


C# development experience sharing: efficient programming skills and practices
C# Development experience sharing: efficient programming skills and practices
In the field of modern software development, C# has become one of the most popular programming languages. As an object-oriented language, C# can be used to develop various types of applications, including desktop applications, web applications, mobile applications, etc. However, developing an efficient application is not just about using the correct syntax and library functions. It also requires following some programming tips and practices to improve the readability and maintainability of the code. In this article, I will share some efficient tips and practices for C# programming, hoping to be helpful to everyone.
- Use appropriate data types
In C#, there are many built-in data types that can be used, such as integers, floating point types, strings, etc. When selecting data types, we should choose the most appropriate type according to specific needs to improve program performance and memory utilization. For example, if a variable only needs to store integers, then the integer type should be selected instead of the floating point type; if a string is a constant, an immutable string type (such as string) should be used instead of a mutable string type. (like StringBuilder). - Using generic collections
In C#, generic collections can provide better type safety and performance. Compared with non-generic collections, generic collections allow us to specify the type of elements in the collection at compile time, thus avoiding type conversion at run time. Generic collections also improve program performance by reducing boxing and unboxing operations. - Use global variables sparingly
Although global variables are convenient, excessive use will lead to a decrease in the readability and maintainability of the program. In actual development, we should try to avoid using global variables and instead limit the scope of variables to within functions or classes. If you really need to share data between multiple functions, you can consider using attributes or parameter passing. - Use LINQ as much as possible
LINQ (Language Integrated Query) is a powerful function in C#. It provides a unified way to query and operate various data sources, including object collections, databases and XML Documentation etc. Using LINQ can greatly simplify code and improve development efficiency. At the same time, using LINQ can also take advantage of its powerful query capabilities to improve program performance. - Exception handling
Exception handling is an important part of writing robust programs. In C#, we can use try-catch statements to catch and handle exceptions. When handling exceptions, we should try to catch exceptions as precisely as possible, rather than simply using a general catch block to catch all types of exceptions. In addition, we should also pay attention to releasing resources through finally blocks at appropriate times to ensure the normal operation of the program. - Write clear comments and documentation
Good comments and documentation can improve the readability and maintainability of your code. When writing code, we should focus on writing clear and concise comments to help other developers understand the logic and design ideas of the code. In addition, we can also use some tools to automatically generate code documentation to further improve the readability of the code. - Testing and debugging
During the development process, we should always maintain the awareness of testing and debugging. Before writing code, we should define test cases to ensure the correctness and stability of the code. When debugging code, we can use debugger tools to step by step trace the execution process of the code and observe the value and status changes of variables. At the same time, we can also use logging tools to collect and analyze program running information to better locate and solve problems.
Summary
The above are some of my experience sharing in C# development. These skills and practices can help developers write efficient and robust programs. However, different projects and scenarios may require different techniques and methods, so we should choose and apply these techniques according to the specific situation. I hope these experiences will be helpful to everyone, and everyone is welcome to share their own experiences and ideas. I wish everyone will go further and further on the road of C# development!
The above is the detailed content of C# development experience sharing: efficient programming skills and practices. For more information, please follow other related articles on the PHP Chinese website!
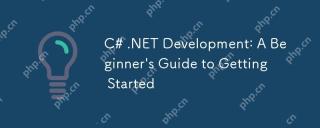
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
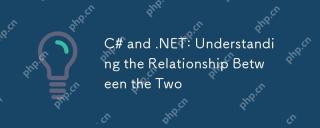
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
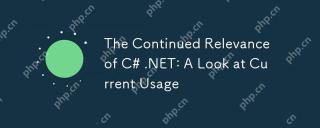
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
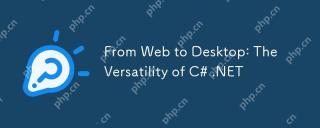
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.
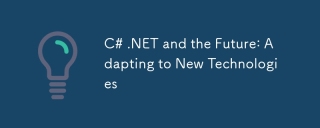
C# and .NET adapt to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET5 introduce record type and performance optimization. 2) .NETCore enhances cloud native and containerized support. 3) ASP.NETCore integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
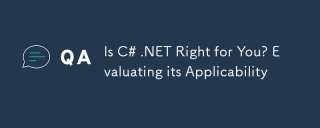
C#.NETissuitableforenterprise-levelapplicationswithintheMicrosoftecosystemduetoitsstrongtyping,richlibraries,androbustperformance.However,itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical,wherelanguageslikeRustorGomightbepreferable.
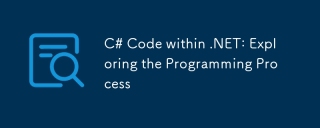
The programming process of C# in .NET includes the following steps: 1) writing C# code, 2) compiling into an intermediate language (IL), and 3) executing by the .NET runtime (CLR). The advantages of C# in .NET are its modern syntax, powerful type system and tight integration with the .NET framework, suitable for various development scenarios from desktop applications to web services.
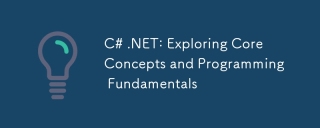
C# is a modern, object-oriented programming language developed by Microsoft and as part of the .NET framework. 1.C# supports object-oriented programming (OOP), including encapsulation, inheritance and polymorphism. 2. Asynchronous programming in C# is implemented through async and await keywords to improve application responsiveness. 3. Use LINQ to process data collections concisely. 4. Common errors include null reference exceptions and index out-of-range exceptions. Debugging skills include using a debugger and exception handling. 5. Performance optimization includes using StringBuilder and avoiding unnecessary packing and unboxing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
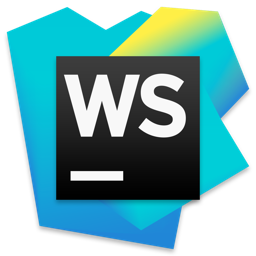
WebStorm Mac version
Useful JavaScript development tools
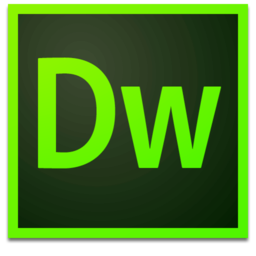
Dreamweaver Mac version
Visual web development tools
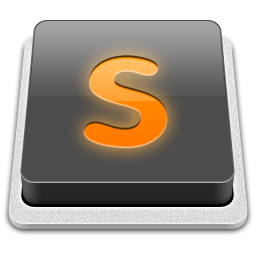
SublimeText3 Mac version
God-level code editing software (SublimeText3)