Development suggestions: How to handle exceptions in ThinkPHP applications
ThinkPHP is a popular PHP framework that provides a development model that is easy to understand and use, allowing developers to build web applications faster and more efficiently. However, even when best practices are used, application errors and exceptions cannot be avoided. Therefore, in this article, we will explore how to handle exceptions in ThinkPHP applications.
- The difference between exceptions and errors
Before handling exceptions, we need to understand the difference between exceptions and errors. In PHP, errors usually occur when something goes wrong in the code. These problems may be syntax errors, type errors, calling undefined functions, etc. Often, errors cause the application to crash or stop working.
Exceptions, on the other hand, are problems that are anticipated when writing code, usually due to external factors such as inability to access the database, network problems, etc. Exceptions typically do not cause the application to crash, but are instead passed through the exception handling mechanism and necessary actions are taken to correct the problem.
- Use try-catch block for exception handling
In ThinkPHP, we can use try-catch block to handle exceptions. Typically, code that may throw exceptions is placed in a try block and one or more catch blocks are defined to catch and handle exceptions. The following is the basic syntax for handling exceptions using try-catch blocks:
try { // 可能发生异常的代码块 } catch (Exception $e) { // 处理异常的代码块 }
In the above code, we use try blocks to wrap the code that may throw exceptions. If an exception is thrown in the try block, control is transferred to the catch block and the exception object is passed to the code in the catch block.
Here is a more concrete example that demonstrates how to handle exceptions using try-catch blocks in ThinkPHP:
try { // 查询数据库 $result = Db::table('user')->where('id', 1)->find(); } catch (Exception $e) { // 处理异常 Log::error('查询数据库错误:' . $e->getMessage()); $result = array(); }
In the above code, we are trying to retrieve from the database the file with id = 1 user information. If any exception occurs during this process, we catch it using catch block, log it and set the result to an empty array.
- Use error handlers to handle errors
When an application encounters an error, a common practice is to output the error to the screen or log it to a log file. In ThinkPHP, we can use error handlers to perform these tasks.
The error handler is a special class that is automatically called when the application encounters an error. ThinkPHP already has an error handler built in, defined in the public/index.php file in the root directory of the application. When an error occurs, the error handler will log the error and print a friendly error message.
The following is the basic syntax of the error handler:
use thinkexceptionHandle; class ExceptionHandler extends Handle { public function render(Exception $e) { // 处理错误 return parent::render($e); } }
In the above code, we extend ThinkPHP's built-in Handle class and override the render method to handle errors. In our implementation, we log errors and call the parent class's render method to output a friendly error message.
- Custom exception handler
We can create our own exception handler to override ThinkPHP's built-in Handle class and implement our own error handling logic. The following is the basic syntax of a custom exception handler:
use thinkexceptionHandle; class ExceptionHandler extends Handle { public function render(Exception $e) { // 处理异常 if ($e instanceof MyException) { // 处理MyException异常 } else { // 调用父类处理其他异常 return parent::render($e); } } }
In the above code, we extend the Handle class and override the render method to handle exceptions. We also define a custom exception class MyException and use an if statement to check whether the current exception is a custom exception. If it is, we will execute our custom logic. Otherwise, we will call the render method of the parent class to handle other exceptions.
Conclusion
In this article, we explored how to handle exceptions in ThinkPHP applications. We learned the difference between exceptions and errors and learned how to use try-catch blocks and error handlers to handle exceptions and errors. Finally, we covered how to create custom exception handlers to implement our own handling logic. With proper exception handling, we can make our applications more robust and reliable.
The above is the detailed content of Development suggestions: How to handle exceptions in ThinkPHP applications. For more information, please follow other related articles on the PHP Chinese website!
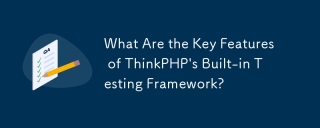
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
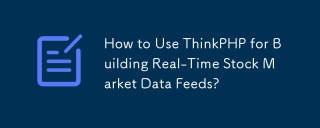
Article discusses using ThinkPHP for real-time stock market data feeds, focusing on setup, data accuracy, optimization, and security measures.

The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
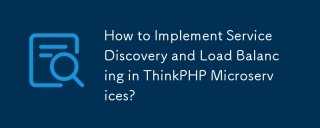
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
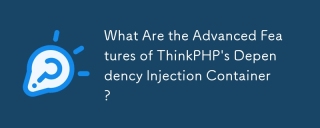
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
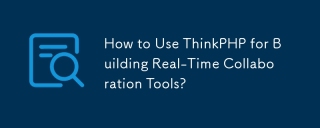
The article discusses using ThinkPHP to build real-time collaboration tools, focusing on setup, WebSocket integration, and security best practices.
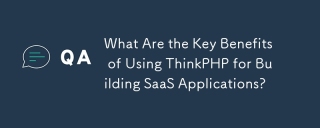
ThinkPHP benefits SaaS apps with its lightweight design, MVC architecture, and extensibility. It enhances scalability, speeds development, and improves security through various features.
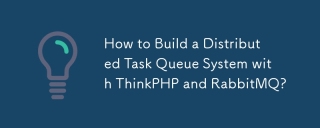
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
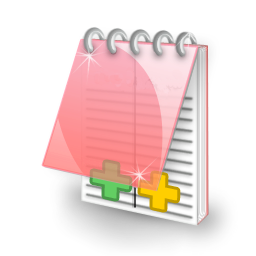
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment
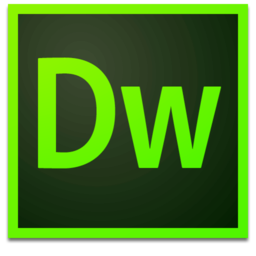
Dreamweaver Mac version
Visual web development tools