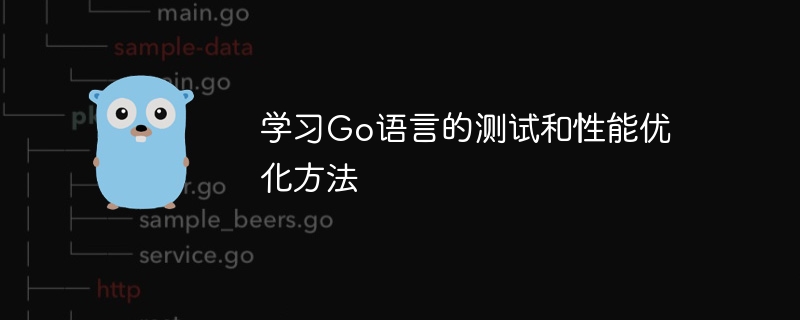
Learn testing and performance optimization methods of Go language
Go language is an open source programming language known for its simplicity, efficiency and concurrency performance. With the wide application of Go language in the fields of cloud computing, network services and big data, the demand for Go language testing and performance optimization is also increasing day by day. This article will introduce testing and performance optimization methods for learning Go language to help readers better understand and use Go language.
1. Testing method
- Unit testing: Unit testing refers to testing a minimum module in the program to verify whether its functions meet expectations. In Go language, unit testing is very simple. You only need to create a test file ending with _test.go in the code file and write the test function in it. Just use the go test command to run the test. For example:
package main
import "testing"
func TestAdd(t *testing.T) {
result := Add(1, 2)
if result != 3 {
t.Errorf("Add(1, 2) = %d; want 3", result)
}
}
- Benchmark testing: Benchmark testing is a testing method used to test the performance of a program under given conditions. In the Go language, benchmarks are controlled using objects of type testing.B. Evaluate the performance of your program by using b.N to determine the number of runs of the benchmark. For example:
package main
import "testing"
func BenchmarkAdd(b *testing.B) {
for i := 0; i < b.N; i++ {
Add(1, 2)
}
}
Run the go test -bench=. command in the command line to run the benchmark test.
2. Performance optimization method
- Use concurrency: Go language inherently supports concurrency, which is achieved by using goroutine and channel. When you need to handle a large number of concurrent tasks, you can use goroutine to execute tasks concurrently, and then synchronize the end of the tasks through channels. This can make full use of the performance of multi-core processors and improve the processing capabilities of the program.
- Avoid memory allocation: In performance optimization, it is important to try to avoid excessive memory allocation and recycling. In the Go language, you can use sync.Pool to cache objects, which can minimize the overhead of memory allocation. In addition, try to avoid using unnecessary pointers and slices to reduce the number of memory allocations.
- Use performance analysis tools: Go language provides some performance analysis tools, such as pprof and go tool pprof. By using these tools, you can analyze the performance bottlenecks of your program and then optimize them accordingly. For example, you can use the go tool pprof command to view a program's CPU usage and memory allocation.
- Write efficient algorithms and data structures: For programs with high performance requirements, it is important to write efficient algorithms and data structures. When designing algorithms and data structures, try to avoid unnecessary calculations and memory accesses to reduce the time and space complexity of the program.
Summary
By learning the testing and performance optimization methods of Go language, you can better understand and use Go language. When writing Go language programs, be sure to pay attention to writing unit tests and benchmark tests to ensure the quality and performance of the program. At the same time, the performance of Go language programs can be further improved by using concurrency, avoiding memory allocation, using performance analysis tools, and writing efficient algorithms and data structures. Continuously learn and practice to create efficient Go language programs and contribute to the success of software development.
The above is the detailed content of Learn testing and performance optimization methods of Go language. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn