


JavaScript and WebSocket: Building an efficient real-time weather forecasting system
JavaScript and WebSocket: Building an efficient real-time weather forecast system
Introduction:
Today, the accuracy of weather forecasts is of great significance to daily life and decision-making . As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples.
- Introduction to WebSocket
WebSocket is a full-duplex communication protocol based on the TCP protocol, which can establish a persistent connection between the client and the server and achieve real-time two-way data transmission. This allows us to obtain weather data in real time and display it to the user. - Get real-time weather data
In order to get real-time weather data, we can use the public weather API. Here we take OpenWeatherMap as an example. This API provides various weather parameters, such as temperature, humidity, wind speed, etc. We can get real-time weather data for a specific city by sending an HTTP request to the API.
The following is a basic sample code that uses JavaScript to send an HTTP request:
const city = "北京"; const apiKey = "YOUR_API_KEY"; const url = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}`; fetch(url) .then(response => response.json()) .then(data => { // 获取到实时天气数据后的处理逻辑 console.log(data); }) .catch(error => { // 处理错误 console.error(error); });
The above code sends an HTTP request using the fetch function to obtain the real-time weather in JSON format returned by the OpenWeatherMap API data. We can choose to parse the data as needed and extract the weather parameters we need.
- Use WebSocket to establish a real-time connection
In order to achieve real-time weather forecast, we need to establish a persistent connection and receive server-side data updates in real time. For this we can use WebSocket technology.
JavaScript provides the WebSocket API to facilitate us to establish a WebSocket connection between the client and the server. The following is a sample code for establishing a simple WebSocket connection:
const socket = new WebSocket("wss://example.com/weather"); socket.addEventListener("open", (event) => { // 连接建立成功后的处理逻辑 console.log("WebSocket 连接已建立"); }); socket.addEventListener("message", (event) => { // 接收到服务器端发送的消息后的处理逻辑 const data = JSON.parse(event.data); console.log(data); }); socket.addEventListener("error", (error) => { // 处理连接错误 console.error(error); }); socket.addEventListener("close", (event) => { // 连接关闭后的处理逻辑 console.log("WebSocket 连接已关闭"); });
The above code uses the WebSocket constructor to create a WebSocket object and specifies the address of the server. By listening to different events, we can implement processing logic in different situations such as successful connection establishment, message reception, connection errors, and connection closure.
- Combining WebSocket and Real-time Weather API
Now, we will combine the previous two parts to implement a real-time weather forecast system.
const socket = new WebSocket("wss://example.com/weather"); socket.addEventListener("open", (event) => { console.log("WebSocket 连接已建立"); const city = "北京"; const apiKey = "YOUR_API_KEY"; const data = { action: "subscribe", city: city, apiKey: apiKey, }; socket.send(JSON.stringify(data)); }); socket.addEventListener("message", (event) => { const data = JSON.parse(event.data); console.log(data); // 更新界面显示天气信息 displayWeather(data); }); socket.addEventListener("error", (error) => { console.error(error); }); socket.addEventListener("close", (event) => { console.log("WebSocket 连接已关闭"); }); function displayWeather(data) { // 根据数据更新界面显示天气信息的逻辑 // ... }
The above code sends a data object containing the subscription city and API key to the server after the WebSocket connection is established. After receiving the data, the server obtains weather data in real time based on the subscribed city and sends the data to the client. After receiving the weather data, the client can process the data as needed and update the weather information displayed on the interface.
Conclusion:
By combining JavaScript and WebSocket technology, we can build an efficient real-time weather forecast system. By working with the real-time weather API, we can obtain and update weather data in real time when the user subscribes to the city. This real-time weather forecast system can provide users with accurate and timely weather information and improve user experience.
The above is the detailed content of JavaScript and WebSocket: Building an efficient real-time weather forecasting system. For more information, please follow other related articles on the PHP Chinese website!
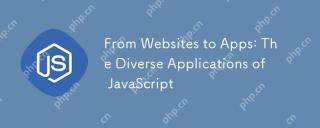
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
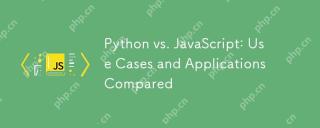
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
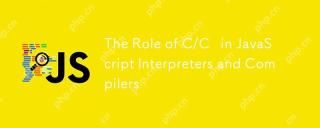
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
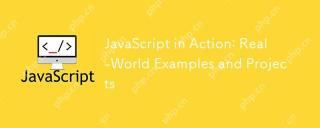
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
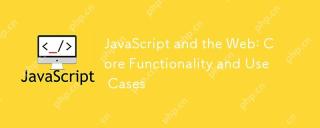
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
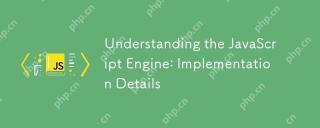
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
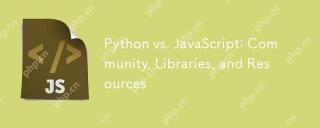
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.