


In-depth exploration of the underlying technology of Python: how to implement the gradient descent algorithm, specific code examples are required
Introduction:
The gradient descent algorithm is a commonly used optimization algorithm. It is widely used in the fields of machine learning and deep learning. This article will delve into the underlying technology of Python, introduce the principle and implementation process of the gradient descent algorithm in detail, and provide specific code examples.
1. Introduction to Gradient Descent Algorithm
The gradient descent algorithm is an optimization algorithm. Its core idea is to gradually approach the minimum value of the loss function by iteratively updating parameters. Specifically, the steps of the gradient descent algorithm are as follows:
- Randomly initialize parameters.
- Calculate the gradient of the loss function to the parameters.
- Update parameters based on the direction of the gradient and the learning rate.
- Repeat steps 2 and 3 until the condition for the algorithm to stop is reached.
2. Implementation process of gradient descent algorithm
In Python, we can implement the gradient descent algorithm through the following steps.
- Preparing data
First, we need to prepare the data set, including input features and target values. Assuming there are m samples and n features, we can represent the input features as an m×n matrix X, and the target value as a vector y of length m. - Initialization parameters
We need to initialize the parameters of the model, including weight w and bias b. In general, the weight w can be set to a vector of dimension n, and the bias b can be initialized to a scalar. -
Calculate the loss function
We need to define a loss function to evaluate the performance of the model. In the gradient descent algorithm, the commonly used loss function is the squared error loss function, which is defined as follows:def loss_function(X, y, w, b): m = len(y) y_pred = np.dot(X, w) + b loss = (1/(2*m))*np.sum((y_pred - y)**2) return loss
-
Calculating the gradient
Next, we need to calculate the effect of the loss function on the weight w and bias Set the gradient of b. The gradient represents the fastest decreasing direction of the objective function at a certain point. For the squared error loss function, the gradient calculation formula is as follows:def gradient(X, y, w, b): m = len(y) y_pred = np.dot(X, w) + b dw = (1/m)*np.dot(X.T, (y_pred - y)) db = (1/m)*np.sum(y_pred - y) return dw, db
-
Update parameters
According to the direction of the gradient and the learning rate alpha, we can update the parameters so that they move towards the loss function Minimize directional movement.def update_parameters(w, b, dw, db, learning_rate): w = w - learning_rate * dw b = b - learning_rate * db return w, b
- Iteratively update parameters
By repeating steps 4 and 5 until the condition for the algorithm to stop is reached. The condition for the algorithm to stop can be that the maximum number of iterations is reached, or the change in the loss function is less than a certain threshold. -
Full code example
The following is a complete code example that implements the gradient descent algorithm.import numpy as np def gradient_descent(X, y, learning_rate, num_iterations): m, n = X.shape w = np.random.randn(n) b = 0 for i in range(num_iterations): loss = loss_function(X, y, w, b) dw, db = gradient(X, y, w, b) w, b = update_parameters(w, b, dw, db, learning_rate) if i % 100 == 0: print(f"Iteration {i}: loss = {loss}") return w, b # 测试代码 X = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) # 输入特征矩阵 y = np.array([4, 7, 10]) # 目标值 learning_rate = 0.01 # 学习率 num_iterations = 1000 # 迭代次数 w, b = gradient_descent(X, y, learning_rate, num_iterations) print(f"Optimized parameters: w = {w}, b = {b}")
Conclusion:
This article deeply explores the underlying technology of Python and introduces the principle and implementation process of the gradient descent algorithm in detail. Through specific code examples, readers can more intuitively understand the implementation details of the gradient descent algorithm. Gradient descent algorithm is an indispensable optimization algorithm in the fields of machine learning and deep learning, and is of great significance for solving practical problems. I hope this article can be helpful to readers and trigger more thinking and discussion about Python's underlying technology.
The above is the detailed content of In-depth exploration of Python's underlying technology: how to implement the gradient descent algorithm. For more information, please follow other related articles on the PHP Chinese website!
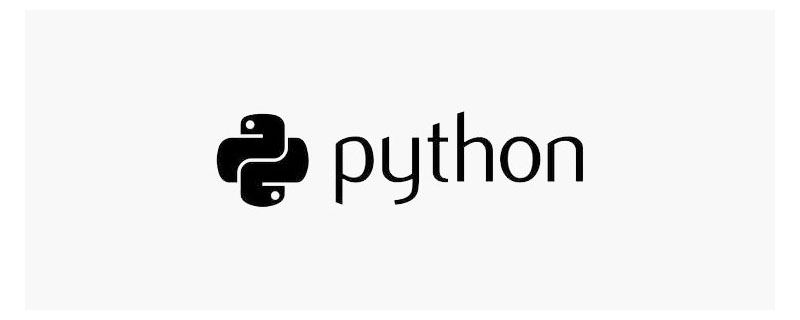
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
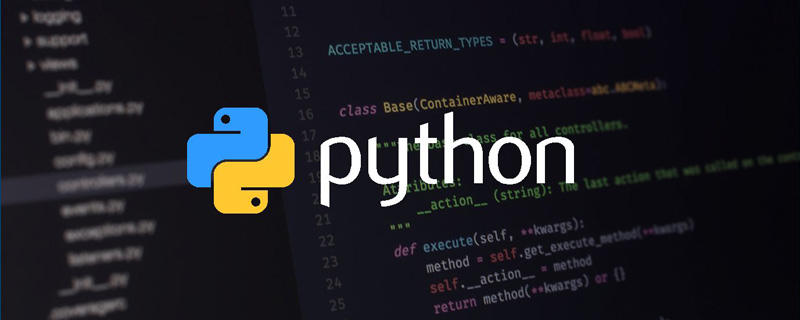
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
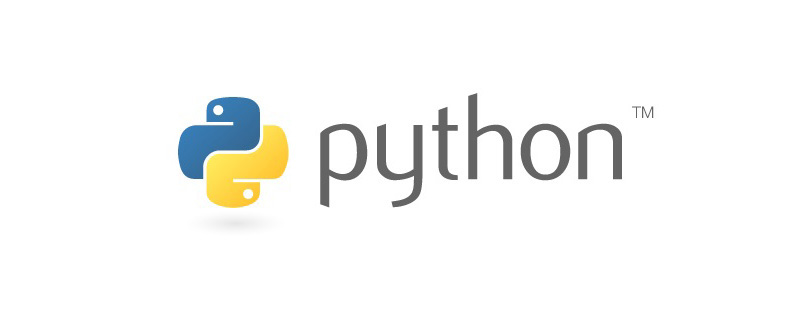
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
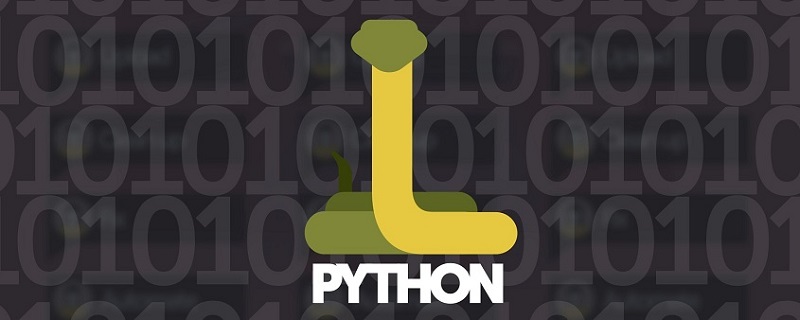
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
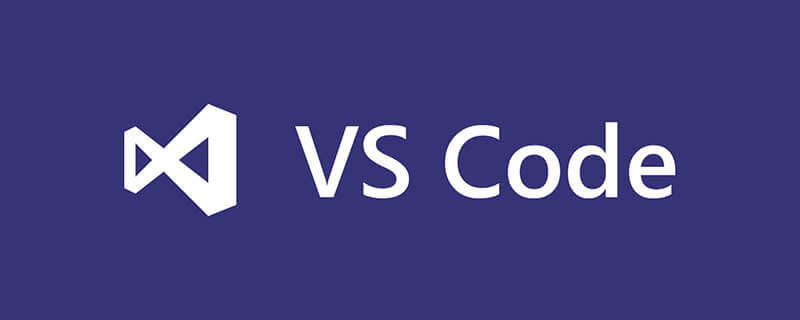
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
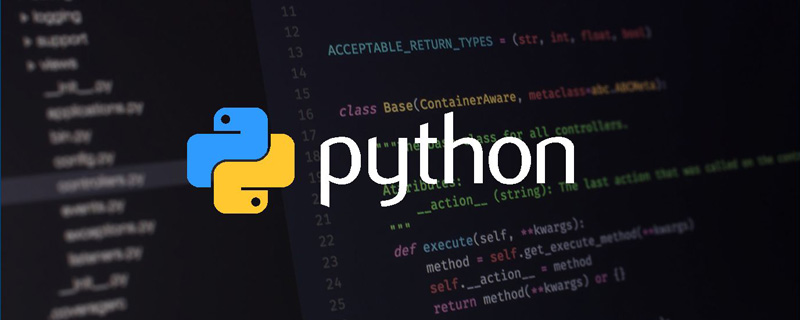
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
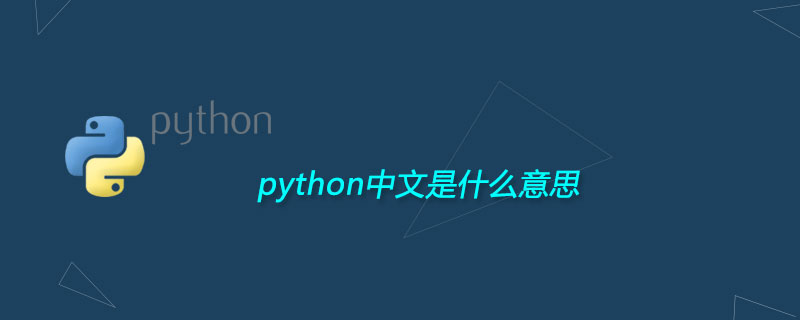
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
