How to Use ThinkPHP for Building Real-Time Stock Market Data Feeds?
To use ThinkPHP for building real-time stock market data feeds, you should follow a structured approach that leverages ThinkPHP's MVC architecture and its support for real-time data processing. Here’s a step-by-step guide:
- Set Up the Environment: Install ThinkPHP and necessary dependencies. Make sure your development environment is ready with PHP, a web server like Apache or Nginx, and a database system like MySQL.
-
Design the Model: Create models to represent stock data. In ThinkPHP, models are used to interact with the database. Define fields that will hold real-time stock prices, volume, and other relevant data.
namespace app\model; use think\Model; class Stock extends Model { protected $table = 'stocks'; protected $autoWriteTimestamp = true; }
-
Implement Real-Time Data Fetching: Use WebSocket or server-sent events (SSE) to receive real-time stock updates. For WebSocket, you can integrate a library like Ratchet or Swoole to enable real-time communication between the server and client.
use Ratchet\MessageComponentInterface; use Ratchet\ConnectionInterface; class StockFeed implements MessageComponentInterface { public function onOpen(ConnectionInterface $conn) { // New connection handling } public function onMessage(ConnectionInterface $conn, $msg) { // Process incoming message } public function onClose(ConnectionInterface $conn) { // Connection closed } public function onError(ConnectionInterface $conn, \Exception $e) { // Error handling } }
-
Update and Store Data: Create a controller that processes incoming data and updates the database. Use ThinkPHP's model to save or update stock data.
namespace app\controller; use app\model\Stock; class StockController { public function updateStock($data) { $stock = new Stock; $stock->save($data); } }
- Front-End Integration: Design a front-end that displays the real-time data. Use JavaScript frameworks like React or Vue.js to update the UI based on the data received via WebSocket or SSE.
- Testing and Deployment: Test the system for latency and accuracy, then deploy on a server capable of handling real-time data streams.
What are the best practices for ensuring data accuracy in ThinkPHP real-time stock feeds?
Ensuring data accuracy in real-time stock feeds using ThinkPHP involves several best practices:
-
Data Validation: Before storing or processing any incoming data, validate it using ThinkPHP's validation rules. This helps to ensure that only correct data formats are processed.
use think\Validate; $validate = new Validate([ 'symbol' => 'require|max:10', 'price' => 'require|number', 'volume' => 'require|number' ]); if (!$validate->check($data)) { // Handle validation failure }
- Data Synchronization: Implement mechanisms to ensure that the database is synchronized with the real-time data source. Use timestamp fields and periodic checks to validate data consistency.
- Error Handling and Logging: Set up comprehensive error handling and logging to track any issues with data feeds. ThinkPHP offers built-in logging which can be extended for custom needs.
- Redundancy and Failover: Have redundant systems in place to ensure data accuracy in case of failures. Use backup servers and databases to maintain data integrity.
- Continuous Monitoring: Use monitoring tools to constantly check the accuracy of the data being fed into the system. Set up alerts for any anomalies.
How can ThinkPHP be optimized for handling high-frequency stock market data updates?
Optimizing ThinkPHP for handling high-frequency stock market data updates involves several key strategies:
-
Use of Swoole: Integrate Swoole with ThinkPHP to handle high-frequency data updates. Swoole offers asynchronous, concurrent processing which is vital for real-time applications.
use Swoole\Http\Server; use Swoole\Http\Request; use Swoole\Http\Response; $server = new Server("0.0.0.0", 9501); $server->on('Request', function (Request $request, Response $response) { // Handle request and response }); $server->start();
-
Caching: Implement caching mechanisms like Redis to reduce database load and improve data retrieval speeds. ThinkPHP supports caching out of the box.
use think\Cache; Cache::store('redis')->set('stock_data', $data, 3600); $stockData = Cache::store('redis')->get('stock_data');
- Database Optimization: Use indexing, partitioning, and optimized queries to ensure the database can handle high-frequency updates efficiently.
- Asynchronous Processing: Use background jobs or queues to offload processing that isn't immediately required, allowing the main system to handle data feeds more efficiently.
- Performance Tuning: Monitor and tune server and application performance. Optimize PHP settings, web server configurations, and use profiling tools to identify bottlenecks.
What security measures should be implemented when using ThinkPHP for real-time stock data feeds?
When using ThinkPHP for real-time stock data feeds, several security measures should be implemented to protect both the data and the system:
- Secure Data Transmission: Use SSL/TLS to encrypt data transmitted over WebSocket or other communication protocols. Ensure all data exchanges are secure.
-
Authentication and Authorization: Implement strong authentication mechanisms for users accessing the system. Use OAuth or JWT to securely manage sessions.
use think\facade\Jwt; $token = Jwt::encode(['uid' => 1], 'your_secret_key', 'HS256'); // Verify token $decoded = Jwt::decode($token, 'your_secret_key', ['HS256']);
- Input Sanitization: Sanitize and validate all incoming data to prevent SQL injection and other forms of attacks. ThinkPHP provides built-in sanitization methods.
- Rate Limiting: Implement rate limiting to prevent DoS attacks by restricting the number of requests from a single IP or user within a time frame.
- Data Encryption: Encrypt sensitive data stored in the database or in transit. Use encryption libraries provided by ThinkPHP or external ones like OpenSSL.
- Audit Logging: Keep detailed logs of all access and modifications to data. This helps in tracking and investigating any security incidents.
- Regular Security Audits: Conduct regular security audits and penetration testing to identify and fix vulnerabilities. Update ThinkPHP and its dependencies to the latest secure versions.
By implementing these security measures, you can significantly enhance the security of your real-time stock data feeds in ThinkPHP.
The above is the detailed content of How to Use ThinkPHP for Building Real-Time Stock Market Data Feeds?. For more information, please follow other related articles on the PHP Chinese website!
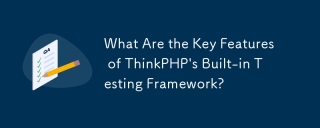
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
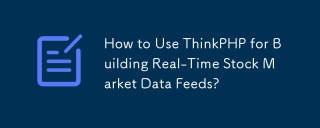
Article discusses using ThinkPHP for real-time stock market data feeds, focusing on setup, data accuracy, optimization, and security measures.

The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
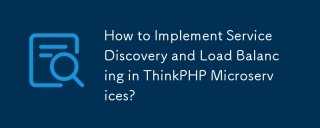
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
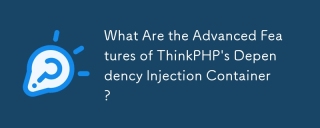
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
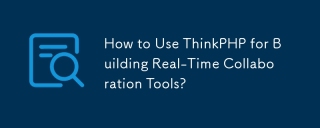
The article discusses using ThinkPHP to build real-time collaboration tools, focusing on setup, WebSocket integration, and security best practices.
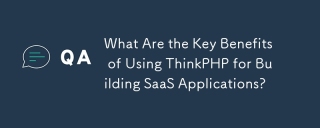
ThinkPHP benefits SaaS apps with its lightweight design, MVC architecture, and extensibility. It enhances scalability, speeds development, and improves security through various features.
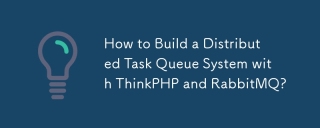
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
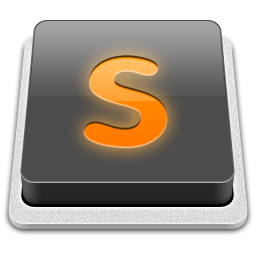
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
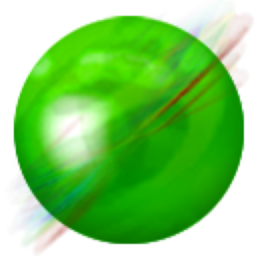
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment