What is the difference between crypt() and password_hash()?
The crypt()
and password_hash()
functions are both used for hashing passwords in PHP, but they have several key differences in their implementation and usage.
-
Implementation and Algorithm Support:
-
crypt()
is a more generic function that supports a variety of algorithms for hashing, depending on the system it's being run on. It can use different algorithms like MD5, Blowfish, and SHA-256. The specific algorithm used can be specified by the salt parameter. -
password_hash()
is a more specialized function specifically designed for hashing passwords. It uses bcrypt by default but can also use Argon2 and other algorithms with future updates. The algorithm choice is easier to manage as it's an option parameter.
-
-
Ease of Use:
-
crypt()
requires the developer to manually manage the salt and the algorithm selection, which can lead to potential security issues if not done correctly. -
password_hash()
abstracts away the complexities of managing salts and algorithm choices. It automatically generates a secure salt and uses bcrypt as the default algorithm, making it much easier and safer to use.
-
-
Output Format:
-
crypt()
produces a string that contains both the hashed password and the salt used, but the format can vary depending on the algorithm used. -
password_hash()
produces a standardized output format that includes the algorithm used, the cost parameter, and the salt along with the hashed password.
-
-
Verification:
- With
crypt()
, verification of a hashed password requires the developer to manually manage the comparison, often usingcrypt()
again with the provided password and the stored hash's salt. -
password_hash()
comes with a companion functionpassword_verify()
that simplifies the verification process by automatically extracting the necessary information from the stored hash.
- With
How does the security level compare between crypt() and password_hash()?
The security level of crypt()
versus password_hash()
can be compared based on several factors:
-
Algorithm Strength:
-
crypt()
supports various algorithms, some of which are outdated (like MD5) and less secure. It is up to the developer to choose a modern and secure algorithm. -
password_hash()
uses bcrypt by default, which is considered robust and suitable for password storage. It also supports newer algorithms like Argon2, which can be used to enhance security further.
-
-
Salt Management:
-
crypt()
requires manual salt management, which can lead to vulnerabilities if not implemented correctly. -
password_hash()
automatically generates a unique salt for each password, reducing the risk of common salt-related vulnerabilities like rainbow table attacks.
-
-
Ease of Implementation:
-
crypt()
requires a deeper understanding of cryptographic principles to use securely, increasing the chance of errors. -
password_hash()
is designed to be secure out-of-the-box, minimizing the risk of implementation errors.
-
-
Future-Proofing:
-
crypt()
might require manual updates to change the hashing algorithm, potentially leaving systems vulnerable if not properly maintained. -
password_hash()
can be updated to use newer algorithms (like Argon2) without changing the existing codebase, as long as the function call remains the same.
-
Overall, password_hash()
provides a higher security level due to its ease of use, better default algorithm choices, and automatic salt management.
Which function, crypt() or password_hash(), is more suitable for modern web applications?
For modern web applications, password_hash()
is more suitable due to the following reasons:
-
Security:
- As mentioned earlier,
password_hash()
offers better security by default through its use of bcrypt and automatic salt management. It's designed with modern security practices in mind, reducing the risk of common vulnerabilities.
- As mentioned earlier,
-
Ease of Use:
- The simplicity of
password_hash()
allows developers to implement secure password hashing without deep cryptographic knowledge. This reduces the likelihood of mistakes that could compromise security.
- The simplicity of
-
Future-Proofing:
-
password_hash()
can be easily updated to use newer, stronger algorithms without requiring significant changes to existing code, making it a future-proof choice for web applications.
-
-
Standardization:
- It produces a standardized output format that is easy to work with, and its companion function
password_verify()
simplifies the password verification process.
- It produces a standardized output format that is easy to work with, and its companion function
While crypt()
can be used securely with the right configuration and care, password_hash()
is the more suitable choice for most modern web applications due to its robust security features and ease of implementation.
What are the key considerations when choosing between crypt() and password_hash() for password storage?
When choosing between crypt()
and password_hash()
for password storage, consider the following key factors:
-
Security Requirements:
- Evaluate the level of security required for your application. If you need robust and easy-to-implement security,
password_hash()
is generally the better choice due to its default use of bcrypt and automatic salt management.
- Evaluate the level of security required for your application. If you need robust and easy-to-implement security,
-
Ease of Implementation:
- Consider your team's experience with cryptography. If your team has limited cryptographic knowledge,
password_hash()
is more straightforward and less prone to errors.
- Consider your team's experience with cryptography. If your team has limited cryptographic knowledge,
-
Flexibility:
- If you need more control over the hashing algorithm and are willing to manage the security aspects carefully,
crypt()
might be suitable. However, remember that this increases the complexity and potential for errors.
- If you need more control over the hashing algorithm and are willing to manage the security aspects carefully,
-
Future-Proofing:
- Consider the ease of updating the hashing algorithm in the future.
password_hash()
allows for seamless transitions to newer algorithms, whilecrypt()
might require more significant changes.
- Consider the ease of updating the hashing algorithm in the future.
-
Standardization and Compatibility:
- Evaluate whether standardized output formats and built-in verification functions are important for your project.
password_hash()
withpassword_verify()
provides a clean, standardized approach to password management.
- Evaluate whether standardized output formats and built-in verification functions are important for your project.
-
Performance and Cost:
- Assess the performance impact of the chosen hashing method. Bcrypt, used by
password_hash()
, is computationally intensive, which might be a consideration for high-traffic applications. However, this also contributes to its security.
- Assess the performance impact of the chosen hashing method. Bcrypt, used by
In summary, for most applications, password_hash()
is the recommended choice due to its balance of security, ease of use, and future-proofing capabilities. However, understanding the specific needs and constraints of your project is crucial in making an informed decision.
The above is the detailed content of What is the difference between crypt() and password_hash()?. For more information, please follow other related articles on the PHP Chinese website!
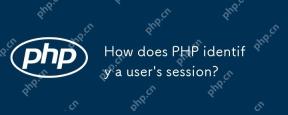
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
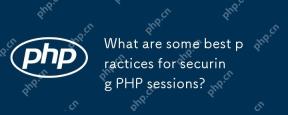
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
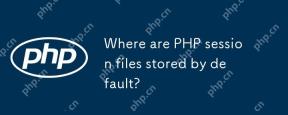
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita
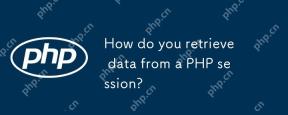
ToretrievedatafromaPHPsession,startthesessionwithsession_start()andaccessvariablesinthe$_SESSIONarray.Forexample:1)Startthesession:session_start().2)Retrievedata:$username=$_SESSION['username'];echo"Welcome,".$username;.Sessionsareserver-si
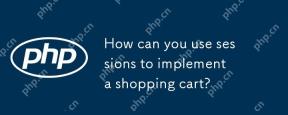
The steps to build an efficient shopping cart system using sessions include: 1) Understand the definition and function of the session. The session is a server-side storage mechanism used to maintain user status across requests; 2) Implement basic session management, such as adding products to the shopping cart; 3) Expand to advanced usage, supporting product quantity management and deletion; 4) Optimize performance and security, by persisting session data and using secure session identifiers.
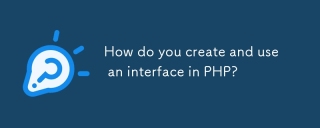
The article explains how to create, implement, and use interfaces in PHP, focusing on their benefits for code organization and maintainability.
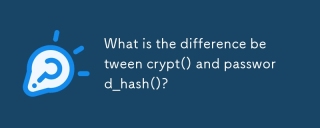
The article discusses the differences between crypt() and password_hash() in PHP for password hashing, focusing on their implementation, security, and suitability for modern web applications.
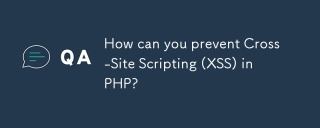
Article discusses preventing Cross-Site Scripting (XSS) in PHP through input validation, output encoding, and using tools like OWASP ESAPI and HTML Purifier.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
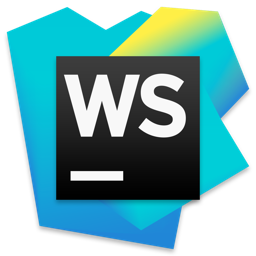
WebStorm Mac version
Useful JavaScript development tools
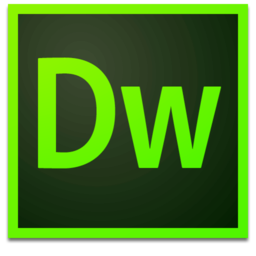
Dreamweaver Mac version
Visual web development tools
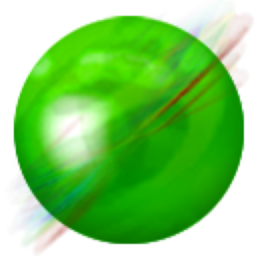
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
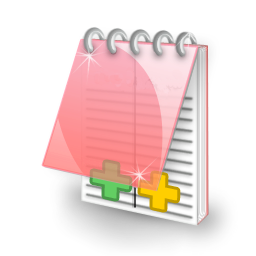
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
