


C++ Reflection Mechanism Practice: Implementing Flexible Runtime Type Information
C Reflection Mechanism Practice: Implementing Flexible Runtime Type Information
Introduction: C is a strongly typed language and does not directly provide reflection mechanisms like other languages. Get the type information of the class. However, with some tricks and technical means, we can also implement similar reflection functions in C. This article describes how to leverage template metaprogramming and macro definitions to achieve flexible runtime type information.
1. What is the reflection mechanism?
The reflection mechanism refers to obtaining the type information of the class at runtime, such as the name of the class, member functions, member variables and other attributes. Through the reflection mechanism, we can dynamically operate on a class without knowing its specific type in advance. In many object-oriented languages, such as Java, C#, etc., the reflection mechanism is built-in and can be used directly, while C does not have native reflection function. However, we can simulate it through some means.
2. Type information based on template metaprogramming
- In C, we can use template metaprogramming to obtain type information. Through function template specialization and type inference, we can automatically deduce the true type of a variable. For example, we can define a template function
getTypeName
to get the name of any type:
template<typename T> std::string getTypeName() { return typeid(T).name(); }
- Then, we can use this function to get the name of any type :
int main() { std::cout << getTypeName<int>() << std::endl; // 输出 int std::cout << getTypeName<double>() << std::endl; // 输出 double std::cout << getTypeName<std::string>() << std::endl; // 输出 std::string return 0; }
Through template metaprogramming, we can flexibly obtain the name of the type, which is very helpful for implementing the reflection mechanism.
3. Use macro definitions to obtain information about member variables and member functions
- The information about member variables and member functions of a class cannot be obtained through ordinary C syntax. In order to implement the reflection mechanism, we can obtain this information with the help of macro definitions. We can define two macros, one to get information about member variables and one to get information about member functions:
#define GET_MEMBER_NAME(class_name, member_name) #class_name "::" #member_name #define GET_METHOD_NAME(class_name, method_name) #class_name "::" #method_name "()"
- Then, we can use these macros to get members of the class Names of variables and member functions:
class Foo { public: int a; void bar() {} }; int main() { std::cout << GET_MEMBER_NAME(Foo, a) << std::endl; // 输出 Foo::a std::cout << GET_METHOD_NAME(Foo, bar) << std::endl; // 输出 Foo::bar() return 0; }
Through macro definition, we can obtain the names of member variables and member functions of the class during compilation, thereby realizing dynamic operations on the class.
4. Combine template metaprogramming and macro definition to implement a flexible reflection mechanism
- We can use template metaprogramming and macro definition in combination to implement a complete reflection mechanism. First, we need to define a class to store type information:
class TypeInfo { public: const char* name; // 其他类型相关的信息 };
- Then, we can define a template function to obtain any type of
TypeInfo
Object:
template<typename T> TypeInfo getTypeInfo() { TypeInfo typeInfo; typeInfo.name = getTypeName<T>().c_str(); // 其他类型相关的信息的获取 return typeInfo; }
- Next, we can define a macro to simplify the process of obtaining the
TypeInfo
object:
#define GET_TYPE_INFO(class_name) getTypeInfo<class_name>()
- Finally, we can use this macro to obtain the type information of the class:
class Foo { public: int a; void bar() {} }; int main() { TypeInfo fooTypeInfo = GET_TYPE_INFO(Foo); std::cout << fooTypeInfo.name << std::endl; // 输出 Foo return 0; }
By combining template metaprogramming and macro definition, we can implement a flexible reflection mechanism in C to easily obtain the class type information.
5. Summary
This article introduces how to use template metaprogramming and macro definition to implement the reflection mechanism in C and achieve flexible acquisition of runtime type information. Through this reflection mechanism, we can dynamically operate classes at runtime, improving the flexibility and scalability of the code. Although C does not natively support reflection, we can achieve similar functionality through some tricks and technical means. I hope this article will help readers understand the C reflection mechanism.
The above is the detailed content of C++ Reflection Mechanism Practice: Implementing Flexible Runtime Type Information. For more information, please follow other related articles on the PHP Chinese website!
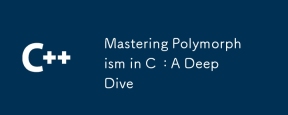
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
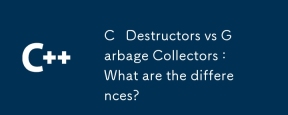
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
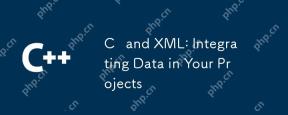
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
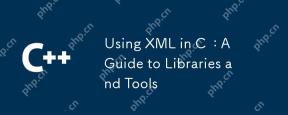
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
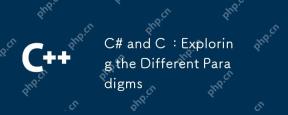
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
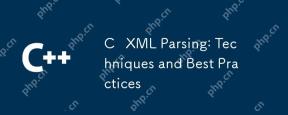
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
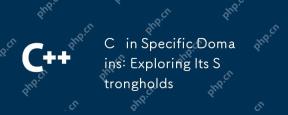
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
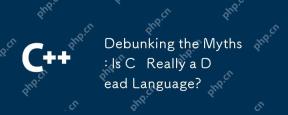
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
