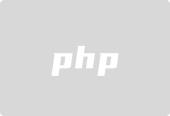
Vue.js is a very powerful JavaScript framework that is widely used to build user interfaces. In actual development, we often encounter various errors and exceptions, so correct error capture and exception handling are very important. This article will introduce you to some best practices for error catching and exception handling in Vue development, and provide some suggestions to help you improve the stability and reliability of your application.
- Use try-catch statement to catch errors
In Vue.js, you can use JavaScript's try-catch statement to catch and handle exceptions. When you suspect that a piece of code may throw an error, you can wrap the code with try and catch the error with catch. The following is a simple example:
try {
// 可能会抛出错误的代码
// ...
} catch (error) {
// 处理错误的代码
// ...
}
- Use Vue's errorCaptured hook to capture global errors
Vue provides an errorCaptured hook that can capture any error information in subcomponents. Adding an errorCaptured hook to the parent component can capture errors thrown by child components and handle them accordingly. You can catch errors globally in the parent component to prevent errors from affecting the entire application.
errorCaptured (err, vm, info) {
// 错误捕获和处理
// ...
}
- Using Vue's global error handler
Vue provides a global error handler to catch uncaught errors in the application. You can use Vue.config.errorHandler to register a global error handler to catch uncaught errors and handle them accordingly.
Vue.config.errorHandler = function (err, vm, info) {
// 全局错误捕获和处理
// ...
}
- Use axios interceptor to handle request errors
When using Vue to make network requests, the axios library is usually used to send HTTP requests. You can leverage axios' interceptors to catch and handle request errors. For example, you can use axios's response interceptor to handle error information in HTTP responses, or use the request interceptor to handle error information before the request is sent.
// 添加响应拦截器
axios.interceptors.response.use(function (response) {
// 正常响应处理
return response;
}, function (error) {
// 错误响应处理
// ...
});
- Write reliable code and avoid unnecessary errors
Last but not least, try to write reliable code and avoid unnecessary errors. This includes sound input validation, error handling, and boundary condition checking, as well as following best programming practices and conventions.
Summary
In Vue development, correct error catching and exception handling are crucial to ensure the stability and reliability of the application. By using try-catch statements, Vue's errorCaptured hooks, global error handlers, and axios interceptors, you can effectively capture and handle various errors and exceptions. Additionally, writing reliable code is very important and can help you avoid many potential errors. I hope the suggestions provided in this article can help you better develop Vue and improve the quality and stability of your application.
The above is the detailed content of Vue development advice: How to do error catching and exception handling. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn