


How to implement large-scale data processing at the bottom of PHP requires specific code examples
In modern software development, data processing is a very important and complex task. For processing large-scale data, performance and efficiency factors especially need to be taken into consideration. In PHP development, we can realize the underlying operations of large-scale data processing by optimizing algorithms and adopting appropriate data structures. This article will introduce some common technologies and specific code examples to help readers implement large-scale data processing at the bottom of PHP.
1. Use efficient data structures
When processing large-scale data, it is very important to choose an appropriate data structure. Commonly used data structures include arrays, linked lists, queues, stacks, and hash tables. In PHP, arrays are the most commonly used data structure, but they are not always the most efficient. In some cases, using the SplFixedArray class can improve performance. The SplFixedArray class is a class provided by PHP specifically for processing large-scale data. It uses a fixed-length array internally to store data, which can reduce memory usage and improve access speed. The following is an example of using the SplFixedArray class:
<?php $array = new SplFixedArray(1000000); // 创建一个包含1000000个元素的固定长度数组 for ($i = 0; $i < $array->getSize(); $i++) { $array[$i] = $i; // 给每个元素赋值 } ?>
2. Use an appropriate amount of loops and conditional statements
When processing large-scale data, the efficiency of loops and conditional statements plays a crucial role in performance important role. Try to avoid using a large number of loops and nested conditional statements, and consider using an appropriate amount of loops and conditional statements to optimize the code. Here are some optimization examples:
- Use foreach instead of for loop to traverse the array:
<?php $array = [1, 2, 3, 4, 5]; foreach ($array as $value) { // 处理数据 } ?>
- Use isset to detect whether an array element exists:
<?php if (isset($array['key'])) { // 处理元素 } ?>
- Use switch statements to replace multiple if-else statements:
<?php switch ($var) { case 'value1': // 处理代码 break; case 'value2': // 处理代码 break; default: // 处理代码 } ?>
3. Parallel processing of data
Parallel processing of data is a way to improve large-scale An important means of data processing efficiency. In PHP, you can use multi-threads or multi-processes to achieve parallel processing of data. The following is an example of using multiple processes to process data in parallel:
<?php $processes = []; // 进程数组 $cpuCores = 4; // CPU核心数 // 创建多个子进程 for ($i = 0; $i < $cpuCores; $i++) { $process = new SwooleProcess(function (SwooleProcess $worker) { // 处理数据 }); $pid = $process->start(); $processes[$pid] = $process; } // 等待子进程执行完毕 foreach ($processes as $process) { SwooleProcess::wait(); } ?>
4. Use caching to accelerate data access
For large-scale data processing, caching is an important means to improve performance. By using caching technology, the number of data reads and writes can be reduced, reducing the pressure on the database and file system. Commonly used caching technologies in PHP include Redis and Memcached. The following is an example of using Redis cache:
<?php $redis = new Redis(); $redis->connect('127.0.0.1', 6379); // 先从缓存中获取数据 $data = $redis->get('data'); if (!$data) { // 缓存中没有数据,从数据库读取数据 $data = getDataFromDatabase(); // 将数据存入缓存 $redis->set('data', $data, 60); // 60秒过期 } // 处理数据 ?>
Summary:
This article introduces some technologies and specific code examples for implementing large-scale data processing at the bottom of PHP. By optimizing the selection of data structures, using appropriate loops and conditional statements, processing data in parallel, and using cache to accelerate data access, the performance and efficiency of large-scale data processing can be improved. It is hoped that readers can use these technologies to process large-scale data in actual development through the introduction of this article.
The above is the detailed content of How to implement large-scale data processing at the bottom of PHP. For more information, please follow other related articles on the PHP Chinese website!
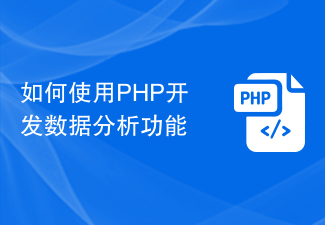
如何使用PHP开发数据分析功能引言:现代数据处理和分析已经成为了企业和组织成功的关键所在。随着海量数据的产生和存储,采集、处理和分析数据变得越来越重要。PHP作为一种广泛应用于Web开发的语言,也提供了强大的数据处理和分析功能。本文将介绍如何使用PHP开发数据分析功能,并提供代码示例。一、数据采集与存储在进行数据分析之前,首先需要采集和存储数据。PHP可以使

大规模数据处理中的Python并发编程问题详解在当今数据爆炸的时代,大规模数据处理成为了许多领域的重要任务。对于处理海量数据,提高处理效率是至关重要的。而在Python中,通过并发编程可以有效提高程序的执行速度,从而更高效地处理大规模数据。然而,并发编程也存在一些问题和挑战,特别是在大规模数据处理中。下面我们将分析并解决一些常见的Python并发编程问题,并
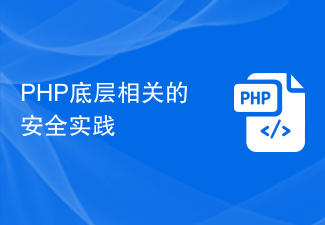
PHP底层相关的安全实践,需要具体代码示例随着Web应用程序的快速发展,网络安全威胁也日益增加。作为广泛使用的后端编程语言之一,PHP应用程序也面临着各种潜在的安全风险。为了能够保护PHP应用程序免受恶意攻击,开发人员需要了解一些基本的底层安全实践,并在代码中采取相应的防护措施。下面将介绍几个与PHP底层相关的安全实践,并提供具体的代码示例。输入验
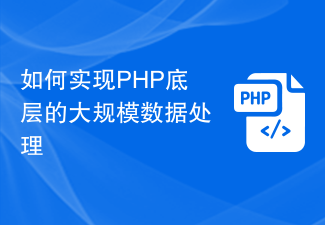
如何实现PHP底层的大规模数据处理,需要具体代码示例在现代的软件开发中,数据处理是一个非常重要而复杂的任务。对于大规模数据的处理,尤其需要考虑到性能和效率的因素。在PHP开发中,我们可以通过优化算法和采用适当的数据结构来实现大规模数据处理的底层操作。本文将介绍一些常见的技术和具体的代码示例,帮助读者实现PHP底层的大规模数据处理。一、使用高效的数据结构在处理
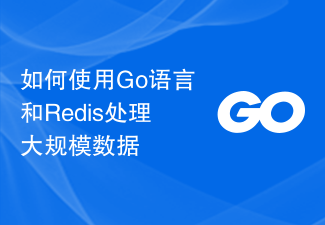
如何使用Go语言和Redis处理大规模数据在当今互联网时代,处理大规模数据成为了许多应用程序的核心需求。Go语言以其简洁、高效和并发性而著称,而Redis是一款高性能的键值存储系统。将它们结合使用,可以优化数据处理速度和资源利用率。本文将介绍如何使用Go语言和Redis处理大规模数据,并提供具体的代码示例。一、连接Redis首先,我们
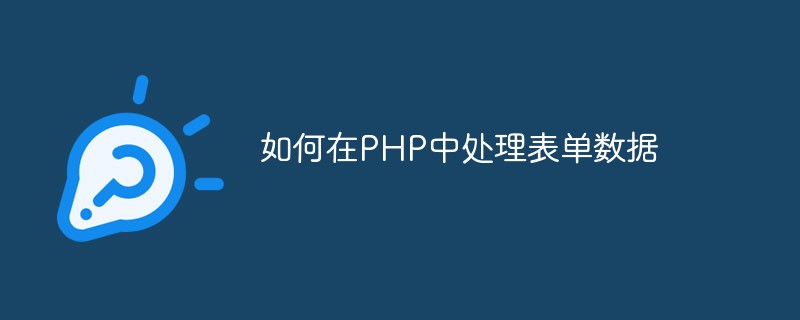
随着互联网的不断发展,表单已经成为了网页开发中不可或缺的一部分。表单可以让用户输入需要提交的数据,进而实现与服务器之间的交互。在PHP中,处理表单数据十分方便。本文将介绍如何在PHP中处理表单数据。1.获取表单数据当用户提交表单时,PHP可以通过$_POST或$_GET变量获取表单提交的数据。其中,$_POST变量用于获取提交方式为POST的表单数
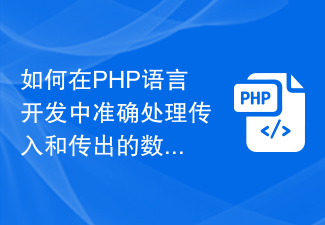
在PHP语言中,数据的传入和传出是非常常见且重要的操作。而对于开发者来说,如何准确地处理这些数据,则是一个十分重要的课题。因此,为了帮助大家更好地掌握这个技巧,本文将简要介绍如何在PHP语言开发中准确处理传入和传出的数据。一、传入数据的准确处理过滤不安全的字符通过引用用户输入、上传或读取的数据,我们可能会引起各种问题,包括SQL注入和XSS攻击。为了防止这
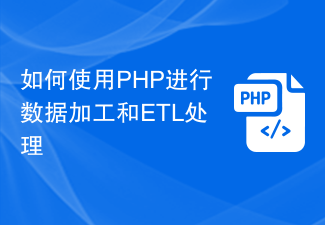
如何使用PHP进行数据加工和ETL处理概述:数据加工(DataWrangling)和ETL(Extract,Transform,Load)是数据处理中常见的任务。PHP作为一种流行的服务器端脚本语言,具有强大的数据处理能力。本文将介绍如何使用PHP进行数据加工和ETL处理,并提供代码示例。一、数据加工(DataWrangling):数据加工是指对原始


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
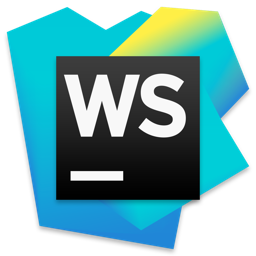
WebStorm Mac version
Useful JavaScript development tools
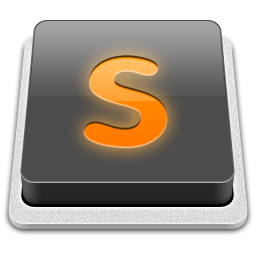
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
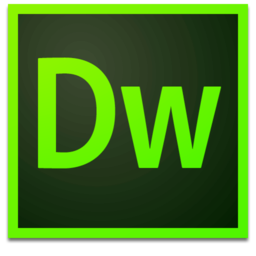
Dreamweaver Mac version
Visual web development tools
