experience and more efficient data interaction.
The above is the detailed content of WebSocket and JavaScript: Create an efficient real-time data interaction system. For more information, please follow other related articles on the PHP Chinese website!
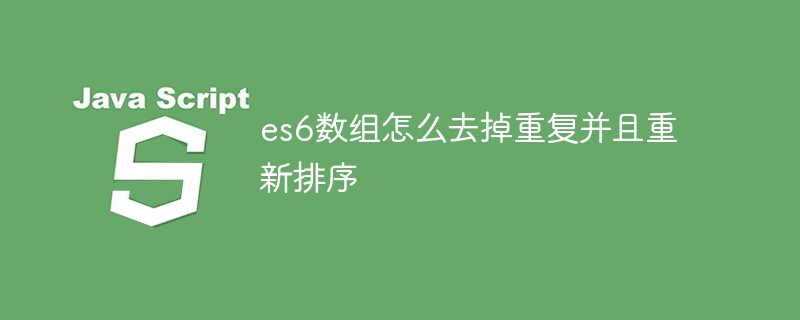
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
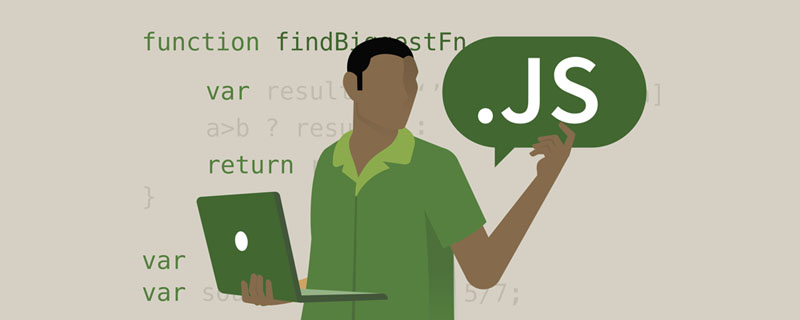
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
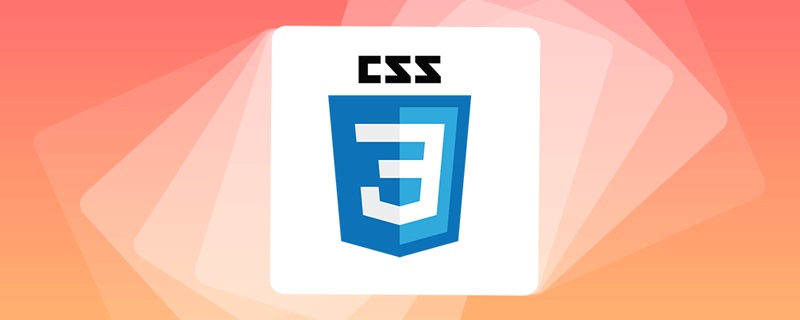
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
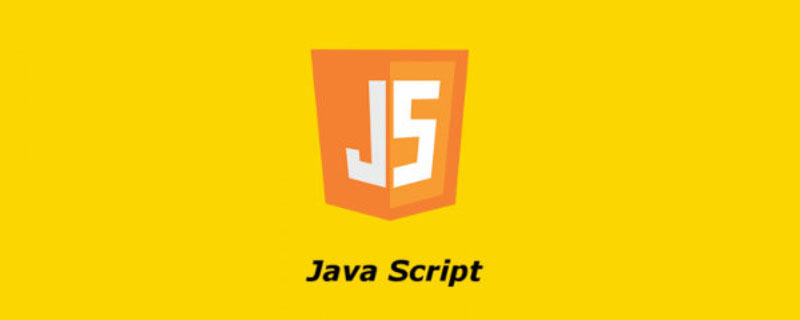
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
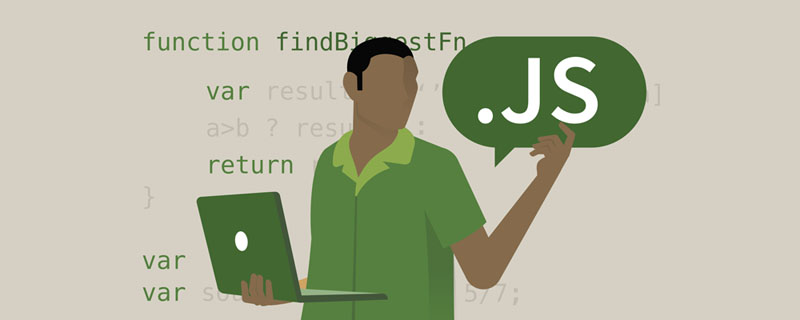
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
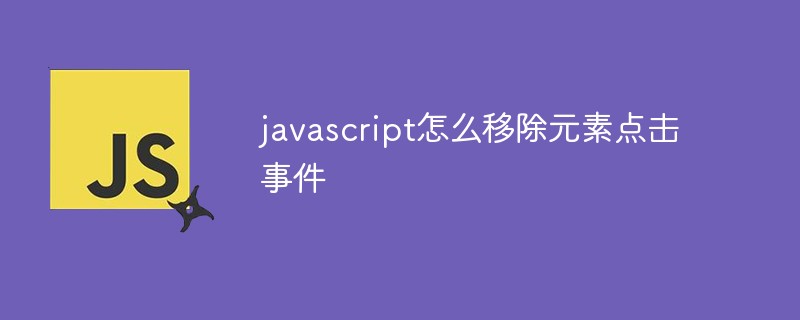
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
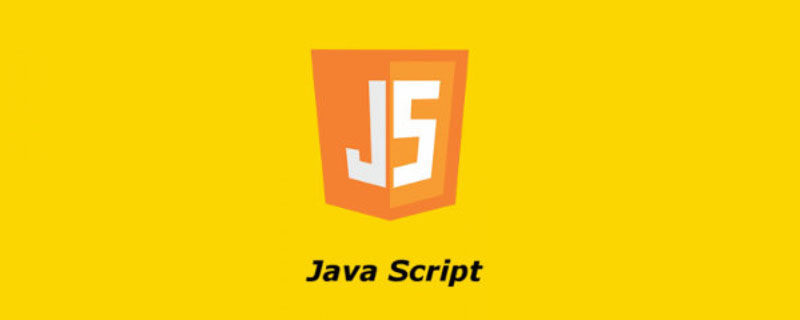
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
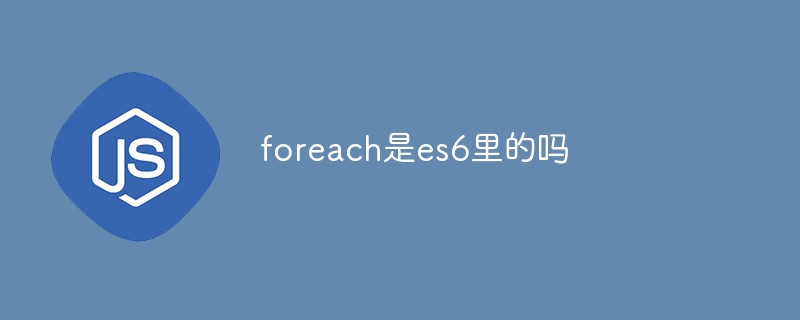
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
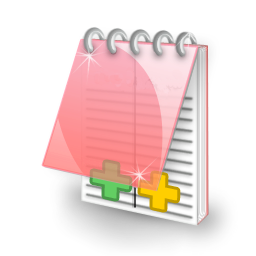
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
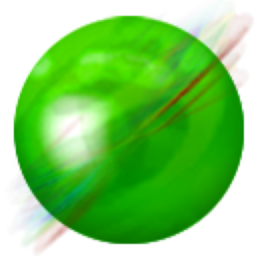
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
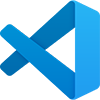
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
