How to use middleware for role management in Laravel
How to use middleware for role management in Laravel
Role management is a very important feature when developing web applications. Through role management, the access rights of different users can be restricted to ensure system security and data confidentiality. In the Laravel framework, role management can be achieved through middleware.
Middleware is a feature of the Laravel framework that can perform some logic before or after the request reaches the route. By using middleware, you can easily restrict users' access based on their roles.
Let’s take a look at the specific steps on how to use middleware for role management.
- Create a middleware
First, we need to create a middleware. Run the following command on the command line to create a middleware named RoleMiddleware:
php artisan make:middleware RoleMiddleware
This command will create a RoleMiddleware.php file in the app/Http/Middleware directory.
In the RoleMiddleware.php file, we need to implement a handle method, which will be executed when the middleware is executed. In this method, we can write our logic to determine whether the user's role has the corresponding permissions.
- Writing middleware logic
In the handle method of the RoleMiddleware.php file, we can write our role management logic. For example, we can use Laravel's Auth facade to get the role of the currently logged in user and compare it with the role we set. If the role matches, we can continue to execute the request, otherwise return an error page or redirect to other pages. The following is a simple sample code:
public function handle($request, Closure $next, ...$roles) { $user = Auth::user(); if (!in_array($user->role, $roles)) { return redirect('/403'); //没有权限 } return $next($request); }
In this example, we get the role of the currently logged in user through the Auth facade and then compare it with the role passed into the middleware. If the user's role is not in the specified role array $roles, we redirect the user to a 403 page and return a page without permissions.
- Register middleware
In the Laravel framework, we need to register the middleware into the middleware group or route before it can be used. In the app/Http/Kernel.php file, we can find the $middlewareGroups attribute or the $routeMiddleware attribute. We can add the middleware we created to these properties in the appropriate places. For example, we can add middleware to the web middleware group so that it applies to all web routes:
protected $middlewareGroups = [ 'web' => [ ... AppHttpMiddlewareRoleMiddleware::class, ], ];
We can also apply middleware directly to a route. For example, we can create a routing group and specify the middleware in the routing group as follows:
Route::middleware('role:admin')->group(function () { //这里的路由只允许角色为admin的用户访问 });
In this example, we apply the RoleMiddleware middleware to this routing group, only those with the role of admin Only users can access these routes.
So far, we have completed the steps of using middleware for role management in Laravel. Through this simple example, you can perform more complex role management according to your actual needs.
Summary
Role management is an important function that can be achieved by using middleware. In the Laravel framework, we can manage roles by creating middleware, writing middleware logic, and registering middleware. Through reasonable use of middleware, we can easily restrict the access rights of different users and improve system security and data confidentiality.
I hope this article can help you use middleware for role management in Laravel. If you have any questions or suggestions, please leave a comment below.
The above is the detailed content of How to use middleware for role management in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Collaborative document editing is an effective tool for distributed teams to optimize their workflows. It improves communication and project progress through real-time collaboration and feedback loops, and common tools include Google Docs, Microsoft Teams, and Notion. Pay attention to challenges such as version control and learning curve when using it.

ThepreviousversionofLaravelissupportedwithbugfixesforsixmonthsandsecurityfixesforoneyearafteranewmajorversion'srelease.Understandingthissupporttimelineiscrucialforplanningupgrades,ensuringprojectstability,andleveragingnewfeaturesandsecurityenhancemen

Laravelcanbeeffectivelyusedforbothfrontendandbackenddevelopment.1)Backend:UtilizeLaravel'sEloquentORMforsimplifieddatabaseinteractions.2)Frontend:LeverageBladetemplatesforcleanHTMLandintegrateVue.jsfordynamicSPAs,ensuringseamlessfrontend-backendinteg

Laravelcanbeusedforfullstackdevelopment.1)BackendmasterywithLaravel'sexpressivesyntaxandfeatureslikeEloquentORMfordatabasemanagement.2)FrontendintegrationusingBladefordynamicHTMLtemplates.3)EnhancingfrontendwithLaravelMixforassetcompilation.4)Fullsta

Answer: The best tools for upgrading Laravel include Laravel's UpgradeGuide, LaravelShift, Rector, Composer, and LaravelPint. 1. Use Laravel's UpgradeGuide as the upgrade roadmap. 2. Use LaravelShift to automate most of the upgrade work, but it requires manual review. 3. Automatically refactor the code through Rector, and you need to understand and possibly customize its rules. 4. Use Composer to manage dependencies and pay attention to possible dependency conflicts. 5. Run LaravelPint to maintain code style consistency, but it does not solve the functional problems.

ToenhanceengagementandcohesionamongdistributedteamsbeyondZoom,implementthesestrategies:1)Organizevirtualcoffeebreaksforinformalchats,2)UseasynchronoustoolslikeSlackfornon-workdiscussions,3)Introducegamificationwithteamgamesorchallenges,and4)Encourage

Laravel10introducesseveralbreakingchanges:1)ItrequiresPHP8.1orhigher,2)TheRouteServiceProvidernowusesabootmethodforloadingroutes,3)ThewithTimestamps()methodonEloquentrelationshipsisdeprecated,and4)TheRequestclassnowpreferstherules()methodforvalidatio

Tomaintainfocusandmotivationinremotework,createastructuredenvironment,managedigitaldistractions,fostermotivationthroughsocialinteractionsandgoalsetting,maintainwork-lifebalance,anduseappropriatetechnology.1)Setupadedicatedworkspaceandsticktoaroutine.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
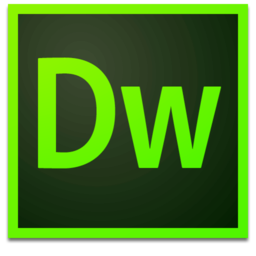
Dreamweaver Mac version
Visual web development tools
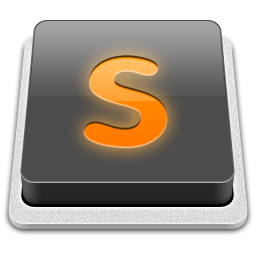
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
