


Asynchronous Coroutine Development Guide: Optimizing the speed and efficiency of big data processing requires specific code examples
[Introduction]
As the amount of data continues to increase As business needs continue to improve, big data processing is becoming more and more common. Traditional synchronous programming methods will face performance bottlenecks and low efficiency when processing large amounts of data. Asynchronous coroutine development can make full use of computing resources and improve the speed and efficiency of data processing by executing tasks concurrently. This article will introduce the basic concepts and specific code examples of asynchronous coroutine development to help readers understand and master this development technology.
[What is asynchronous coroutine development]
Asynchronous coroutine development is a concurrent programming technology that decomposes the tasks in the program into independent coroutines so that these coroutines can be executed concurrently , and switch according to a specific scheduling algorithm. Compared with traditional multi-threaded programming, coroutines are more lightweight, have no switching overhead between threads, and are more suitable for large-scale data processing.
[Advantages of asynchronous coroutines]
- Reduce waiting time: Asynchronous coroutines can make full use of computing resources, allowing the program to perform other tasks while waiting for IO, reducing waiting time. Improve efficiency.
- Improve overall performance: Due to the lightweight nature of coroutines, high concurrent processing can be achieved, greatly improving the speed and throughput of data processing.
- Simplify programming logic: Asynchronous coroutines can simplify complex thread synchronization issues, reduce the use of thread synchronization mechanisms such as locks and conditions, and reduce programming difficulty.
[Specific code examples for asynchronous coroutine development]
The following will give a code example of a practical scenario to demonstrate the application of asynchronous coroutine development in big data processing.
Suppose there is a requirement: read data from a database that stores massive data, perform some kind of processing operation, and finally write the processing results to another database. Traditional synchronous programming may take a long time, but using asynchronous coroutines can greatly improve processing speed and efficiency.
First, we use Python’s coroutine library asynio to implement asynchronous coroutine development. The following is a coroutine function that reads database data:
import aiohttp async def fetch_data(url): async with aiohttp.ClientSession() as session: async with session.get(url) as response: data = await response.json() return data
In the above code, we use the aiohttp
library to send asynchronous HTTP requests and return the response data in JSON format.
Next is the coroutine function for processing data:
async def process_data(data): # 处理数据的逻辑 # ... return processed_data
In the process_data
function, we can write specific data processing logic.
The last is the coroutine function that writes to the database:
import aiomysql async def write_data(data): conn = await aiomysql.connect(host='localhost', port=3306, user='username', password='password', db='database') cursor = await conn.cursor() await cursor.execute('INSERT INTO table (data) VALUES (?)', (data,)) await conn.commit() await cursor.close() conn.close()
In the above code, we use the aiomysql
library to connect to the database and perform the insertion operation.
Finally, in the main function, we can schedule and run these coroutine functions by creating an event loop:
import asyncio async def main(): url = 'http://www.example.com/api/data' data = await fetch_data(url) processed_data = await process_data(data) await write_data(processed_data) loop = asyncio.get_event_loop() loop.run_until_complete(main())
Through the above code example, we can see that asynchronous coroutine Program development can handle large-scale data in a very concise and efficient way. In actual applications, we can tune and expand according to specific needs and environments, such as setting the number of concurrencies, using cache, etc.
[Conclusion]
Asynchronous coroutine development is an important technology to improve the speed and efficiency of big data processing. This article introduces the basic concepts and advantages of asynchronous coroutines through the introduction, and then gives a specific code example to demonstrate the application of asynchronous coroutine development in big data processing. By learning and mastering asynchronous coroutine development, we can better cope with the challenges of the big data era and improve the speed and efficiency of data processing.
The above is the detailed content of Asynchronous Coroutine Development Guide: Optimizing the Speed and Efficiency of Big Data Processing. For more information, please follow other related articles on the PHP Chinese website!
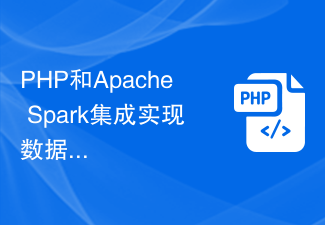
随着数据的不断增长,数据分析和处理的需求也越来越重要。因此,现在越来越多的人开始将PHP和ApacheSpark集成来实现数据分析和处理。在本文中,我们将讨论什么是PHP和ApacheSpark,如何将二者集成到一起,并且用实例说明集成后的数据分析和处理过程。什么是PHP和ApacheSpark?PHP是一种通用的开源脚本语言,主要用于Web开发和服务
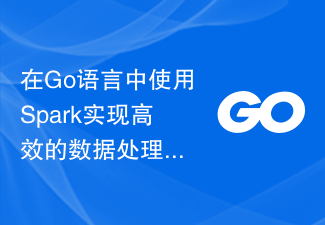
随着大数据时代的到来,数据处理变得越来越重要。对于各种不同的数据处理任务,不同的技术也应运而生。其中,Spark作为一种适用于大规模数据处理的技术,已经被广泛地应用于各个领域。此外,Go语言作为一种高效的编程语言,也在近年来得到了越来越多的关注。在本文中,我们将探讨如何在Go语言中使用Spark实现高效的数据处理。我们将首先介绍Spark的一些基本概念和原理
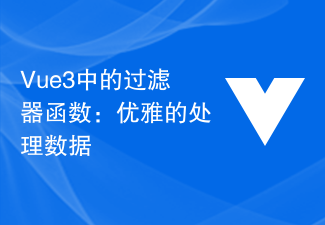
Vue3中的过滤器函数:优雅的处理数据Vue是一个流行的JavaScript框架,拥有庞大的社区和强大的插件系统。在Vue中,过滤器函数是一种非常实用的工具,允许我们在模板中对数据进行处理和格式化。Vue3中的过滤器函数有了一些改变,在这篇文章中,我们将深入探讨Vue3中的过滤器函数,学习如何使用它们优雅地处理数据。什么是过滤器函数?在Vue中,过滤器函数是
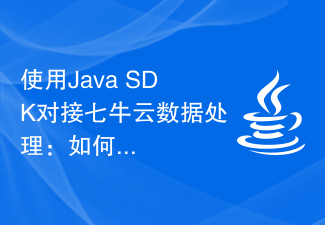
使用JavaSDK对接七牛云数据处理:如何实现数据转换和分析?概述:在云计算和大数据时代,数据处理是一个非常重要的环节。七牛云提供了强大的数据处理功能,可以对存储在七牛云中的各种类型的文件进行图像处理、音视频处理、文字处理等。本文将介绍如何使用JavaSDK对接七牛云的数据处理功能,并给出一些常用的代码示例。安装JavaSDK首先,我们需要在项目中引入
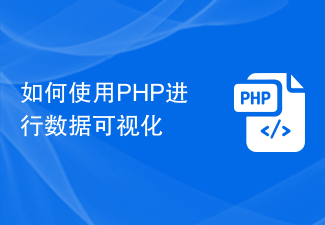
数据可视化是当前许多企业和个人在处理数据时非常关注的问题,它可以将复杂的数据信息转化为直观易懂的图表和图像,从而帮助用户更好地了解数据的内在规律和趋势。而PHP作为一种高效的脚本语言,在数据可视化方面也具有一定的优势,本文将介绍如何使用PHP进行数据可视化。一、了解PHP图表插件在PHP的数据可视化领域,大量的图表插件可以提供图表绘制、图表美化以及图表数据呈
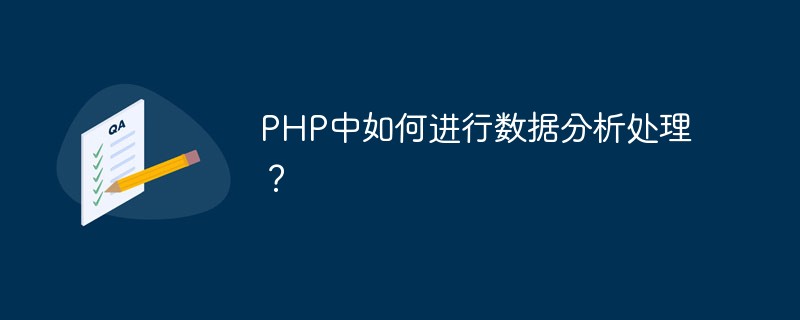
PHP是一门广泛应用于Web开发的语言,通常被用来构建动态的Web应用程序。随着数据驱动型应用程序的兴起,PHP在数据分析和处理方面也变得越来越重要。本文将介绍如何使用PHP进行数据分析处理,从数据的获取、存储、分析和可视化展示等方面进行讲解。一、数据获取要进行数据分析处理,首先需要获取数据。数据可以来自各种不同的来源,例如数据库、文件、网络等。在PHP中,
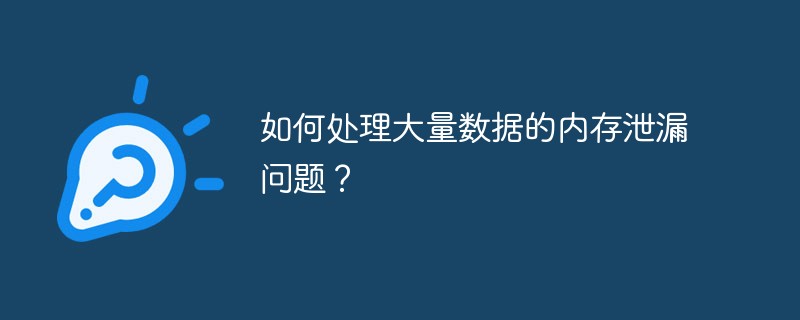
随着数据量不断增大,数据分析和处理也变得越来越复杂。在大规模数据处理的过程中,内存泄漏是很常见的问题之一。如果不正确地处理,内存泄漏不仅会导致程序崩溃,还会对性能和稳定性产生严重影响。本文将介绍如何处理大量数据的内存泄漏问题。了解内存泄漏的原因和表现内存泄漏是指程序在使用内存过程中,分配的内存没有被及时释放而导致内存空间浪费。这种情况常常发生在大量数据处理的
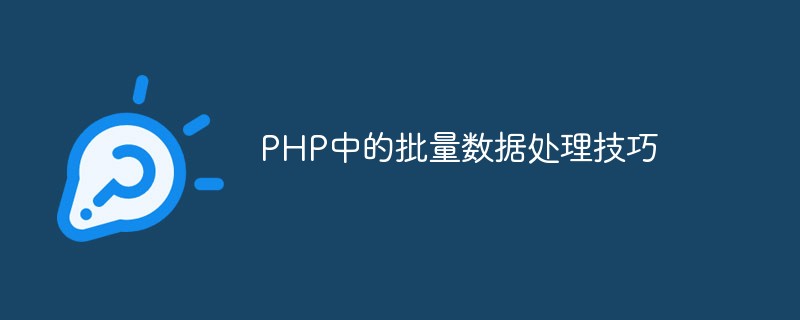
随着互联网和信息技术的迅速发展,数据处理已经成为了现代计算机科学和工程学的一个重要研究领域,许多程序员和开发者都需要在他们的应用程序中处理大量数据。PHP作为一种简单易用的脚本语言,也逐渐成为了数据处理中的有力工具。在本文中,我们将介绍PHP中的一些批量数据处理技巧,以帮助开发者更高效地处理大量数据。使用for循环处理数据for循环是PHP中最基本的循环结构


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
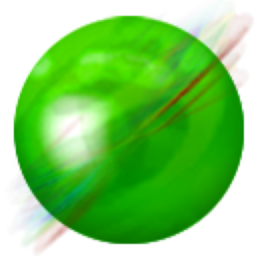
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
