Use JavaScript functions to dynamically update data visualizations
Use JavaScript functions to implement dynamic updates of data visualization
Data visualization is a very important part in the era of big data. It can display data in an intuitive way and help People understand and analyze data better. JavaScript, as a client-side scripting language, can achieve dynamic updates of data visualization through functions. This article will introduce how to use JavaScript functions to achieve this functionality and provide specific code examples.
1. Basics of Data Visualization
Before we start writing code, we first need to understand some basic knowledge. Data visualization usually displays data by drawing charts. In JavaScript, we can use some commonly used libraries to draw charts, such as D3.js, ECharts, etc. These libraries provide rich APIs and functions that can help us quickly draw various types of charts.
2. Dynamic update of data
In practical applications, data often changes dynamically. In order to achieve dynamic updating of data, we need to write some functions to update the data in the chart and redraw the chart. The following is a simple sample code:
// 定义数据 var data = [10, 20, 30, 40, 50]; // 定义画布的宽度和高度 var width = 400; var height = 300; // 创建SVG画布 var svg = d3.select("body") .append("svg") .attr("width", width) .attr("height", height); // 创建柱状图 svg.selectAll("rect") .data(data) .enter() .append("rect") .attr("x", function(d, i) {return i * 50;}) .attr("y", function(d, i) {return height - d;}) .attr("width", 40) .attr("height", function(d, i) {return d;}) .attr("fill", "blue"); // 定义更新函数 function updateData() { // 生成随机数据 var newData = []; for (var i = 0; i < data.length; i++) { newData.push(Math.random() * 50); } // 更新图表 svg.selectAll("rect") .data(newData) .transition() .duration(1000) .attr("y", function(d, i) {return height - d;}) .attr("height", function(d, i) {return d;}); } // 每隔一段时间调用更新函数 setInterval(updateData, 2000);
The above code first defines an array containing 5 data, then creates an SVG canvas, and uses the D3.js library to draw a histogram. Then a function named updateData
is defined, which generates random data and updates the chart. Finally, use the setInterval
function to call the updateData
function every 2 seconds to achieve dynamic updating of data.
3. Conclusion
This article introduces how to use JavaScript functions to achieve dynamic updates of data visualization, and provides a simple code example. Of course, this is just a basic example, and actual applications will be more complicated. I hope readers can use this example to further delve into and explore the world of data visualization.
The above is the detailed content of Use JavaScript functions to dynamically update data visualizations. For more information, please follow other related articles on the PHP Chinese website!
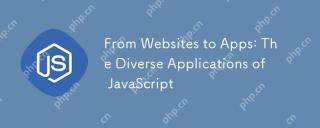
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
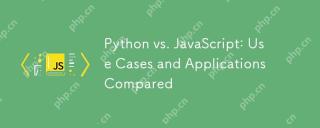
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
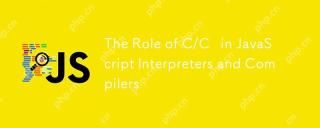
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
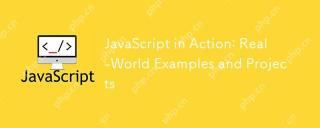
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
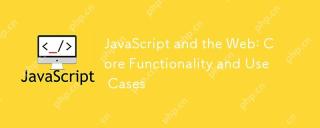
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
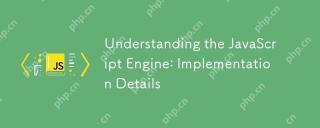
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
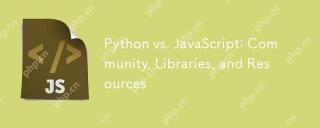
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
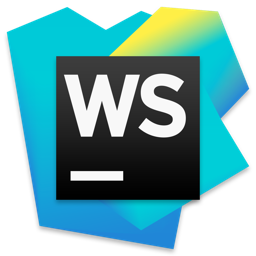
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
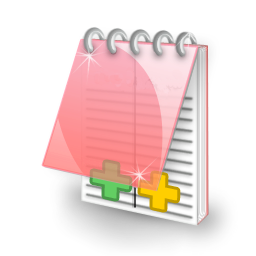
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software